利用Python爬取优惠券的步骤主要有:选择目标网站、分析网页结构、使用请求库获取网页内容、解析网页提取数据、存储数据、处理反爬虫机制。选择目标网站、分析网页结构、使用请求库获取网页内容、解析网页提取数据、存储数据、处理反爬虫机制,其中选择合适的请求库和解析库是关键步骤。接下来,我将详细描述如何实现这些步骤。
一、选择目标网站
首先,我们需要确定想要爬取优惠券的目标网站。目标网站通常是一些电商平台、优惠券聚合网站或者品牌官网。在选择目标网站时,需要注意其是否允许爬虫操作,最好查看其 robots.txt
文件以确认爬虫权限。
二、分析网页结构
在确定目标网站后,我们需要分析网页的HTML结构,以便找出存放优惠券信息的具体位置。使用浏览器的开发者工具(F12)可以查看网页的DOM结构,找到我们需要的优惠券数据所在的标签和属性。
<div class="coupon">
<p class="coupon-code">SAVE10</p>
<p class="coupon-desc">Get 10% off on your next purchase</p>
</div>
例如,上述代码片段显示了一个优惠券的HTML结构,其中优惠券代码和描述分别存放在 p
标签中。
三、使用请求库获取网页内容
接下来,我们使用Python的请求库(如 requests
)来获取网页内容。以下是一个示例代码:
import requests
url = "https://www.example.com/coupons"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
response = requests.get(url, headers=headers)
检查请求是否成功
if response.status_code == 200:
html_content = response.text
else:
print("Failed to retrieve the webpage")
在这个例子中,我们发送了一个HTTP GET请求来获取目标网页的HTML内容,并检查请求是否成功。
四、解析网页提取数据
获取网页内容后,我们需要解析HTML并提取优惠券数据。通常使用 BeautifulSoup
或 lxml
库来解析HTML。以下是使用 BeautifulSoup
的示例代码:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
coupons = []
for coupon_div in soup.find_all('div', class_='coupon'):
code = coupon_div.find('p', class_='coupon-code').text
desc = coupon_div.find('p', class_='coupon-desc').text
coupons.append({'code': code, 'description': desc})
打印提取的优惠券信息
for coupon in coupons:
print(f"Code: {coupon['code']}, Description: {coupon['description']}")
在这个示例中,我们使用 BeautifulSoup
解析HTML,并提取包含优惠券信息的 div
标签。然后,我们将提取到的优惠券代码和描述存储在一个字典中。
五、存储数据
提取优惠券数据后,我们需要将其存储在合适的存储介质中,例如数据库、CSV文件或JSON文件。以下是存储为CSV文件的示例代码:
import csv
with open('coupons.csv', mode='w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['Code', 'Description'])
for coupon in coupons:
writer.writerow([coupon['code'], coupon['description']])
在这个示例中,我们使用 csv
库将提取到的优惠券数据写入一个CSV文件。
六、处理反爬虫机制
许多网站会有反爬虫机制来防止爬虫程序的访问。常见的反爬措施包括:请求频率限制、IP封禁、验证码等。以下是一些应对策略:
- 请求频率控制:通过增加请求之间的间隔时间,避免触发频率限制。
- 代理IP轮换:使用代理池进行IP轮换,避免单个IP被封禁。
- 模拟用户行为:通过设置合适的HTTP头部(如 User-Agent)、随机点击等方式,模拟真实用户的浏览行为。
- 处理验证码:可以使用OCR技术或手动输入验证码,绕过验证码机制。
以下是一个使用 time
模块控制请求频率的示例代码:
import time
for i in range(len(coupons)):
# 发送请求并处理响应
time.sleep(2) # 等待2秒
七、代码优化和错误处理
为了使爬虫程序更加健壮,我们需要加入错误处理机制。例如,使用 try
和 except
块来捕获和处理异常,避免程序在遇到错误时崩溃。
import requests
from bs4 import BeautifulSoup
import csv
import time
url = "https://www.example.com/coupons"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
coupons = []
try:
response = requests.get(url, headers=headers)
response.raise_for_status() # 检查HTTP请求是否成功
soup = BeautifulSoup(response.text, 'html.parser')
for coupon_div in soup.find_all('div', class_='coupon'):
code = coupon_div.find('p', class_='coupon-code').text
desc = coupon_div.find('p', class_='coupon-desc').text
coupons.append({'code': code, 'description': desc})
time.sleep(2) # 等待2秒
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}")
except Exception as e:
print(f"An error occurred: {e}")
存储数据
with open('coupons.csv', mode='w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['Code', 'Description'])
for coupon in coupons:
writer.writerow([coupon['code'], coupon['description']])
在这个示例中,我们在发送HTTP请求和解析HTML时加入了错误处理机制,以确保程序能够在遇到错误时正常处理并继续执行。
八、进一步优化
要进一步优化爬虫程序,我们可以考虑以下几个方面:
- 多线程或异步爬取:使用多线程或异步编程技术来提高爬取效率。
- 数据清洗和去重:对提取的数据进行清洗和去重,确保数据质量。
- 动态加载处理:对于动态加载的页面,可以使用Selenium或Playwright等浏览器自动化工具来处理。
以下是一个使用 threading
模块进行多线程爬取的示例代码:
import threading
def fetch_coupons(url):
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
soup = BeautifulSoup(response.text, 'html.parser')
for coupon_div in soup.find_all('div', class_='coupon'):
code = coupon_div.find('p', class_='coupon-code').text
desc = coupon_div.find('p', class_='coupon-desc').text
coupons.append({'code': code, 'description': desc})
time.sleep(2)
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}")
except Exception as e:
print(f"An error occurred: {e}")
创建线程
threads = []
for i in range(5): # 假设有5个页面需要爬取
thread = threading.Thread(target=fetch_coupons, args=(url,))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
存储数据
with open('coupons.csv', mode='w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['Code', 'Description'])
for coupon in coupons:
writer.writerow([coupon['code'], coupon['description']])
通过使用多线程技术,我们可以同时发送多个请求,从而提高爬取效率。
总结
通过以上步骤,我们可以利用Python爬取优惠券数据。主要步骤包括选择目标网站、分析网页结构、使用请求库获取网页内容、解析网页提取数据、存储数据、处理反爬虫机制以及进一步优化爬虫程序。希望这些内容对你有所帮助。
相关问答FAQs:
如何选择合适的Python库来爬取优惠券数据?
在进行优惠券数据爬取时,选择合适的Python库至关重要。常用的库包括BeautifulSoup和Scrapy。BeautifulSoup适合小规模爬虫,易于上手,适合处理HTML和XML文档。而Scrapy则是一个功能强大的框架,适合大规模爬虫项目,支持异步处理,可以高效抓取和解析数据。根据您的需求,选择合适的库将帮助您更高效地完成任务。
爬取优惠券数据时需要注意哪些法律和伦理问题?
在进行数据爬取时,务必遵循相关法律法规和网站的使用条款。很多网站在其robots.txt文件中明确规定了哪些内容可以被爬取,哪些内容不可以。在爬取之前,了解并遵守这些规定是十分重要的。此外,过于频繁的请求可能会导致您的IP被封禁,因此应当设置合理的请求间隔,避免对目标网站造成负担。
如何处理爬取到的优惠券数据以便后续分析?
在成功爬取优惠券数据后,您可能需要对数据进行清洗和整理,以便后续分析。可以使用Pandas库对数据进行处理,方便进行筛选和分析。同时,可以将数据存储在CSV、Excel或数据库中,以便于后续的数据挖掘与分析。确保对数据进行去重和格式化,以提高数据质量和分析的准确性。
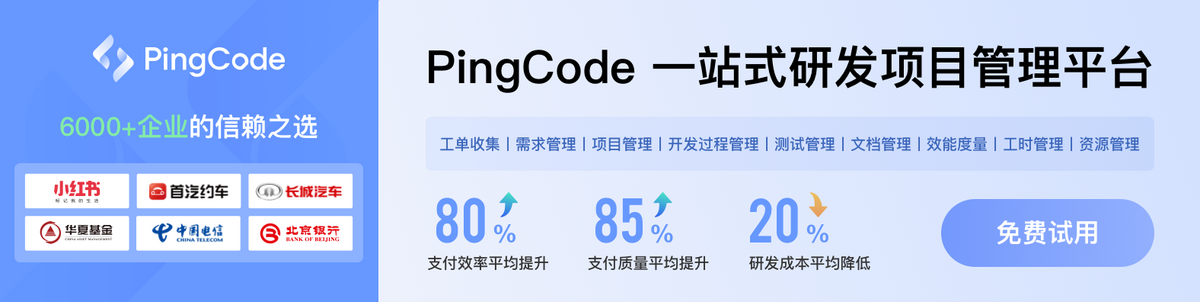