开头段落:
要用Python写一个QQ聊天机器人,可以使用Python库进行网络连接、消息处理和自动回复、用户管理、集成第三方API。其中,网络连接是关键,因为它决定了你的机器人能否顺利连接到QQ服务器并接收和发送消息。通过使用现有的QQ协议库,可以简化这个过程,快速实现一个基本的QQ聊天机器人。
一、网络连接
要实现QQ聊天机器人,首先需要解决的是网络连接问题。QQ协议并不是公开的,但有一些第三方库可以帮助我们完成这些工作。其中,最常见的是使用CoolQ
或CQHttp
等第三方库,这些库提供了与QQ服务器通信的接口。
1.1 使用CoolQ
CoolQ是一个QQ机器人框架,支持各种插件,通过HTTP API与其他程序通信。首先,我们需要安装CoolQ和相关插件,然后用Python通过HTTP API与CoolQ通信。
import requests
def send_message(group_id, message):
url = 'http://127.0.0.1:5700/send_group_msg'
payload = {
'group_id': group_id,
'message': message
}
response = requests.post(url, json=payload)
return response.json()
group_id = 123456789
message = 'Hello, this is a test message!'
send_message(group_id, message)
1.2 使用CQHttp
CQHttp是CoolQ的一个HTTP插件,它通过HTTP接口和其他程序通信。使用CQHttp可以更灵活地与QQ进行通信。
import requests
def send_private_msg(user_id, message):
url = 'http://127.0.0.1:5700/send_private_msg'
payload = {
'user_id': user_id,
'message': message
}
response = requests.post(url, json=payload)
return response.json()
user_id = 123456789
message = 'Hello, this is a test message!'
send_private_msg(user_id, message)
二、消息处理和自动回复
在连接成功后,我们需要处理接收到的消息并进行自动回复。可以通过设置消息处理函数来实现这一点。
2.1 消息处理函数
我们可以通过编写一个消息处理函数来处理接收到的消息,并根据消息内容进行相应的回复。
def handle_message(event):
message = event['message']
user_id = event['user_id']
if 'hello' in message.lower():
reply = 'Hello! How can I help you today?'
elif 'bye' in message.lower():
reply = 'Goodbye! Have a nice day!'
else:
reply = 'Sorry, I did not understand that.'
send_private_msg(user_id, reply)
2.2 注册消息处理函数
我们需要将消息处理函数注册到消息接收事件中,以便在接收到消息时调用该函数。
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def webhook():
event = request.json
handle_message(event)
return jsonify({'status': 'ok'})
if __name__ == '__main__':
app.run(port=5700)
三、用户管理
为了提高聊天机器人的实用性,可以添加用户管理功能,例如记录用户信息、管理用户权限等。
3.1 用户信息记录
可以使用数据库或文件系统来记录用户信息,并在接收到消息时进行更新。
import sqlite3
def create_user_table():
conn = sqlite3.connect('users.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS users
(user_id INTEGER PRIMARY KEY, username TEXT, last_message TEXT)''')
conn.commit()
conn.close()
def add_user(user_id, username):
conn = sqlite3.connect('users.db')
c = conn.cursor()
c.execute('INSERT INTO users (user_id, username) VALUES (?, ?)', (user_id, username))
conn.commit()
conn.close()
def update_last_message(user_id, last_message):
conn = sqlite3.connect('users.db')
c = conn.cursor()
c.execute('UPDATE users SET last_message = ? WHERE user_id = ?', (last_message, user_id))
conn.commit()
conn.close()
3.2 用户权限管理
可以根据用户的权限级别来决定是否允许用户执行某些操作。
def check_permission(user_id):
# 假设我们有一个权限表,存储用户的权限级别
conn = sqlite3.connect('users.db')
c = conn.cursor()
c.execute('SELECT permission_level FROM users WHERE user_id = ?', (user_id,))
result = c.fetchone()
conn.close()
if result:
permission_level = result[0]
return permission_level >= 1 # 假设1是普通用户的权限级别
else:
return False
def handle_message(event):
message = event['message']
user_id = event['user_id']
if not check_permission(user_id):
reply = 'Sorry, you do not have permission to use this command.'
elif 'hello' in message.lower():
reply = 'Hello! How can I help you today?'
elif 'bye' in message.lower():
reply = 'Goodbye! Have a nice day!'
else:
reply = 'Sorry, I did not understand that.'
send_private_msg(user_id, reply)
四、集成第三方API
为了增强聊天机器人的功能,可以集成第三方API,例如天气查询、翻译服务等。
4.1 天气查询
可以使用天气API来查询天气信息,并将结果发送给用户。
import requests
def get_weather(city):
api_key = 'your_api_key'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
data = response.json()
if data['cod'] == 200:
weather = data['weather'][0]['description']
temperature = data['main']['temp'] - 273.15 # 转换为摄氏度
return f'The weather in {city} is {weather} with a temperature of {temperature:.2f}°C.'
else:
return 'Sorry, I could not find the weather information for that city.'
def handle_message(event):
message = event['message']
user_id = event['user_id']
if 'weather' in message.lower():
city = message.split(' ')[-1]
reply = get_weather(city)
elif 'hello' in message.lower():
reply = 'Hello! How can I help you today?'
elif 'bye' in message.lower():
reply = 'Goodbye! Have a nice day!'
else:
reply = 'Sorry, I did not understand that.'
send_private_msg(user_id, reply)
4.2 翻译服务
可以使用翻译API来提供翻译服务,并将翻译结果发送给用户。
from googletrans import Translator
def translate_text(text, dest_language):
translator = Translator()
translation = translator.translate(text, dest=dest_language)
return translation.text
def handle_message(event):
message = event['message']
user_id = event['user_id']
if 'translate' in message.lower():
parts = message.split(' ')
text = ' '.join(parts[1:-1])
dest_language = parts[-1]
translation = translate_text(text, dest_language)
reply = f'Translation: {translation}'
elif 'hello' in message.lower():
reply = 'Hello! How can I help you today?'
elif 'bye' in message.lower():
reply = 'Goodbye! Have a nice day!'
else:
reply = 'Sorry, I did not understand that.'
send_private_msg(user_id, reply)
通过以上步骤,我们可以用Python实现一个功能齐全的QQ聊天机器人。这个机器人不仅可以发送和接收消息,还可以处理用户请求、管理用户信息,并集成第三方API提供更多功能。希望这些内容能帮助你实现自己的QQ聊天机器人项目。
相关问答FAQs:
如何用Python实现QQ的基本功能?
在Python中实现QQ的基本功能需要考虑到多个方面,例如用户注册、登录、消息发送和接收等。可以使用Flask或Django等框架搭建服务器端,利用Socket编程实现实时消息传递。同时,使用数据库(如SQLite或MySQL)存储用户信息和聊天记录。前端可以使用Tkinter或PyQt创建用户界面。
我需要哪些库或模块来开发QQ聊天应用?
开发QQ聊天应用时,可以考虑使用以下库:socket
用于网络通信,threading
实现多线程处理,Flask
或Django
构建后端服务,SQLAlchemy
或PyMongo
进行数据库操作。此外,Tkinter
或PyQt
可以帮助创建图形用户界面,提升用户体验。
如何保证QQ应用的安全性和隐私保护?
在开发QQ应用时,安全性和隐私保护至关重要。可以通过加密协议(如SSL/TLS)确保数据传输的安全性,使用哈希算法对用户密码进行加密存储。此外,定期进行安全审计和漏洞检测,采用权限控制策略以限制用户访问敏感信息,都是增强应用安全性的有效方法。
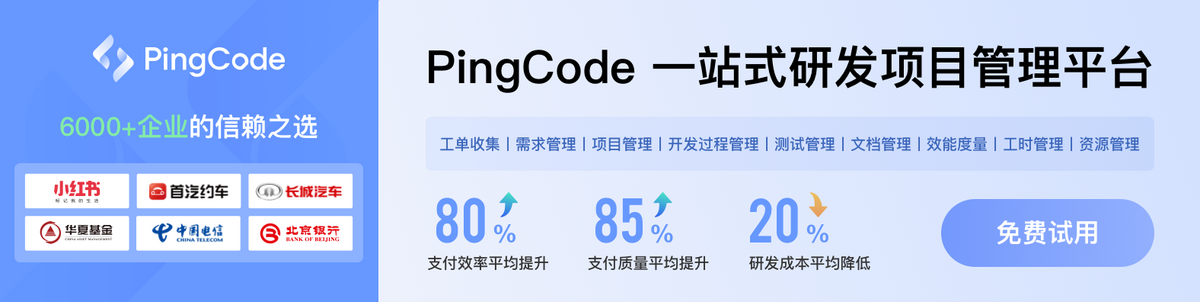