要用Python计算圆柱体的体积,你需要知道圆柱体的半径和高度。体积的计算公式是:V = π * r² * h。首先,我们可以利用Python中的数学模块(math module)来处理π的值。使用Python计算圆柱体体积的步骤如下:
import math
def calculate_cylinder_volume(radius, height):
volume = math.pi * radius 2 * height
return volume
Example usage
radius = 5
height = 10
volume = calculate_cylinder_volume(radius, height)
print(f"The volume of the cylinder is: {volume}")
在这段代码中,首先导入了math模块,然后定义了一个函数calculate_cylinder_volume
来计算圆柱体的体积。接着,利用这个函数,传入半径和高度的值,计算并输出体积。
一、圆柱体体积计算基础
计算圆柱体的体积需要使用基本的几何公式。圆柱体的体积(V)等于底面积(A)乘以高度(h)。底面积是一个圆形的面积,其公式为A = π * r²。因此,圆柱体的体积公式为V = π * r² * h。
1、理解圆柱体体积的公式
圆柱体的体积公式 V = π * r² * h,这里的每一个符号都代表特定的含义:
- V 表示体积(Volume)
- π 是圆周率,约等于3.14159
- r 是圆的半径(radius)
- h 是圆柱体的高度(height)
2、使用Python中的math模块
Python中的math模块提供了许多数学函数和常量,包括圆周率π。我们可以使用math.pi来表示π,从而提高计算的准确性。
import math
定义计算圆柱体体积的函数
def calculate_cylinder_volume(radius, height):
volume = math.pi * radius 2 * height
return volume
二、详细代码实现
在实际应用中,可能需要处理用户输入、输入验证等情况。以下是一个更完整的示例,包括用户输入和错误处理。
1、定义函数和处理用户输入
首先,定义一个函数来计算圆柱体的体积,并处理用户输入。
def get_user_input(prompt):
while True:
try:
value = float(input(prompt))
if value <= 0:
raise ValueError("The value must be positive.")
return value
except ValueError as e:
print(e)
def calculate_cylinder_volume(radius, height):
volume = math.pi * radius 2 * height
return volume
def main():
print("Calculate the volume of a cylinder")
radius = get_user_input("Enter the radius of the base: ")
height = get_user_input("Enter the height of the cylinder: ")
volume = calculate_cylinder_volume(radius, height)
print(f"The volume of the cylinder is: {volume}")
if __name__ == "__main__":
main()
2、验证用户输入
确保用户输入的是正数,并且能够处理错误输入。函数get_user_input
循环提示用户输入,直到输入一个正数为止。
def get_user_input(prompt):
while True:
try:
value = float(input(prompt))
if value <= 0:
raise ValueError("The value must be positive.")
return value
except ValueError as e:
print(e)
三、优化和扩展
在实际项目中,可能需要进一步优化代码,并考虑更多的应用场景。例如,可以将计算函数模块化,便于在其他程序中调用;可以添加更多的功能,如计算圆柱体的表面积等。
1、模块化代码
将计算圆柱体体积的功能封装到一个模块中,便于重用。
# cylinder.py
import math
def calculate_cylinder_volume(radius, height):
if radius <= 0 or height <= 0:
raise ValueError("Radius and height must be positive.")
return math.pi * radius 2 * height
在主程序中导入该模块并使用。
# main.py
from cylinder import calculate_cylinder_volume
def main():
print("Calculate the volume of a cylinder")
radius = float(input("Enter the radius of the base: "))
height = float(input("Enter the height of the cylinder: "))
try:
volume = calculate_cylinder_volume(radius, height)
print(f"The volume of the cylinder is: {volume}")
except ValueError as e:
print(e)
if __name__ == "__main__":
main()
2、计算表面积
除了计算体积外,还可以计算圆柱体的表面积。表面积包括两个圆的面积和圆柱侧面的面积。公式为:A = 2πr² + 2πrh。
import math
def calculate_cylinder_surface_area(radius, height):
if radius <= 0 or height <= 0:
raise ValueError("Radius and height must be positive.")
base_area = 2 * math.pi * radius 2
side_area = 2 * math.pi * radius * height
return base_area + side_area
在主程序中添加计算表面积的功能。
from cylinder import calculate_cylinder_volume, calculate_cylinder_surface_area
def main():
print("Calculate the volume and surface area of a cylinder")
radius = float(input("Enter the radius of the base: "))
height = float(input("Enter the height of the cylinder: "))
try:
volume = calculate_cylinder_volume(radius, height)
surface_area = calculate_cylinder_surface_area(radius, height)
print(f"The volume of the cylinder is: {volume}")
print(f"The surface area of the cylinder is: {surface_area}")
except ValueError as e:
print(e)
if __name__ == "__main__":
main()
四、实际应用场景
在实际项目中,计算圆柱体体积的应用非常广泛。以下是几个实际应用场景:
1、工业设计
在工业设计中,经常需要计算各种容器的体积。通过编写Python脚本,可以快速计算出设计中各种圆柱体容器的体积,帮助设计师进行优化。
2、科学研究
在科学研究中,特别是化学和物理实验中,常常需要计算实验容器的体积。使用Python脚本,可以快速准确地进行计算,减少人为误差。
3、教育教学
在教育教学中,可以通过编写Python脚本,帮助学生理解几何体积的计算方法。学生可以通过实际操作,更好地理解几何公式的应用。
五、总结
通过上述内容,我们详细介绍了如何用Python计算圆柱体的体积。我们从基础公式入手,逐步扩展到实际应用场景。同时,通过模块化代码和错误处理,提高了代码的复用性和可靠性。希望这些内容能够帮助到大家,更好地理解和应用Python进行几何计算。
相关问答FAQs:
圆柱体的体积计算公式是什么?
圆柱体的体积可以通过公式 V = πr²h 计算,其中 V 表示体积,r 是圆柱底面的半径,h 是圆柱的高度。π(圆周率)通常取值为3.14或使用 Python 的 math 库中的 math.pi,可以提供更高的精度。
在 Python 中如何实现圆柱体体积的计算?
使用 Python 计算圆柱体体积相对简单。可以通过定义一个函数来接收半径和高度作为参数,并返回计算出的体积。例如:
import math
def cylinder_volume(radius, height):
return math.pi * radius ** 2 * height
这样,你可以通过调用 cylinder_volume(radius, height)
来得到圆柱体的体积。
如何处理用户输入以计算圆柱体的体积?
为了让程序更具互动性,可以使用 input()
函数来获取用户输入的半径和高度。可以将输入转换为浮点数,以确保计算的准确性。示例代码如下:
radius = float(input("请输入圆柱体的半径: "))
height = float(input("请输入圆柱体的高度: "))
volume = cylinder_volume(radius, height)
print(f"圆柱体的体积是: {volume}")
以上代码将提示用户输入所需的值,并输出计算结果。
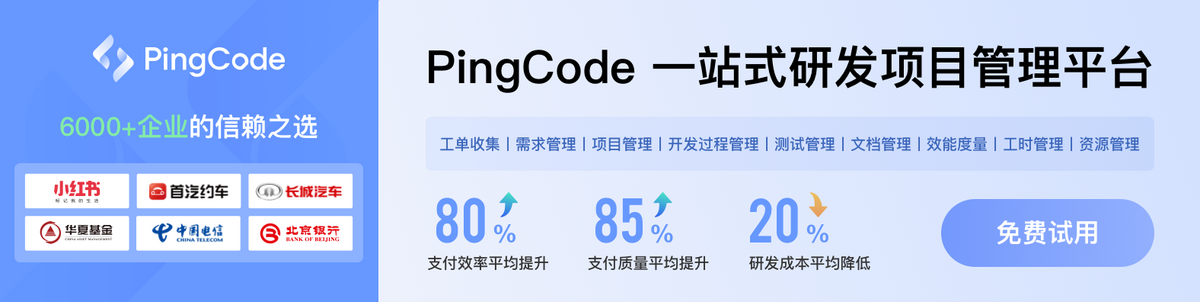