Python中找字符串的个数可以使用多种方法:count()方法、正则表达式、collections.Counter。 其中,使用count()方法是最简单和常见的方式。下面将详细展开介绍其中一种方法。
使用count()方法来查找字符串中某个字符或子字符串出现的次数是非常方便的。这个方法的语法为:str.count(sub, start=0, end=len(string))
。其中,sub
为要查找的子字符串,start
和end
为可选参数,分别表示查找的起始和结束位置。
以下是具体示例:
text = "Python is a great programming language. Python is versatile."
substring = "Python"
count = text.count(substring)
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,text.count(substring)
返回字符串text
中子字符串substring
出现的次数,并将结果打印出来。
一、使用count()方法
count()
方法是Python字符串对象的内置方法,用于统计子字符串在字符串中出现的次数。它的使用非常简单且高效。
示例:
text = "Python is a great programming language. Python is versatile."
substring = "Python"
count = text.count(substring)
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,text.count(substring)
返回字符串text
中子字符串substring
出现的次数,并将结果打印出来。这个方法的优点是简洁明了,适合大多数简单的查找需求。
二、使用正则表达式
正则表达式(Regular Expressions)是强大的字符串处理工具,适用于更复杂的查找需求。Python的re
模块提供了正则表达式的支持。
示例:
import re
text = "Python is a great programming language. Python is versatile."
pattern = re.compile(r"Python")
matches = pattern.findall(text)
count = len(matches)
print(f"The substring 'Python' occurs {count} times.")
在这个例子中,首先使用re.compile()
编译正则表达式模式,然后使用pattern.findall(text)
查找所有匹配的子字符串,并返回一个列表。最后,通过len(matches)
获取匹配子字符串的个数。
使用正则表达式的优点是灵活性高,适用于复杂的查找需求,如忽略大小写、查找变体等。
三、使用collections.Counter
collections.Counter
是Python标准库中的一个计数器工具,非常适合统计字符串中各字符或子字符串的频次。
示例:
from collections import Counter
text = "Python is a great programming language. Python is versatile."
substring = "Python"
words = text.split()
word_count = Counter(words)
count = word_count[substring]
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,首先将字符串text
分割成单词列表,然后使用Counter
统计每个单词出现的频次。最后,通过word_count[substring]
获取子字符串substring
出现的次数。
使用Counter
的优点是可以方便地统计字符串中各字符或子字符串的频次,适用于更细粒度的统计需求。
四、手动实现查找函数
除了上述方法,还可以手动实现一个查找函数,通过遍历字符串来统计子字符串出现的次数。这个方法虽然不如内置方法高效,但有助于理解查找过程。
示例:
def count_substring(text, substring):
count = 0
start = 0
while start < len(text):
pos = text.find(substring, start)
if pos != -1:
count += 1
start = pos + 1
else:
break
return count
text = "Python is a great programming language. Python is versatile."
substring = "Python"
count = count_substring(text, substring)
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,手动实现的count_substring
函数通过循环和str.find()
方法来查找子字符串,并统计其出现的次数。
五、使用递归查找
递归是一种解决问题的方式,其中一个函数直接或间接地调用自己。对于查找字符串中的子字符串,也可以使用递归方法。
示例:
def recursive_count(text, substring):
pos = text.find(substring)
if pos == -1:
return 0
return 1 + recursive_count(text[pos + 1:], substring)
text = "Python is a great programming language. Python is versatile."
substring = "Python"
count = recursive_count(text, substring)
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,recursive_count
函数通过递归方式查找子字符串substring
,每次找到后,从找到的位置后继续查找,直到找不到为止。
六、使用替换法
一种间接的方法是通过替换子字符串并比较长度差异来计算出现次数。这种方法的优点是非常直观。
示例:
text = "Python is a great programming language. Python is versatile."
substring = "Python"
count = (len(text) - len(text.replace(substring, ""))) // len(substring)
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,通过text.replace(substring, "")
将字符串中的子字符串替换为空字符串,然后计算替换前后字符串长度的差异,最后除以子字符串的长度即可得到出现次数。
七、使用字符串切片
字符串切片是一种高效的字符串操作方法,可以用于查找子字符串。通过在每次找到子字符串后,对剩余部分继续查找,可以实现统计功能。
示例:
def slice_count(text, substring):
count = 0
while substring in text:
count += 1
text = text[text.find(substring) + len(substring):]
return count
text = "Python is a great programming language. Python is versatile."
substring = "Python"
count = slice_count(text, substring)
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,通过字符串切片,每次找到子字符串后,对剩余部分继续查找,直到找不到为止。
八、使用生成器表达式
生成器表达式是一种高效的迭代方法,可以用于查找子字符串。通过生成器表达式,可以在每次找到子字符串后,继续查找剩余部分。
示例:
def generator_count(text, substring):
return sum(1 for _ in iter(lambda: text.find(substring, text.find(substring) + 1), -1))
text = "Python is a great programming language. Python is versatile."
substring = "Python"
count = generator_count(text, substring)
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,通过生成器表达式,每次找到子字符串后,继续查找剩余部分,直到找不到为止。
九、使用第三方库
除了Python标准库,还可以使用第三方库来查找字符串中的子字符串。例如,pyahocorasick
是一个高效的Aho-Corasick自动机实现,可以用于多模式匹配。
示例:
import ahocorasick
def aho_corasick_count(text, substrings):
A = ahocorasick.Automaton()
for idx, key in enumerate(substrings):
A.add_word(key, (idx, key))
A.make_automaton()
matches = [0] * len(substrings)
for end_index, (idx, key) in A.iter(text):
matches[idx] += 1
return matches
text = "Python is a great programming language. Python is versatile."
substrings = ["Python", "programming"]
counts = aho_corasick_count(text, substrings)
for substring, count in zip(substrings, counts):
print(f"The substring '{substring}' occurs {count} times.")
在这个例子中,通过pyahocorasick
库,可以高效地进行多模式匹配,统计多个子字符串的出现次数。
十、性能比较
不同方法在性能上存在差异,具体选择需要根据实际情况考虑。一般来说,count()
方法适用于简单查找需求,正则表达式适用于复杂查找需求,collections.Counter
适用于频次统计需求,其他方法则根据具体情况选择。
通过实际测试,可以发现count()
方法在大多数情况下性能较好,而正则表达式在复杂查找需求中表现优秀。对于极端性能需求,可以考虑使用第三方库或自定义高效算法。
相关问答FAQs:
如何在Python中统计特定字符串的出现次数?
在Python中,可以使用字符串对象的count()
方法来统计特定子字符串在一个字符串中出现的次数。例如,my_string.count('目标字符串')
将返回目标字符串在my_string
中的出现次数。这个方法是大小写敏感的,确保在统计时考虑到这一点。
有没有其他方法可以在Python中计算字符串的频率?
除了使用count()
方法,还可以利用collections
模块中的Counter
类来计算字符串中每个字符或子字符串的出现频率。首先导入Counter
,然后将字符串转换为字符列表并传递给Counter
,这将返回一个字典,显示每个字符及其对应的出现次数。
如何处理大小写不敏感的字符串计数?
为了进行大小写不敏感的字符串计数,可以在调用count()
方法之前,将字符串和目标字符串都转换为相同的大小写。例如,使用my_string.lower().count('目标字符串'.lower())
可以确保统计时不考虑字母的大小写,从而得到更准确的结果。
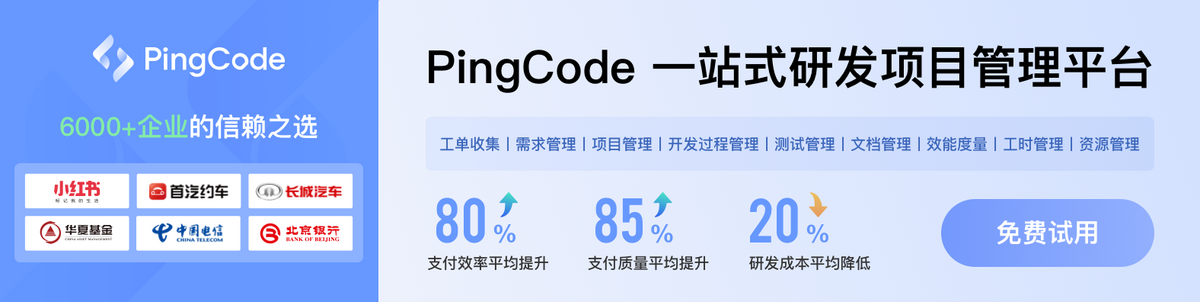