在Python中,输入字符串和输出字符串的方法包括使用input()
函数获取用户输入、使用print()
函数输出字符串、可以对字符串进行格式化输出、对输入输出进行字符串操作。 其中,print()
函数是输出字符串的主要方法,通过该函数可以将任何类型的数据转换为字符串输出。
Python提供了很多方法来处理字符串输入和输出。接下来,我们将详细介绍这些方法。
一、字符串输入
1. 使用input()
函数
input()
函数用于从用户获取输入。这个函数会暂停程序,等待用户输入并按下回车键,然后将输入作为字符串返回。
user_input = input("请输入字符串:")
print("你输入的字符串是:", user_input)
2. 从文件读取输入
Python提供了多种方法来从文件读取字符串,例如使用open()
函数和文件对象的read()
, readline()
, readlines()
方法。
with open('example.txt', 'r') as file:
content = file.read()
print("文件内容:", content)
二、字符串输出
1. 使用print()
函数
print()
函数用于将字符串输出到控制台。
print("Hello, World!")
2. 字符串格式化输出
Python提供了多种字符串格式化方法,包括使用百分号(%)进行格式化、str.format()
方法和f-字符串(Python 3.6及以上)。
百分号格式化:
name = "Alice"
age = 30
print("名字: %s, 年龄: %d" % (name, age))
str.format()
方法:
name = "Alice"
age = 30
print("名字: {}, 年龄: {}".format(name, age))
f-字符串:
name = "Alice"
age = 30
print(f"名字: {name}, 年龄: {age}")
3. 输出到文件
可以使用open()
函数和文件对象的write()
方法将字符串输出到文件。
with open('output.txt', 'w') as file:
file.write("Hello, World!")
三、字符串操作
1. 字符串拼接
可以使用加号(+)进行字符串拼接。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result)
2. 字符串分割
可以使用split()
方法将字符串分割成列表。
text = "Hello, World!"
words = text.split(', ')
print(words)
3. 字符串替换
可以使用replace()
方法替换字符串中的子串。
text = "Hello, World!"
new_text = text.replace("World", "Python")
print(new_text)
4. 字符串查找
可以使用find()
方法查找子串的位置。
text = "Hello, World!"
position = text.find("World")
print(position)
四、字符串处理技巧
1. 去除字符串两端的空白
可以使用strip()
方法去除字符串两端的空白字符。
text = " Hello, World! "
clean_text = text.strip()
print(clean_text)
2. 修改字符串大小写
可以使用lower()
, upper()
, title()
方法修改字符串的大小写。
text = "Hello, World!"
print(text.lower())
print(text.upper())
print(text.title())
3. 字符串判断
可以使用startswith()
, endswith()
, isalpha()
, isdigit()
等方法判断字符串的特性。
text = "Hello, World!"
print(text.startswith("Hello"))
print(text.endswith("World!"))
print(text.isalpha()) # False, because there are non-alphabet characters
print(text.isdigit()) # False, because there are no digit characters
4. 字符串长度
可以使用len()
函数获取字符串的长度。
text = "Hello, World!"
print(len(text))
五、总结
通过上述内容的介绍,已经详细了解了Python中输入字符串和输出字符串的方法。我们可以通过input()
函数从用户获取输入、使用print()
函数输出字符串、利用字符串格式化方法进行输出,还可以从文件读取或输出到文件。此外,还介绍了字符串拼接、分割、替换、查找等常见操作,以及处理字符串时的一些技巧。掌握这些方法和技巧,可以帮助我们更高效地处理字符串输入输出问题,并在实际编程中应用自如。
相关问答FAQs:
如何在Python中输入字符串?
在Python中,可以使用内置的input()
函数来获取用户的输入。这个函数会暂停程序的执行,等待用户输入字符串,直到用户按下回车键。例如,可以使用以下代码来接收用户输入的字符串:
user_input = input("请输入一个字符串:")
如何在Python中输出字符串?
输出字符串可以使用print()
函数。这个函数会将提供的内容显示在控制台上。比如,输出之前接收到的字符串可以用以下代码实现:
print("您输入的字符串是:", user_input)
Python支持哪些字符串操作?
Python提供丰富的字符串操作功能,包括拼接、切片、查找和替换等。拼接字符串可以用+
运算符,切片则使用方括号。例如:
str1 = "Hello"
str2 = "World"
combined = str1 + " " + str2 # 拼接
print(combined) # 输出: Hello World
substring = combined[0:5] # 切片
print(substring) # 输出: Hello
通过这些基本操作,您可以轻松处理和展示字符串。
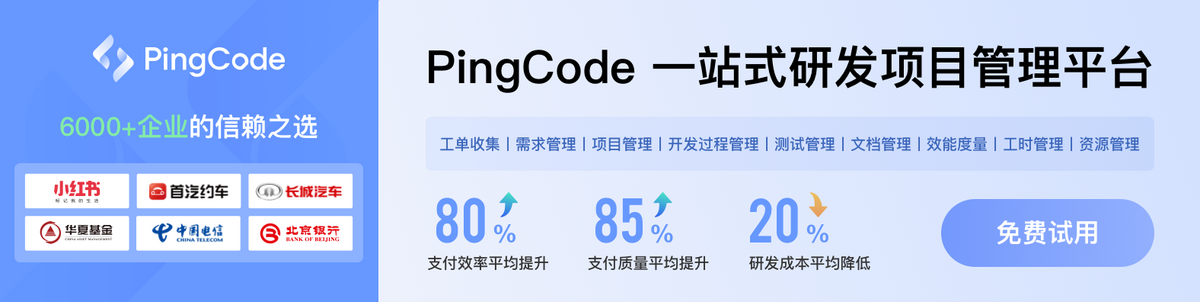