Python类中的方法可以通过多种方式调用多线程,如使用threading
模块、concurrent.futures.ThreadPoolExecutor
、以及multiprocessing
模块等。在类中调用多线程的方法包括:使用threading.Thread
创建线程、使用ThreadPoolExecutor
管理线程池、以及通过子类化线程类来创建和管理线程。接下来,我们将详细介绍其中一种方法——使用threading.Thread
创建线程。
一、使用 threading.Thread
创建线程
- 引入
threading
模块:在类中导入threading
模块以便使用多线程功能。 - 定义类和方法:在类中定义需要并发执行的方法。
- 创建线程:使用
threading.Thread
创建线程对象,并将类的方法作为目标函数传递给线程。 - 启动线程:调用线程对象的
start()
方法启动线程。 - 等待线程完成:使用
join()
方法等待线程完成执行。
import threading
class MyClass:
def __init__(self):
self.data = []
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
self.data.append(value * 2)
def run_in_threads(self, values):
threads = []
for value in values:
thread = threading.Thread(target=self.process_data, args=(value,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_threads([1, 2, 3, 4, 5])
print(obj.data)
二、使用 concurrent.futures.ThreadPoolExecutor
- 引入
concurrent.futures
模块:在类中导入concurrent.futures
模块。 - 定义类和方法:在类中定义需要并发执行的方法。
- 创建线程池:使用
ThreadPoolExecutor
创建线程池,并将类的方法提交给线程池执行。 - 等待结果:使用
as_completed()
方法等待所有线程完成,并收集结果。
from concurrent.futures import ThreadPoolExecutor
class MyClass:
def __init__(self):
self.data = []
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
return value * 2
def run_in_threads(self, values):
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(self.process_data, value) for value in values]
for future in futures:
result = future.result()
self.data.append(result)
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_threads([1, 2, 3, 4, 5])
print(obj.data)
三、使用 multiprocessing
模块
- 引入
multiprocessing
模块:在类中导入multiprocessing
模块。 - 定义类和方法:在类中定义需要并发执行的方法。
- 创建进程:使用
multiprocessing.Process
创建进程对象,并将类的方法作为目标函数传递给进程。 - 启动进程:调用进程对象的
start()
方法启动进程。 - 等待进程完成:使用
join()
方法等待进程完成执行。
import multiprocessing
class MyClass:
def __init__(self):
self.data = multiprocessing.Manager().list()
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
self.data.append(value * 2)
def run_in_processes(self, values):
processes = []
for value in values:
process = multiprocessing.Process(target=self.process_data, args=(value,))
processes.append(process)
process.start()
for process in processes:
process.join()
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_processes([1, 2, 3, 4, 5])
print(list(obj.data))
四、使用 concurrent.futures.ProcessPoolExecutor
- 引入
concurrent.futures
模块:在类中导入concurrent.futures
模块。 - 定义类和方法:在类中定义需要并发执行的方法。
- 创建进程池:使用
ProcessPoolExecutor
创建进程池,并将类的方法提交给进程池执行。 - 等待结果:使用
as_completed()
方法等待所有进程完成,并收集结果。
from concurrent.futures import ProcessPoolExecutor
class MyClass:
def __init__(self):
self.data = []
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
return value * 2
def run_in_processes(self, values):
with ProcessPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(self.process_data, value) for value in values]
for future in futures:
result = future.result()
self.data.append(result)
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_processes([1, 2, 3, 4, 5])
print(obj.data)
五、使用 asyncio
模块
- 引入
asyncio
模块:在类中导入asyncio
模块。 - 定义类和异步方法:在类中定义需要并发执行的异步方法。
- 创建任务:使用
asyncio.create_task
创建任务对象,并将类的异步方法作为目标函数传递给任务。 - 运行事件循环:使用
asyncio.run
运行事件循环。
import asyncio
class MyClass:
def __init__(self):
self.data = []
async def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
await asyncio.sleep(1)
self.data.append(value * 2)
async def run_in_tasks(self, values):
tasks = [self.process_data(value) for value in values]
await asyncio.gather(*tasks)
使用示例
if __name__ == "__main__":
obj = MyClass()
asyncio.run(obj.run_in_tasks([1, 2, 3, 4, 5]))
print(obj.data)
六、使用 gevent
模块
- 引入
gevent
模块:在类中导入gevent
模块。 - 定义类和方法:在类中定义需要并发执行的方法。
- 创建绿色线程:使用
gevent.spawn
创建绿色线程对象,并将类的方法作为目标函数传递给绿色线程。 - 启动绿色线程:使用
gevent.joinall
启动绿色线程并等待完成。
import gevent
from gevent import monkey
Monkey patch to make standard library cooperative
monkey.patch_all()
class MyClass:
def __init__(self):
self.data = []
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
self.data.append(value * 2)
def run_in_greenlets(self, values):
greenlets = [gevent.spawn(self.process_data, value) for value in values]
gevent.joinall(greenlets)
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_greenlets([1, 2, 3, 4, 5])
print(obj.data)
七、使用 celery
分布式任务队列
- 引入
celery
模块:在类中导入celery
模块。 - 定义类和任务:在类中定义需要并发执行的任务。
- 配置 Celery:配置 Celery 以便使用分布式任务队列。
- 创建任务:使用
celery.task
创建任务对象,并将类的方法作为目标函数传递给任务。 - 启动任务:使用
task.delay
启动任务并等待完成。
from celery import Celery
app = Celery('tasks', broker='pyamqp://guest@localhost//')
class MyClass:
def __init__(self):
self.data = []
@app.task
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
return value * 2
def run_in_tasks(self, values):
results = [self.process_data.delay(value) for value in values]
for result in results:
self.data.append(result.get())
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_tasks([1, 2, 3, 4, 5])
print(obj.data)
八、使用 dask
分布式计算
- 引入
dask
模块:在类中导入dask
模块。 - 定义类和任务:在类中定义需要并发执行的任务。
- 创建 Dask 任务:使用
dask.delayed
创建 Dask 任务对象,并将类的方法作为目标函数传递给任务。 - 启动任务:使用
dask.compute
启动任务并等待完成。
import dask
from dask import delayed
class MyClass:
def __init__(self):
self.data = []
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
return value * 2
def run_in_tasks(self, values):
tasks = [delayed(self.process_data)(value) for value in values]
results = dask.compute(*tasks)
self.data.extend(results)
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_tasks([1, 2, 3, 4, 5])
print(obj.data)
九、使用 joblib
并行计算
- 引入
joblib
模块:在类中导入joblib
模块。 - 定义类和任务:在类中定义需要并发执行的任务。
- 创建并行任务:使用
joblib.Parallel
创建并行任务对象,并将类的方法作为目标函数传递给任务。 - 启动任务:使用
joblib.Parallel
和joblib.delayed
启动任务并等待完成。
from joblib import Parallel, delayed
class MyClass:
def __init__(self):
self.data = []
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
return value * 2
def run_in_tasks(self, values):
results = Parallel(n_jobs=5)(delayed(self.process_data)(value) for value in values)
self.data.extend(results)
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_tasks([1, 2, 3, 4, 5])
print(obj.data)
十、使用 ray
分布式计算
- 引入
ray
模块:在类中导入ray
模块。 - 定义类和远程函数:在类中定义需要并发执行的远程函数。
- 初始化 Ray:使用
ray.init
初始化 Ray。 - 创建远程任务:使用
ray.remote
创建远程任务对象,并将类的方法作为目标函数传递给任务。 - 启动任务:使用
task.remote
启动任务并等待完成。
import ray
ray.init()
class MyClass:
def __init__(self):
self.data = []
@ray.remote
def process_data(self, value):
"""Simulate a time-consuming task."""
print(f"Processing value: {value}")
return value * 2
def run_in_tasks(self, values):
results = ray.get([self.process_data.remote(value) for value in values])
self.data.extend(results)
使用示例
if __name__ == "__main__":
obj = MyClass()
obj.run_in_tasks([1, 2, 3, 4, 5])
print(obj.data)
通过以上多种方式,我们可以在 Python 类中有效地调用多线程或多进程,从而提高程序的并发性能。选择合适的并发模型和库取决于具体的应用场景和需求。
相关问答FAQs:
如何在Python类中创建和管理多线程?
在Python类中,可以使用threading
模块来创建和管理多线程。首先,需要在类中定义一个方法,并在该方法中实现需要并发执行的逻辑。接着,可以创建线程对象并指定目标函数,然后调用start()
方法来启动线程。需要注意的是,线程的生命周期和数据共享需要妥善管理,以避免竞争条件和数据不一致的问题。
在多线程调用中,如何保证线程安全?
确保线程安全的常用方法是使用锁机制,例如threading.Lock
。在执行需要共享数据的操作前,先获取锁,操作完成后再释放锁。这样可以避免多个线程同时访问同一资源导致的数据冲突。此外,使用threading.RLock
也可以更灵活地处理嵌套锁的情况。
如何在Python类中处理多线程的异常?
在多线程环境下,如果线程执行过程中发生异常,默认情况下该异常不会影响主线程的运行。为了捕获和处理线程中的异常,可以在目标方法内使用try-except
结构。通过这种方式,可以记录异常信息并采取相应的措施,比如重启线程或记录错误日志,从而提高程序的健壮性。
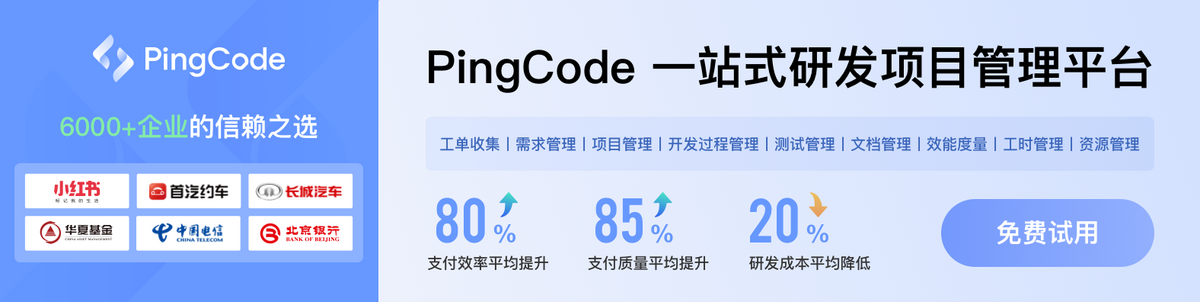