使用Python创建自己的库
创建一个Python库的过程包括几个关键步骤:定义库的功能、编写代码、组织目录结构、编写文档、配置打包文件、发布到PyPI。在这篇文章中,我们将详细介绍每个步骤,指导你如何用Python编写并发布自己的库。
一、定义库的功能
在开始编写库之前,首先需要明确你想要实现什么功能。这个功能可以是一个简单的数学运算库,也可以是一个复杂的机器学习工具。明确功能后,你可以开始设计代码结构和模块划分。
二、编写代码
1、创建项目目录结构
一个良好的项目目录结构可以帮助你更好地组织代码和文档。以下是一个典型的Python库项目目录结构:
my_library/
│
├── my_library/
│ ├── __init__.py
│ ├── module1.py
│ └── module2.py
│
├── tests/
│ ├── __init__.py
│ ├── test_module1.py
│ └── test_module2.py
│
├── setup.py
├── README.md
└── LICENSE
2、编写代码文件
在my_library
文件夹中创建Python模块和包。比如,你可以在module1.py
中实现一些基本功能:
# my_library/module1.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
在module2.py
中实现更多功能:
# my_library/module2.py
def multiply(a, b):
return a * b
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
在__init__.py
中导入模块,使其成为一个包:
# my_library/__init__.py
from .module1 import add, subtract
from .module2 import multiply, divide
三、编写文档
一个好的库不仅需要良好的代码,还需要详细的文档。在README.md
中描述你的库的功能、安装方法和使用示例:
# My Library
My Library is a simple Python library for basic mathematical operations.
## Installation
You can install My Library using pip:
pip install my_library
## Usage
```python
from my_library import add, subtract, multiply, divide
print(add(2, 3)) # Output: 5
print(subtract(5, 2)) # Output: 3
print(multiply(3, 4)) # Output: 12
print(divide(10, 2)) # Output: 5.0
### 四、编写测试
编写单元测试可以确保你的库功能正确。在`tests`文件夹中创建测试文件:
```python
tests/test_module1.py
import unittest
from my_library import add, subtract
class TestModule1(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
def test_subtract(self):
self.assertEqual(subtract(5, 2), 3)
self.assertEqual(subtract(0, 0), 0)
if __name__ == '__main__':
unittest.main()
# tests/test_module2.py
import unittest
from my_library import multiply, divide
class TestModule2(unittest.TestCase):
def test_multiply(self):
self.assertEqual(multiply(3, 4), 12)
self.assertEqual(multiply(-1, 1), -1)
def test_divide(self):
self.assertEqual(divide(10, 2), 5.0)
with self.assertRaises(ValueError):
divide(10, 0)
if __name__ == '__main__':
unittest.main()
五、配置打包文件
1、创建setup.py文件
setup.py
文件是配置你的库如何被打包和发布的核心文件。以下是一个简单的setup.py
示例:
# setup.py
from setuptools import setup, find_packages
setup(
name='my_library',
version='0.1.0',
packages=find_packages(),
description='A simple Python library for basic mathematical operations',
long_description=open('README.md').read(),
long_description_content_type='text/markdown',
author='Your Name',
author_email='your.email@example.com',
url='https://github.com/yourusername/my_library',
classifiers=[
'Programming Language :: Python :: 3',
'License :: OSI Approved :: MIT License',
'Operating System :: OS Independent',
],
python_requires='>=3.6',
)
2、创建LICENSE文件
选择一个开源许可证并在LICENSE
文件中包含其内容。以下是一个MIT许可证示例:
MIT License
Copyright (c) 2023 Your Name
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
...
六、发布到PyPI
1、创建PyPI账户
在发布库之前,你需要在PyPI上创建一个账户。
2、安装必要的工具
在你的开发环境中安装twine
和setuptools
:
pip install twine setuptools
3、构建分发包
运行以下命令来构建你的库:
python setup.py sdist bdist_wheel
4、上传到PyPI
使用twine
将你的库上传到PyPI:
twine upload dist/*
七、维护和更新
发布库后,你可能需要修复bug、添加新功能或改进文档。以下是一些维护和更新库的最佳实践:
1、版本控制
使用版本控制系统(如Git)来管理你的代码和版本。每次发布新版本时,更新setup.py
中的版本号,并在README.md
中记录更改。
2、持续集成
设置持续集成(CI)工具(如Travis CI、GitHub Actions)来自动运行测试和构建你的库。这可以帮助你确保每次提交的代码都是稳定和可用的。
3、用户反馈
积极响应用户的反馈和问题。在GitHub上创建一个issue模板,以便用户可以轻松报告bug或提出功能请求。
总结
创建并发布一个Python库可能看起来很复杂,但通过分解成多个步骤,可以更容易地管理和实现。定义库的功能、编写代码、组织目录结构、编写文档、配置打包文件、发布到PyPI,每一步都有其重要性。通过遵循这些步骤,你可以创建一个高质量的Python库,并与全球的Python开发者共享你的代码。
相关问答FAQs:
如何开始创建自己的Python库?
创建一个Python库的第一步是明确你的库的功能。选择一个你感兴趣的主题或有用的工具,确保它能够解决特定问题。接下来,组织你的代码,确保每个功能模块化,这样方便维护和扩展。最后,准备好项目结构,通常需要包括setup.py
文件、README.md
文档以及必要的源代码文件。
我需要哪些工具来创建Python库?
创建Python库通常需要一些基本的工具。首先,你需要安装Python及其开发环境,可以使用如PyCharm或VSCode等IDE来编写代码。为了管理依赖关系,使用pip
和virtualenv
会非常有帮助。此外,使用Git进行版本控制可以帮助你跟踪更改并与他人协作。
如何发布我的Python库以供他人使用?
在你的库完成后,发布到PyPI(Python Package Index)是一个很好的选择。你需要创建一个PyPI账户,并确保你的setup.py
文件正确配置。使用twine
工具可以方便地上传你的包。在发布之前,建议先在本地或测试环境中进行充分的测试,确保没有错误,并且文档齐全,以便其他用户能够轻松上手。
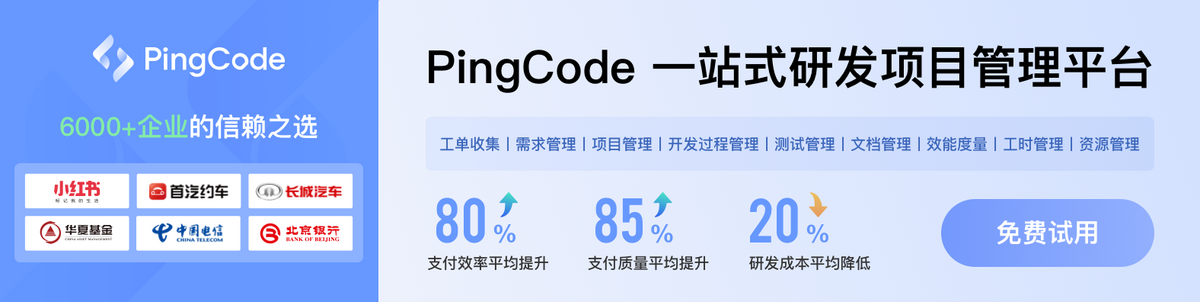