Python中可以使用逻辑运算符 and
和 or
来编写包含多个条件的语句,and
用于要求所有条件都为真,而 or
则用于只要有一个条件为真即可。 例如,判断一个数字是否在10到20之间可以使用 if 10 < number < 20:
,要判断一个数是否在10到20之间或者在30到40之间,可以使用 if (10 < number < 20) or (30 < number < 40):
。下面详细介绍如何在Python中使用这两个条件。
一、使用 and
逻辑运算符
逻辑运算符 and
表示“并且”,即要求所有条件都为真时,整个表达式才为真。
1、基本用法
number = 15
if number > 10 and number < 20:
print("Number is between 10 and 20")
else:
print("Number is not between 10 and 20")
在这个例子中,if
语句中的 number > 10 and number < 20
表示,只有当 number
同时大于10并且小于20时,才会执行 print("Number is between 10 and 20")
。
2、多条件判断
a = 5
b = 10
c = 15
if a < b and b < c:
print("a is less than b and b is less than c")
else:
print("Condition not met")
这里,我们有三个变量 a
, b
, c
,并使用 and
运算符来判断 a
是否小于 b
并且 b
是否小于 c
。
二、使用 or
逻辑运算符
逻辑运算符 or
表示“或”,即只要有一个条件为真,整个表达式就为真。
1、基本用法
number = 25
if number < 10 or number > 20:
print("Number is either less than 10 or greater than 20")
else:
print("Number is between 10 and 20")
在这个例子中,if
语句中的 number < 10 or number > 20
表示,只要 number
小于10或大于20,都会执行 print("Number is either less than 10 or greater than 20")
。
2、多条件判断
x = 5
y = 20
if x < 10 or y > 15:
print("Either x is less than 10 or y is greater than 15")
else:
print("Condition not met")
在这个例子中,我们使用了 or
运算符来判断 x
是否小于10或者 y
是否大于15,只要其中一个条件为真,条件就成立。
三、结合使用 and
和 or
有时候,我们需要结合使用 and
和 or
运算符来判断更复杂的条件。
a = 5
b = 15
c = 25
if (a < 10 and b > 10) or c == 25:
print("Condition met")
else:
print("Condition not met")
在这个例子中,if
语句中的条件 (a < 10 and b > 10) or c == 25
表示,只要 (a < 10 and b > 10)
为真,或者 c == 25
为真,条件就成立。
四、实际应用案例
1、判断用户输入
用户输入通常需要多个条件进行判断。例如,检查用户输入的年龄是否在某个范围内,并且是否具有特定的资格。
age = int(input("Enter your age: "))
qualification = input("Enter your qualification: ")
if (age > 18 and age < 35) and (qualification == "Graduate" or qualification == "Postgraduate"):
print("You are eligible")
else:
print("You are not eligible")
在这个例子中,我们结合使用 and
和 or
来判断用户的年龄和资格。
2、处理多个条件的复杂逻辑
在实际应用中,特别是数据处理和分析中,经常需要根据多个条件来处理数据。例如,筛选出符合特定条件的记录。
data = [
{"name": "John", "age": 28, "city": "New York"},
{"name": "Anna", "age": 22, "city": "London"},
{"name": "Mike", "age": 32, "city": "San Francisco"},
]
filtered_data = [person for person in data if (person["age"] > 25 and person["city"] == "New York") or person["age"] < 30]
print(filtered_data)
在这个例子中,我们有一个包含多个字典的列表,使用列表解析和逻辑运算符筛选出符合条件的记录。
五、注意事项
1、运算符优先级
需要注意的是,and
的优先级高于 or
,这意味着在没有使用括号的情况下,and
会先计算。例如:
if True or False and False:
print("Condition met")
else:
print("Condition not met")
这段代码会输出 Condition met
,因为 False and False
先计算,结果为 False
,然后 True or False
结果为 True
。
2、使用括号提高可读性
为了避免运算符优先级带来的困扰,建议在复杂条件判断中使用括号来提高代码的可读性和正确性。
if (True or False) and False:
print("Condition met")
else:
print("Condition not met")
这段代码会输出 Condition not met
,因为 (True or False)
结果为 True
,然后 True and False
结果为 False
。
六、总结
在Python中,使用 and
和 or
逻辑运算符可以方便地对多个条件进行判断。and
用于所有条件都必须为真,or
用于只要一个条件为真即可。结合使用 and
和 or
可以处理更复杂的逻辑,括号的使用可以提高代码的可读性和正确性。无论是在简单的条件判断还是在复杂的数据处理中,这些逻辑运算符都是不可或缺的工具。
相关问答FAQs:
如何在Python中使用多个条件进行判断?
在Python中,可以使用逻辑运算符如and
和or
来连接多个条件。使用and
时,只有当所有条件都为真时,整体条件才为真;而使用or
时,只要有一个条件为真,整体条件就为真。例如:
if condition1 and condition2:
# 当两个条件均为真时执行的代码
elif condition1 or condition2:
# 当至少一个条件为真时执行的代码
在Python中如何使用嵌套条件?
嵌套条件指的是在一个条件语句内部再使用另一个条件语句。这对于处理复杂的条件逻辑非常有用。例如:
if condition1:
if condition2:
# condition1 和 condition2 都为真时执行的代码
else:
# condition1 为真,但 condition2 为假时执行的代码
else:
# condition1 为假时执行的代码
这种结构可以使代码更清晰,便于阅读和维护。
在Python中如何处理多个条件的优先级?
在Python中,逻辑运算符的优先级决定了多个条件的执行顺序。not
的优先级最高,其次是 and
,最后是 or
。例如,condition1 or condition2 and condition3
会先计算 condition2 and condition3
,再与 condition1
进行或运算。如果想要改变这种优先级,可以使用括号来明确顺序,例如:
if condition1 or (condition2 and condition3):
# 根据优先级执行的代码
使用括号不仅能改变条件的计算顺序,还能提高代码的可读性。
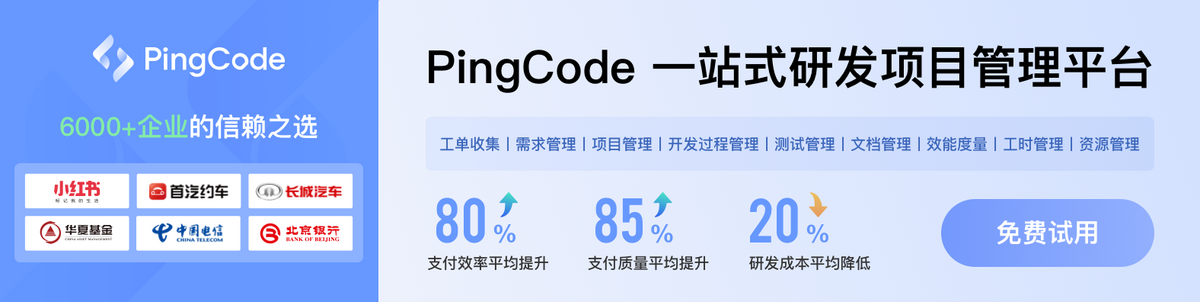