核心观点:使用字符串方法、使用正则表达式、遍历列表处理。在Python中,去掉列表中每个元素的标点符号的方法有多种,其中常见的方法包括使用字符串方法、使用正则表达式和遍历列表处理。下面将详细介绍其中一种方法,即使用正则表达式来实现。
使用正则表达式(Regular Expressions,简称regex)是一种强大的文本处理工具,可以方便地进行复杂的字符串匹配和替换操作。通过正则表达式,我们可以很容易地匹配和删除标点符号。
import re
列表示例
sample_list = ["Hello, World!", "Python is fun.", "Let's code:"]
去掉标点符号的函数
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
遍历列表并去掉每个元素的标点符号
cleaned_list = [remove_punctuation(item) for item in sample_list]
print(cleaned_list)
在这个例子中,我们定义了一个名为 remove_punctuation
的函数,该函数使用 re.sub
方法将字符串中的标点符号替换为空字符串。然后,我们使用列表推导式遍历列表中的每个元素,并调用 remove_punctuation
函数来处理每个元素。最终,我们得到了一个去掉了标点符号的新列表。
一、使用字符串方法去掉标点
除了使用正则表达式,我们还可以利用Python的字符串方法来去掉标点符号。字符串方法通常更简单,但处理起来可能不如正则表达式灵活。下面是一个示例:
import string
列表示例
sample_list = ["Hello, World!", "Python is fun.", "Let's code:"]
去掉标点符号的函数
def remove_punctuation(text):
return text.translate(str.maketrans('', '', string.punctuation))
遍历列表并去掉每个元素的标点符号
cleaned_list = [remove_punctuation(item) for item in sample_list]
print(cleaned_list)
在这个例子中,我们使用了 str.translate
方法来删除标点符号。str.maketrans
方法创建了一个映射表,该映射表将标点符号映射为空字符串。然后,我们遍历列表中的每个元素,并调用 remove_punctuation
函数来处理每个元素。
二、遍历列表处理标点
有时候,我们可能需要更灵活的处理方式,比如只删除某些特定的标点符号,或者在删除标点符号的同时保留空格等。此时,我们可以通过遍历列表和字符串的方式来进行处理:
# 列表示例
sample_list = ["Hello, World!", "Python is fun.", "Let's code:"]
定义需要去掉的标点符号
punctuation = ".,:;!?()[]{}'\""
去掉标点符号的函数
def remove_punctuation(text):
return ''.join(char for char in text if char not in punctuation)
遍历列表并去掉每个元素的标点符号
cleaned_list = [remove_punctuation(item) for item in sample_list]
print(cleaned_list)
在这个例子中,我们手动定义了一组需要去掉的标点符号,然后使用列表推导式遍历字符串的每个字符,并将不在标点符号列表中的字符拼接成一个新的字符串。这样可以更灵活地控制哪些标点符号需要去掉。
三、使用内置函数和库简化处理
Python还提供了一些内置函数和库,可以进一步简化去掉标点符号的操作。比如,可以使用 str.translate
方法结合 string.punctuation
来一次性去掉所有标点符号:
import string
列表示例
sample_list = ["Hello, World!", "Python is fun.", "Let's code:"]
去掉标点符号的函数
def remove_punctuation(text):
return text.translate(str.maketrans('', '', string.punctuation))
遍历列表并去掉每个元素的标点符号
cleaned_list = [remove_punctuation(item) for item in sample_list]
print(cleaned_list)
在这个例子中,string.punctuation
包含了所有常见的标点符号,而 str.maketrans
方法可以将这些标点符号映射为空字符串,从而实现去掉标点符号的效果。
四、处理不同语言的标点符号
需要注意的是,不同语言的标点符号可能有所不同。如果处理的文本包含多种语言的标点符号,我们可能需要考虑使用更复杂的正则表达式或者其他方法来处理。例如,中文文本中的标点符号包括逗号(,)、句号(。)、引号(“”)、书名号(《》)等。这些标点符号需要单独处理:
import re
列表示例
sample_list = ["Hello, World!", "Python is fun.", "你好,世界!", "编程很有趣。"]
去掉标点符号的函数
def remove_punctuation(text):
return re.sub(r'[^\w\s]|[_\u4e00-\u9fff]', '', text)
遍历列表并去掉每个元素的标点符号
cleaned_list = [remove_punctuation(item) for item in sample_list]
print(cleaned_list)
在这个例子中,我们在正则表达式中添加了对中文字符范围的匹配(\u4e00-\u9fff
),从而保留了中文字符并去掉标点符号。
五、综合使用不同方法
有时候,单一的方法可能无法满足所有需求。在这种情况下,我们可以综合使用不同的方法来处理标点符号。例如,可以先使用正则表达式去掉大部分标点符号,再使用字符串方法处理剩余的特殊情况:
import re
import string
列表示例
sample_list = ["Hello, World!", "Python is fun.", "你好,世界!", "编程很有趣。"]
去掉标点符号的函数
def remove_punctuation(text):
text = re.sub(r'[^\w\s]|[_\u4e00-\u9fff]', '', text)
return text.translate(str.maketrans('', '', string.punctuation))
遍历列表并去掉每个元素的标点符号
cleaned_list = [remove_punctuation(item) for item in sample_list]
print(cleaned_list)
在这个例子中,我们首先使用正则表达式去掉大部分标点符号,包括中文标点符号。然后,使用 str.translate
方法处理剩余的特殊标点符号,从而得到最终的结果。
六、处理标点符号的性能优化
在处理大规模文本数据时,性能可能成为一个重要的考虑因素。为了提高性能,可以尝试以下几种优化方法:
- 预编译正则表达式:如果需要多次使用相同的正则表达式,可以先将其预编译,以减少每次匹配时的开销。
import re
列表示例
sample_list = ["Hello, World!", "Python is fun.", "你好,世界!", "编程很有趣。"]
预编译正则表达式
pattern = re.compile(r'[^\w\s]|[_\u4e00-\u9fff]')
去掉标点符号的函数
def remove_punctuation(text):
return pattern.sub('', text)
遍历列表并去掉每个元素的标点符号
cleaned_list = [remove_punctuation(item) for item in sample_list]
print(cleaned_list)
- 使用生成器表达式:在处理大规模文本数据时,可以使用生成器表达式来节省内存。
import re
列表示例
sample_list = ["Hello, World!", "Python is fun.", "你好,世界!", "编程很有趣。"]
预编译正则表达式
pattern = re.compile(r'[^\w\s]|[_\u4e00-\u9fff]')
去掉标点符号的函数
def remove_punctuation(text):
return pattern.sub('', text)
使用生成器表达式处理列表
cleaned_list = (remove_punctuation(item) for item in sample_list)
将生成器转换为列表并打印
print(list(cleaned_list))
通过这些优化方法,可以显著提高处理大规模文本数据时的性能。
七、处理嵌套列表
在实际应用中,我们可能会遇到嵌套列表的情况。为了处理嵌套列表中的标点符号,可以使用递归函数来遍历列表中的每个元素,并进行处理:
import re
嵌套列表示例
nested_list = [["Hello, World!", "Python is fun."], ["你好,世界!", ["编程很有趣。", "Let's code:"]]]
预编译正则表达式
pattern = re.compile(r'[^\w\s]|[_\u4e00-\u9fff]')
去掉标点符号的函数
def remove_punctuation(text):
return pattern.sub('', text)
递归处理嵌套列表的函数
def process_nested_list(nested_list):
for i, item in enumerate(nested_list):
if isinstance(item, list):
process_nested_list(item)
elif isinstance(item, str):
nested_list[i] = remove_punctuation(item)
处理嵌套列表
process_nested_list(nested_list)
print(nested_list)
在这个例子中,我们定义了一个递归函数 process_nested_list
,该函数遍历嵌套列表中的每个元素。如果元素是列表,则递归调用 process_nested_list
;如果元素是字符串,则调用 remove_punctuation
进行处理。最终,我们得到了一个去掉了标点符号的嵌套列表。
八、处理其他特殊字符
除了标点符号,有时候我们还需要处理其他特殊字符,比如换行符、制表符等。可以在正则表达式中添加相应的匹配规则,或者使用字符串方法进行处理:
import re
列表示例
sample_list = ["Hello, World!\n", "Python\tis fun.", "你好,世界!\n", "编程很有趣。"]
去掉标点符号和特殊字符的函数
def remove_punctuation_and_special_chars(text):
text = re.sub(r'[^\w\s]|[_\u4e00-\u9fff]', '', text)
return text.replace('\n', '').replace('\t', '')
遍历列表并去掉每个元素的标点符号和特殊字符
cleaned_list = [remove_punctuation_and_special_chars(item) for item in sample_list]
print(cleaned_list)
在这个例子中,我们在正则表达式中去掉标点符号的同时,使用 str.replace
方法去掉了换行符和制表符。
通过以上多种方法,我们可以灵活地处理列表中的标点符号和其他特殊字符,以满足不同的需求。在实际应用中,可以根据具体情况选择合适的方法,或者综合使用多种方法,以获得最佳的处理效果。
相关问答FAQs:
如何在Python中去掉列表元素中的标点符号?
可以使用Python内置的字符串方法和列表推导式来实现。通过str.translate()
和str.maketrans()
方法,可以轻松去掉元素中的标点符号。示例代码如下:
import string
my_list = ["hello, world!", "python@2023", "good#morning!"]
cleaned_list = [s.translate(str.maketrans('', '', string.punctuation)) for s in my_list]
print(cleaned_list)
在处理字符串时,如何更好地处理不同语言的标点符号?
如果你的列表中包含多种语言的字符串,使用string.punctuation
可能不足以去掉所有的标点符号。可以考虑使用正则表达式模块re
,它支持更复杂的模式匹配。示例代码如下:
import re
my_list = ["你好,世界!", "Python@2023", "早上好!"]
cleaned_list = [re.sub(r'[^\w\s]', '', s) for s in my_list]
print(cleaned_list)
在清除标点符号后,如何确保列表中的元素保持原有的格式?
去掉标点符号后,元素的格式可能会发生变化,如果想保持特定的格式,可以在清除标点时考虑使用格式化方法。比如,如果要保留空格,可以在去掉标点的同时处理空格的数量或位置。可以结合使用str.strip()
或str.replace()
方法来达到目的。示例:
my_list = [" hello, world! ", "python@2023 ", "good#morning!"]
cleaned_list = [s.translate(str.maketrans('', '', string.punctuation)).strip() for s in my_list]
print(cleaned_list)
以上代码确保了去掉标点符号后,元素的前后空格被清除,保持了整洁的格式。
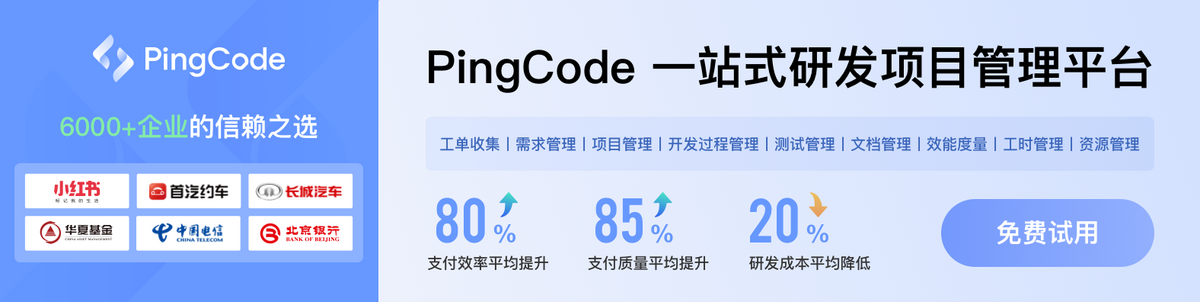