Python下读取二进制文件的方法包括:使用open函数、使用with语句、使用struct模块解析数据、使用numpy库读取大文件。 我们将详细介绍其中的使用open函数和with语句的方法。
在Python中,读取二进制文件的基本方式是使用open
函数,并将模式设置为'rb'
。open
函数返回一个文件对象,我们可以使用这个对象的read
方法读取文件内容。为了确保文件在处理完后能够正确关闭,推荐使用with
语句,这样即使发生异常,文件也会被正确关闭。
一、使用 open
函数读取二进制文件
使用 open
函数读取二进制文件时,我们需要将模式设置为 'rb'
,表示以二进制模式读取文件。以下是一个简单的示例:
def read_binary_file(file_path):
try:
with open(file_path, 'rb') as file:
data = file.read()
return data
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/binary/file'
binary_data = read_binary_file(file_path)
在这个示例中,我们定义了一个函数 read_binary_file
,它接收一个文件路径作为参数,使用 open
函数以二进制模式打开文件,并读取文件内容。读取的数据存储在变量 data
中,并在函数结束时返回。使用 with
语句可以确保文件在读取完成后自动关闭。
二、使用 struct
模块解析二进制文件
对于复杂的二进制文件,我们可能需要解析文件中的数据结构。这时可以使用 struct
模块,它提供了将字节数据解析为Python数据类型的功能。
import struct
def parse_binary_file(file_path):
try:
with open(file_path, 'rb') as file:
header = file.read(8) # 假设文件开头有8字节的头部信息
magic_number, version = struct.unpack('I4s', header)
print(f"Magic Number: {magic_number}, Version: {version.decode('utf-8')}")
# 继续读取和解析文件中的数据...
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/binary/file'
parse_binary_file(file_path)
在这个示例中,我们使用 struct.unpack
方法将文件头部的8字节数据解析为一个整数和一个字符串。'I4s'
表示解析一个4字节的无符号整数和一个4字节的字符串。解析后的数据存储在 magic_number
和 version
变量中。
三、使用 numpy
库读取大文件
如果需要处理大文件,可以使用 numpy
库,它提供了高效的数组操作方法,并且可以直接从二进制文件中读取数据。
import numpy as np
def read_large_binary_file(file_path, dtype, count):
try:
data = np.fromfile(file_path, dtype=dtype, count=count)
return data
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/binary/file'
dtype = np.float32 # 假设文件中存储的是浮点数
count = 100 # 读取前100个数据
binary_data = read_large_binary_file(file_path, dtype, count)
在这个示例中,我们使用 numpy.fromfile
方法从二进制文件中读取数据。dtype
参数指定数据类型,count
参数指定要读取的数据数量。读取的数据存储在 binary_data
数组中。
四、处理二进制文件中的结构化数据
有时候,我们需要从二进制文件中读取结构化数据,例如图像文件、音频文件等。此时可以使用相应的库来处理这些文件。
读取图像文件
对于读取图像文件,可以使用 Pillow
库:
from PIL import Image
def read_image_file(file_path):
try:
with Image.open(file_path) as img:
img.show()
return img
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/image/file'
image = read_image_file(file_path)
在这个示例中,我们使用 Pillow
库的 Image.open
方法打开图像文件,并使用 img.show
方法显示图像。
读取音频文件
对于读取音频文件,可以使用 wave
库:
import wave
def read_audio_file(file_path):
try:
with wave.open(file_path, 'rb') as wf:
params = wf.getparams()
frames = wf.readframes(params.nframes)
return params, frames
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/audio/file'
params, audio_data = read_audio_file(file_path)
在这个示例中,我们使用 wave
库的 wave.open
方法打开音频文件,并读取文件参数和音频数据。
五、处理二进制文件中的文本数据
如果二进制文件中包含文本数据,可以使用 codecs
模块进行解码:
import codecs
def read_text_from_binary_file(file_path, encoding='utf-8'):
try:
with open(file_path, 'rb') as file:
data = file.read()
text = codecs.decode(data, encoding)
return text
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/binary/file'
text_data = read_text_from_binary_file(file_path)
在这个示例中,我们使用 codecs.decode
方法将二进制数据解码为文本数据。
六、处理二进制文件中的压缩数据
如果二进制文件中包含压缩数据,可以使用 zlib
模块进行解压缩:
import zlib
def decompress_binary_file(file_path):
try:
with open(file_path, 'rb') as file:
compressed_data = file.read()
decompressed_data = zlib.decompress(compressed_data)
return decompressed_data
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/compressed/file'
decompressed_data = decompress_binary_file(file_path)
在这个示例中,我们使用 zlib.decompress
方法将压缩的二进制数据解压缩。
七、处理自定义格式的二进制文件
对于自定义格式的二进制文件,通常需要根据文件格式规范编写解析代码。以下是一个示例,假设我们有一个自定义格式的文件,其中包含多个记录,每个记录包含一个整数和一个浮点数:
import struct
def parse_custom_binary_file(file_path):
records = []
try:
with open(file_path, 'rb') as file:
while True:
record_data = file.read(8) # 每个记录8字节
if not record_data:
break
record = struct.unpack('if', record_data)
records.append(record)
return records
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/custom/binary/file'
records = parse_custom_binary_file(file_path)
在这个示例中,我们假设每个记录包含一个整数和一个浮点数,总共占用8字节。我们使用 struct.unpack
方法解析每个记录,并将解析后的数据存储在 records
列表中。
八、处理二进制文件中的图像数据
有时候,我们需要从二进制文件中读取图像数据,并将其转换为可视化图像。以下是一个示例,使用 opencv
库读取二进制图像数据:
import cv2
import numpy as np
def read_image_from_binary_file(file_path, width, height, channels):
try:
with open(file_path, 'rb') as file:
data = file.read()
image = np.frombuffer(data, dtype=np.uint8)
image = image.reshape((height, width, channels))
return image
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/binary/image/file'
width, height, channels = 640, 480, 3 # 假设图像宽度640,高度480,RGB通道
image = read_image_from_binary_file(file_path, width, height, channels)
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
在这个示例中,我们使用 numpy.frombuffer
方法将二进制数据转换为 numpy
数组,并使用 reshape
方法将其转换为图像的形状。然后,我们使用 opencv
库的 imshow
方法显示图像。
九、处理二进制文件中的音频数据
有时候,我们需要从二进制文件中读取音频数据,并将其转换为可播放的音频。以下是一个示例,使用 pydub
库读取二进制音频数据:
from pydub import AudioSegment
import io
def read_audio_from_binary_file(file_path):
try:
with open(file_path, 'rb') as file:
data = file.read()
audio = AudioSegment.from_file(io.BytesIO(data), format="wav")
return audio
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/binary/audio/file'
audio = read_audio_from_binary_file(file_path)
audio.play()
在这个示例中,我们使用 pydub
库的 AudioSegment.from_file
方法将二进制数据转换为音频段,并使用 play
方法播放音频。
十、处理二进制文件中的视频数据
有时候,我们需要从二进制文件中读取视频数据,并将其转换为可播放的视频。以下是一个示例,使用 opencv
库读取二进制视频数据:
import cv2
import numpy as np
def read_video_from_binary_file(file_path, width, height):
try:
with open(file_path, 'rb') as file:
data = file.read()
video = np.frombuffer(data, dtype=np.uint8)
video = video.reshape((-1, height, width, 3)) # 假设视频每帧为RGB格式
return video
except FileNotFoundError:
print(f"File not found: {file_path}")
except IOError:
print(f"Error reading file: {file_path}")
file_path = 'path/to/your/binary/video/file'
width, height = 640, 480 # 假设视频每帧宽度640,高度480
video = read_video_from_binary_file(file_path, width, height)
for frame in video:
cv2.imshow('Frame', frame)
if cv2.waitKey(30) & 0xFF == ord('q'):
break
cv2.destroyAllWindows()
在这个示例中,我们使用 numpy.frombuffer
方法将二进制数据转换为 numpy
数组,并使用 reshape
方法将其转换为视频帧的形状。然后,我们使用 opencv
库的 imshow
方法显示每一帧。
通过以上方法,可以处理各种类型的二进制文件,包括图像、音频、视频、自定义格式等。在实际应用中,可以根据文件格式选择合适的方法进行处理。
相关问答FAQs:
如何在Python中打开和读取二进制文件?
在Python中,可以使用内置的open()
函数以二进制模式打开文件。可以使用'rb'
模式来读取二进制文件。打开文件后,可以使用read()
方法读取文件内容,或者使用readline()
和readlines()
来逐行读取。以下是一个简单的示例:
with open('yourfile.bin', 'rb') as file:
content = file.read()
这样你就可以读取整个二进制文件的内容。
读取二进制文件时需要注意哪些事项?
在读取二进制文件时,确保使用正确的模式打开文件。使用'rb'
模式可以避免文本编码问题。还需注意文件大小,如果文件非常大,可以分块读取,使用file.read(size)
方法指定每次读取的字节数,以避免占用过多内存。
如何将读取的二进制数据转换为其他格式?
读取的二进制数据通常是以字节形式呈现的。如果需要将其转换为其他格式,例如整数、浮点数或字符串,可以使用struct
模块。这个模块提供了方法来解析字节流并将其转换为不同的数据类型。示例代码如下:
import struct
with open('yourfile.bin', 'rb') as file:
data = file.read(4) # 假设我们读取4个字节
number = struct.unpack('i', data)[0] # 将字节转换为整数
根据实际文件的结构,选择合适的格式符来解析数据。
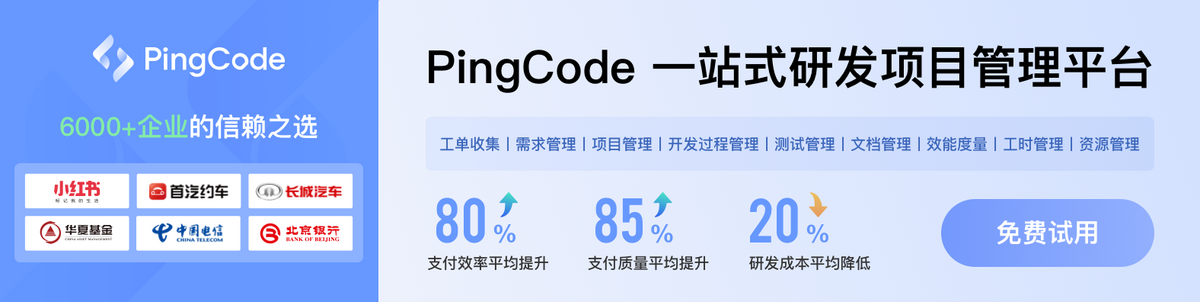