PYTHON如何判断是新一天
使用日期时间模块、比较当前时间和上次记录时间、使用文件或数据库存储上次记录时间。在Python中,可以通过日期时间模块来判断是否是新的一天。具体方法是将当前时间与上次记录的时间进行比较,如果当前时间的日期与上次记录的日期不同,则说明已经是新的一天了。下面详细介绍一种方法来实现这一逻辑。
一、使用日期时间模块
Python的日期时间模块datetime
非常强大,它提供了许多方法来处理日期和时间。可以使用这个模块来获取当前时间,并将其与之前记录的时间进行比较。
import datetime
获取当前日期
current_date = datetime.date.today()
假设上次记录的日期是昨天
last_recorded_date = current_date - datetime.timedelta(days=1)
判断是否是新的一天
if current_date != last_recorded_date:
print("是新的一天")
else:
print("不是新的一天")
在这个例子中,使用datetime.date.today()
函数获取当前日期,并用datetime.timedelta
函数减去一天,模拟上次记录的日期。然后比较两个日期,如果不同则表示是新的一天。
二、比较当前时间和上次记录时间
将当前时间与上次记录的时间进行比较是判断是否是新的一天的关键步骤。为了确保数据的持久性,可以将上次记录的时间保存在文件或数据库中。
import datetime
def is_new_day(last_recorded_date_str):
# 获取当前日期
current_date = datetime.date.today()
# 将字符串转换为日期对象
last_recorded_date = datetime.datetime.strptime(last_recorded_date_str, '%Y-%m-%d').date()
# 比较日期
if current_date != last_recorded_date:
return True
else:
return False
假设上次记录的日期是昨天
last_recorded_date_str = '2023-10-10'
判断是否是新的一天
if is_new_day(last_recorded_date_str):
print("是新的一天")
else:
print("不是新的一天")
在这个例子中,使用datetime.datetime.strptime
函数将上次记录的日期字符串转换为日期对象,然后与当前日期进行比较。如果日期不同,则表示是新的一天。
三、使用文件或数据库存储上次记录时间
为了确保上次记录的时间能够持久化存储,可以使用文件或数据库来存储。以下是一个使用文件存储上次记录时间的例子。
使用文件存储上次记录时间
import datetime
import os
def get_last_recorded_date(file_path):
if os.path.exists(file_path):
with open(file_path, 'r') as file:
date_str = file.read().strip()
return datetime.datetime.strptime(date_str, '%Y-%m-%d').date()
else:
return None
def save_current_date(file_path):
with open(file_path, 'w') as file:
file.write(datetime.date.today().strftime('%Y-%m-%d'))
def is_new_day(file_path):
last_recorded_date = get_last_recorded_date(file_path)
current_date = datetime.date.today()
if last_recorded_date is None or current_date != last_recorded_date:
save_current_date(file_path)
return True
else:
return False
定义存储日期的文件路径
file_path = 'last_recorded_date.txt'
判断是否是新的一天
if is_new_day(file_path):
print("是新的一天")
else:
print("不是新的一天")
在这个例子中,get_last_recorded_date
函数用于读取存储在文件中的上次记录日期,save_current_date
函数用于将当前日期保存到文件中。is_new_day
函数用于判断是否是新的一天,如果是新的一天,则会更新文件中的日期。
四、使用数据库存储上次记录时间
如果项目中已经使用了数据库,那么可以将上次记录的时间存储在数据库中。以下是一个使用SQLite数据库的例子。
import sqlite3
import datetime
def get_last_recorded_date(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute('CREATE TABLE IF NOT EXISTS record_date (date TEXT)')
cursor.execute('SELECT date FROM record_date ORDER BY rowid DESC LIMIT 1')
row = cursor.fetchone()
conn.close()
if row is not None:
return datetime.datetime.strptime(row[0], '%Y-%m-%d').date()
else:
return None
def save_current_date(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute('INSERT INTO record_date (date) VALUES (?)', (datetime.date.today().strftime('%Y-%m-%d'),))
conn.commit()
conn.close()
def is_new_day(db_path):
last_recorded_date = get_last_recorded_date(db_path)
current_date = datetime.date.today()
if last_recorded_date is None or current_date != last_recorded_date:
save_current_date(db_path)
return True
else:
return False
定义数据库文件路径
db_path = 'record_date.db'
判断是否是新的一天
if is_new_day(db_path):
print("是新的一天")
else:
print("不是新的一天")
在这个例子中,使用SQLite数据库来存储上次记录的日期。get_last_recorded_date
函数用于从数据库中读取上次记录的日期,save_current_date
函数用于将当前日期保存到数据库中,is_new_day
函数用于判断是否是新的一天,并在需要时更新数据库中的日期。
五、处理跨时区问题
在实际应用中,如果系统涉及到跨时区的问题,需要特别注意。可以使用Python的pytz
库来处理时区转换。
import datetime
import pytz
def is_new_day_in_timezone(last_recorded_date_str, timezone_str):
# 设置时区
timezone = pytz.timezone(timezone_str)
# 获取当前日期和时间,并转换为指定时区
current_datetime = datetime.datetime.now(timezone)
current_date = current_datetime.date()
# 将字符串转换为日期对象
last_recorded_date = datetime.datetime.strptime(last_recorded_date_str, '%Y-%m-%d').date()
# 比较日期
if current_date != last_recorded_date:
return True
else:
return False
假设上次记录的日期是昨天
last_recorded_date_str = '2023-10-10'
timezone_str = 'America/New_York'
判断是否是新的一天
if is_new_day_in_timezone(last_recorded_date_str, timezone_str):
print("是新的一天")
else:
print("不是新的一天")
在这个例子中,使用pytz
库设置时区,并将当前时间转换为指定时区的时间。然后比较日期是否相同,如果不同则表示是新的一天。
六、总结
Python中判断是否是新的一天可以通过多种方法实现,主要包括使用日期时间模块、比较当前时间和上次记录时间、使用文件或数据库存储上次记录时间,以及处理跨时区问题。关键在于获取当前日期,并将其与上次记录的日期进行比较。如果不同,则表示是新的一天。在实际应用中,可以根据具体需求选择合适的方法来实现这一逻辑。
相关问答FAQs:
如何在Python中判断当前时间是否是新的一天?
在Python中,可以通过获取当前时间和之前记录的时间进行比较。如果当前时间的日期部分与之前记录的日期部分不同,就可以判断为新的一天。可以使用datetime
模块来实现这一功能。示例代码如下:
from datetime import datetime
# 假设这是之前记录的时间
previous_time = datetime(2023, 10, 1, 10, 0, 0)
# 获取当前时间
current_time = datetime.now()
# 判断是否是新的一天
if current_time.date() != previous_time.date():
print("是新的一天")
else:
print("还不是新的一天")
如何在Python中获取当前日期?
获取当前日期非常简单,可以使用datetime
模块中的datetime.now()
方法。该方法返回当前的日期和时间。使用.date()
方法可以提取日期部分。以下是获取当前日期的示例代码:
from datetime import datetime
# 获取当前时间
current_time = datetime.now()
# 提取当前日期
current_date = current_time.date()
print("当前日期是:", current_date)
如何在Python中设置和比较日期?
在Python中,使用datetime
模块不仅可以获取当前日期,还可以设置特定的日期进行比较。可以通过datetime
类创建自定义日期,然后与当前日期进行比较。示例代码如下:
from datetime import datetime
# 设置一个特定日期
specific_date = datetime(2023, 10, 1).date()
# 获取当前日期
current_date = datetime.now().date()
# 比较日期
if current_date > specific_date:
print("当前日期在特定日期之后")
elif current_date < specific_date:
print("当前日期在特定日期之前")
else:
print("当前日期与特定日期相同")
这些方法可以帮助用户轻松判断是否进入新的一天以及处理日期的比较问题。
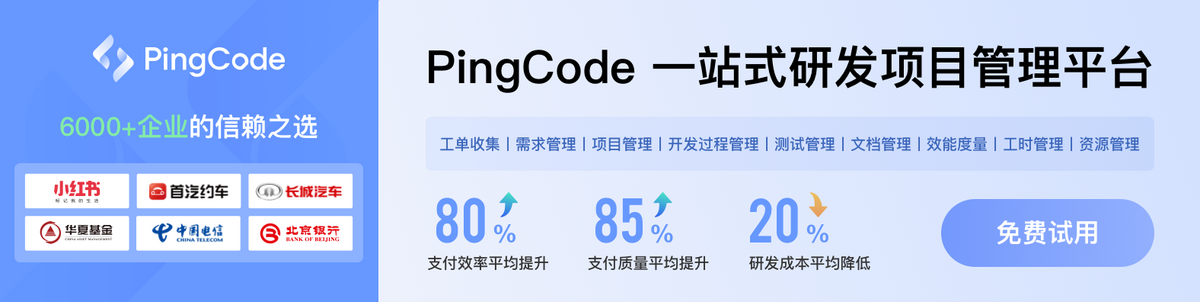