合并两个文档内容的方法有很多种,如使用文件操作、字符串操作、内置库等。其中一种常见的方法是使用Python的文件操作功能,将两个文档的内容读取并写入到一个新的文档中。下面将详细介绍如何使用Python来合并两个文档的内容,并且提供示例代码。
一、文件操作合并文档内容
使用Python内置的文件操作功能,可以方便地读取和写入文档内容。具体步骤如下:
- 打开第一个文档,读取其内容。
- 打开第二个文档,读取其内容。
- 创建一个新的文档,将前两个文档的内容写入新的文档中。
def merge_files(file1, file2, output_file):
with open(file1, 'r', encoding='utf-8') as f1:
content1 = f1.read()
with open(file2, 'r', encoding='utf-8') as f2:
content2 = f2.read()
with open(output_file, 'w', encoding='utf-8') as of:
of.write(content1)
of.write("\n") # 可选:添加换行符
of.write(content2)
示例用法
merge_files('document1.txt', 'document2.txt', 'merged_document.txt')
二、字符串操作合并文档内容
除了文件操作,还可以使用字符串操作来合并文档内容。以下是一个示例,展示如何使用字符串操作来合并两个文档内容:
def merge_files_str(file1, file2, output_file):
with open(file1, 'r', encoding='utf-8') as f1:
content1 = f1.read()
with open(file2, 'r', encoding='utf-8') as f2:
content2 = f2.read()
merged_content = content1 + "\n" + content2
with open(output_file, 'w', encoding='utf-8') as of:
of.write(merged_content)
示例用法
merge_files_str('document1.txt', 'document2.txt', 'merged_document.txt')
三、利用Pandas库合并文档内容
如果处理的是结构化数据,例如CSV文件,可以使用Pandas库来合并文档内容。Pandas提供了强大的数据处理功能,适合处理数据量较大的文档。
import pandas as pd
def merge_csv_files(file1, file2, output_file):
df1 = pd.read_csv(file1)
df2 = pd.read_csv(file2)
merged_df = pd.concat([df1, df2], ignore_index=True)
merged_df.to_csv(output_file, index=False)
示例用法
merge_csv_files('data1.csv', 'data2.csv', 'merged_data.csv')
四、使用pathlib库合并文档内容
Python的pathlib库提供了面向对象的文件系统路径操作方式,结合文件操作可以简化代码的可读性和维护性。
from pathlib import Path
def merge_files_pathlib(file1, file2, output_file):
content1 = Path(file1).read_text(encoding='utf-8')
content2 = Path(file2).read_text(encoding='utf-8')
merged_content = content1 + "\n" + content2
Path(output_file).write_text(merged_content, encoding='utf-8')
示例用法
merge_files_pathlib('document1.txt', 'document2.txt', 'merged_document.txt')
五、处理大文件时的合并方法
如果需要合并的文档非常大,可能无法一次性全部加载到内存中。此时可以逐行读取和写入,确保内存使用率低。
def merge_large_files(file1, file2, output_file):
with open(output_file, 'w', encoding='utf-8') as of:
with open(file1, 'r', encoding='utf-8') as f1:
for line in f1:
of.write(line)
of.write("\n") # 可选:添加换行符
with open(file2, 'r', encoding='utf-8') as f2:
for line in f2:
of.write(line)
示例用法
merge_large_files('large_document1.txt', 'large_document2.txt', 'merged_large_document.txt')
六、处理不同编码格式的文档合并
在处理不同编码格式的文档时,需要确保正确处理编码问题。以下示例展示了如何处理不同编码格式的文档合并:
def merge_files_different_encoding(file1, encoding1, file2, encoding2, output_file, output_encoding):
with open(file1, 'r', encoding=encoding1) as f1:
content1 = f1.read()
with open(file2, 'r', encoding=encoding2) as f2:
content2 = f2.read()
merged_content = content1 + "\n" + content2
with open(output_file, 'w', encoding=output_encoding) as of:
of.write(merged_content)
示例用法
merge_files_different_encoding('document1_utf8.txt', 'utf-8', 'document2_iso.txt', 'ISO-8859-1', 'merged_document.txt', 'utf-8')
七、合并多个文档内容
如果需要合并多个文档内容,可以通过循环来实现。以下示例展示了如何合并多个文档内容:
def merge_multiple_files(file_list, output_file):
with open(output_file, 'w', encoding='utf-8') as of:
for file in file_list:
with open(file, 'r', encoding='utf-8') as f:
of.write(f.read())
of.write("\n") # 可选:添加换行符
示例用法
files_to_merge = ['document1.txt', 'document2.txt', 'document3.txt']
merge_multiple_files(files_to_merge, 'merged_document.txt')
八、合并文档内容并添加标记
有时我们需要在合并的文档中添加标记,以便于区分不同来源的内容。以下示例展示了如何添加标记:
def merge_files_with_labels(file1, label1, file2, label2, output_file):
with open(file1, 'r', encoding='utf-8') as f1:
content1 = f"{label1}\n" + f1.read()
with open(file2, 'r', encoding='utf-8') as f2:
content2 = f"{label2}\n" + f2.read()
merged_content = content1 + "\n" + content2
with open(output_file, 'w', encoding='utf-8') as of:
of.write(merged_content)
示例用法
merge_files_with_labels('document1.txt', 'Document 1:', 'document2.txt', 'Document 2:', 'merged_document_with_labels.txt')
九、合并特定内容的文档
在某些情况下,只需要合并文档中的特定内容,例如包含某些关键词的段落。以下示例展示了如何实现:
def merge_files_with_keywords(file1, keyword1, file2, keyword2, output_file):
def extract_content_with_keyword(file, keyword):
content = ""
with open(file, 'r', encoding='utf-8') as f:
for line in f:
if keyword in line:
content += line
return content
content1 = extract_content_with_keyword(file1, keyword1)
content2 = extract_content_with_keyword(file2, keyword2)
merged_content = content1 + "\n" + content2
with open(output_file, 'w', encoding='utf-8') as of:
of.write(merged_content)
示例用法
merge_files_with_keywords('document1.txt', 'important', 'document2.txt', 'critical', 'merged_document_with_keywords.txt')
十、合并文档内容并按顺序排列
有时需要将合并的文档内容按特定顺序排列,例如按字母顺序排列。以下示例展示了如何实现:
def merge_files_with_sorting(file1, file2, output_file):
with open(file1, 'r', encoding='utf-8') as f1:
content1 = f1.readlines()
with open(file2, 'r', encoding='utf-8') as f2:
content2 = f2.readlines()
merged_content = content1 + content2
merged_content.sort()
with open(output_file, 'w', encoding='utf-8') as of:
of.writelines(merged_content)
示例用法
merge_files_with_sorting('document1.txt', 'document2.txt', 'merged_sorted_document.txt')
通过上述方法,我们可以根据具体需求选择合适的方式来合并两个或多个文档的内容。每种方法都有其适用场景和优缺点,根据实际情况进行选择,可以实现高效、便捷的文档内容合并操作。
相关问答FAQs:
如何使用Python合并两个文本文件的内容?
要合并两个文本文件,可以使用Python的内置文件操作功能。首先,打开两个文件,读取它们的内容,并将内容写入到一个新的文件中。以下是一个简单的示例代码:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2, open('merged.txt', 'w') as merged_file:
merged_file.write(file1.read())
merged_file.write('\n') # 添加换行符
merged_file.write(file2.read())
这种方法确保了两个文件的内容被顺利合并。
合并文档时如何处理不同格式的文件?
如果需要合并不同格式的文件,例如一个是文本文件,另一个是Word文档,可以使用Python的第三方库,例如python-docx
来处理Word文件。读取Word文档的内容后,将其转换为文本并合并。示例代码如下:
from docx import Document
def read_word_file(file_path):
doc = Document(file_path)
return '\n'.join([para.text for para in doc.paragraphs])
text_content = ''
with open('file1.txt', 'r') as file1:
text_content += file1.read() + '\n'
text_content += read_word_file('file2.docx')
with open('merged.txt', 'w') as merged_file:
merged_file.write(text_content)
这样,您可以轻松合并不同格式的文档。
合并多个文档时,有哪些最佳实践?
在合并多个文档时,遵循一些最佳实践可以帮助提高效率和可读性。首先,确保所有文档的编码格式一致,避免字符编码问题。其次,添加适当的分隔符,以便在合并后,能清晰地辨别各个文档的内容。最后,保留合并文档的原始格式,尤其是在处理格式化文档时,可以使用相关库来保持格式一致性。这样做不仅可以提高合并后的文档质量,还能提升后续处理的便利性。
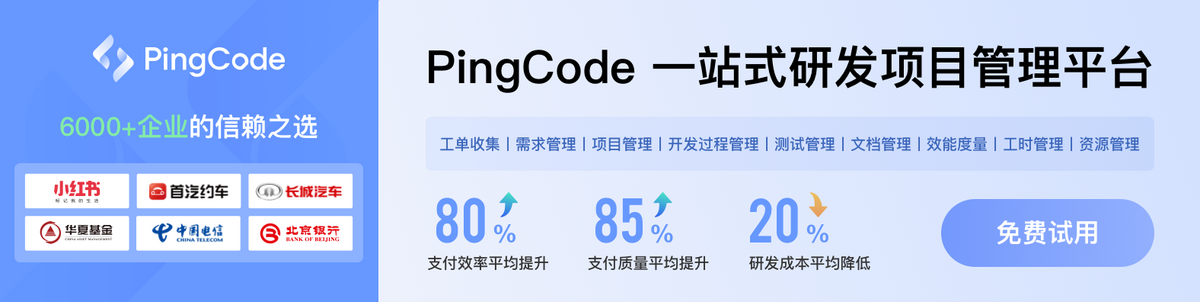