使用Python画出折线统计图的方法主要包括:利用Matplotlib库、设置图形标签、调整图形样式、保存图形文件。本文将详细阐述这些步骤及一些高级技巧。
一、MATPLOTLIB库的安装与基本使用
Matplotlib是Python中一个非常强大的绘图库,支持多种图表的绘制。首先,我们需要安装Matplotlib库。可以通过pip安装:
pip install matplotlib
安装完成后,我们可以通过以下代码绘制一个最简单的折线统计图:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图
plt.plot(x, y)
显示图形
plt.show()
在上述代码中,plt.plot(x, y)
是绘制折线图的基本命令,其中x和y是数据点的横纵坐标。plt.show()
显示出图形。
二、设置图形标签
为了使图形更加清晰明了,我们可以添加标题、坐标轴标签和图例。例如:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图
plt.plot(x, y)
添加标题和坐标轴标签
plt.title('Sample Line Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
这样,我们就给图形加上了标题和坐标轴标签,使得图形更加易于理解。
三、调整图形样式
Matplotlib提供了丰富的样式选项,可以调整折线的颜色、线型、标记等。例如:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图,设置线条颜色、线型和标记
plt.plot(x, y, color='green', linestyle='--', marker='o')
添加标题和坐标轴标签
plt.title('Styled Line Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
在上述代码中,color
参数设置线条颜色,linestyle
参数设置线型,marker
参数设置数据点的标记。
四、保存图形文件
有时候我们需要将图形保存为文件。可以通过 savefig
函数来实现:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图
plt.plot(x, y)
添加标题和坐标轴标签
plt.title('Save Plot Example')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
保存图形文件
plt.savefig('line_plot.png')
显示图形
plt.show()
在上述代码中,plt.savefig('line_plot.png')
将图形保存为PNG格式的文件。
五、添加图例
如果我们在一张图表中绘制多条线,为了区分不同的数据线,可以添加图例。例如:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y1 = [2, 3, 5, 7, 11]
y2 = [1, 4, 6, 8, 10]
绘制多条折线图
plt.plot(x, y1, label='Data 1', color='blue')
plt.plot(x, y2, label='Data 2', color='red')
添加标题、坐标轴标签和图例
plt.title('Multiple Lines Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
plt.legend()
显示图形
plt.show()
在上述代码中,label
参数用于指定图例的名称,plt.legend()
添加图例。
六、绘制带误差的折线图
有时候我们的数据带有误差,我们可以使用误差条来表示数据的不确定性。例如:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
yerr = [0.5, 0.4, 0.3, 0.2, 0.1]
绘制带误差条的折线图
plt.errorbar(x, y, yerr=yerr, fmt='-o', color='blue', ecolor='red', capsize=5)
添加标题和坐标轴标签
plt.title('Error Bar Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
在上述代码中,plt.errorbar
用于绘制带误差条的折线图,yerr
参数指定误差值,fmt
参数指定线型和标记,ecolor
参数指定误差条的颜色,capsize
参数指定误差条帽的大小。
七、设置坐标轴范围和刻度
我们可以通过 xlim
和 ylim
函数来设置坐标轴的范围,通过 xticks
和 yticks
函数来设置坐标轴的刻度。例如:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图
plt.plot(x, y)
设置坐标轴范围
plt.xlim(0, 6)
plt.ylim(0, 12)
设置坐标轴刻度
plt.xticks([0, 1, 2, 3, 4, 5, 6])
plt.yticks([0, 2, 4, 6, 8, 10, 12])
添加标题和坐标轴标签
plt.title('Custom Axis Range and Ticks')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
在上述代码中,plt.xlim
和 plt.ylim
设置坐标轴的范围,plt.xticks
和 plt.yticks
设置坐标轴的刻度。
八、使用子图
有时候我们需要在一张图中绘制多个子图,可以使用 subplot
函数。例如:
import matplotlib.pyplot as plt
定义数据
x = [1, 2, 3, 4, 5]
y1 = [2, 3, 5, 7, 11]
y2 = [1, 4, 6, 8, 10]
创建子图
plt.subplot(2, 1, 1)
plt.plot(x, y1, color='blue')
plt.title('Subplot 1')
plt.subplot(2, 1, 2)
plt.plot(x, y2, color='red')
plt.title('Subplot 2')
调整子图间距
plt.tight_layout()
显示图形
plt.show()
在上述代码中,plt.subplot(2, 1, 1)
创建一个2行1列的子图,并绘制第一个子图,plt.subplot(2, 1, 2)
绘制第二个子图,plt.tight_layout()
调整子图间距。
九、动态更新图形
有时候我们需要动态更新图形,例如实时显示数据。可以使用 FuncAnimation
函数。例如:
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
定义数据
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x)
fig, ax = plt.subplots()
line, = ax.plot(x, y)
def update(frame):
line.set_ydata(np.sin(x + frame / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, blit=True)
plt.show()
在上述代码中,FuncAnimation
函数用于创建动画,update
函数用于更新图形数据。
以上是使用Python绘制折线统计图的详细步骤及一些高级技巧,通过这些方法可以实现多样化的图形展示。在实际应用中,根据具体需求选择合适的方法和参数,以达到最佳的视觉效果。
相关问答FAQs:
如何选择合适的库来绘制折线统计图?
在Python中,有多个库可以用来绘制折线统计图,其中最常用的是Matplotlib和Seaborn。Matplotlib是一个强大的绘图库,适合创建各种类型的图表,包括折线图。Seaborn则在Matplotlib的基础上进行了扩展,提供了更美观的默认样式和更简便的接口。如果您需要快速实现且不太关注图表的外观,Matplotlib是个不错的选择;而如果您想要更专业的可视化效果,Seaborn将会是更好的选择。
如何准备数据以便绘制折线统计图?
在绘制折线统计图之前,您需要将数据准备成适合的格式。通常情况下,数据应当以两个列表或者数组的形式存在,其中一个代表x轴的数据(如时间或类别),另一个代表y轴的数据(如数值)。确保数据的长度一致,且数据类型适合进行数值运算,通常使用Pandas库来处理数据会更加高效与方便。
如何自定义折线图的样式和标签?
在使用Matplotlib或Seaborn绘制折线图时,您可以通过各种参数自定义图表的样式。您可以设置线条的颜色、线型、宽度等属性。此外,添加图例、标题和坐标轴标签也是提升图表可读性的关键步骤。使用plt.title()
、plt.xlabel()
和plt.ylabel()
等方法可以轻松实现这些自定义。对于更复杂的可视化,Seaborn还支持调色板和主题的设置,使得图表更加美观。
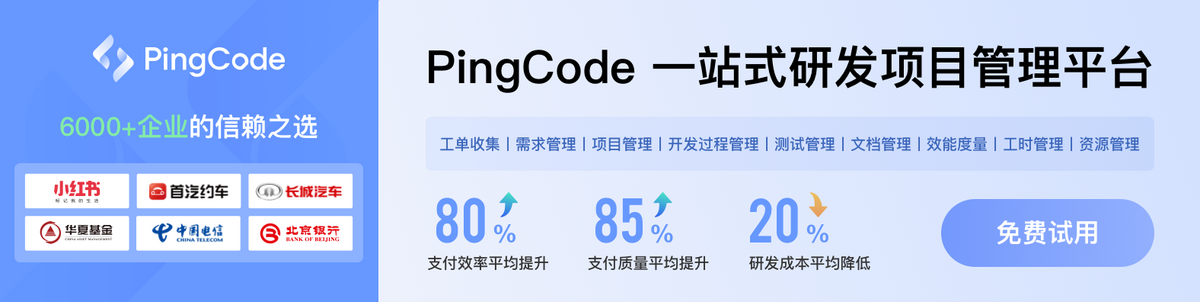