要使用Python调数据库语句,您需要安装和使用适合的数据库连接库、编写SQL查询语句、使用游标执行查询、处理结果。 例如,您可以使用sqlite3
库连接SQLite数据库、执行SQL查询并处理结果。接下来将详细介绍如何使用Python进行数据库操作。
一、安装和导入所需库
要与数据库进行交互,首先需要安装并导入相应的数据库连接库。以下是常用的几种数据库连接库及其安装方法:
1. SQLite
SQLite是一个轻量级的嵌入式数据库,Python内置支持,可以直接使用sqlite3
模块。
import sqlite3
2. MySQL
要连接MySQL数据库,需要安装mysql-connector-python
库。
pip install mysql-connector-python
导入库:
import mysql.connector
3. PostgreSQL
要连接PostgreSQL数据库,需要安装psycopg2
库。
pip install psycopg2
导入库:
import psycopg2
二、连接到数据库
不同的数据库有不同的连接方法,以下分别介绍如何连接到SQLite、MySQL和PostgreSQL数据库。
1. 连接到SQLite数据库
conn = sqlite3.connect('example.db')
2. 连接到MySQL数据库
conn = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
3. 连接到PostgreSQL数据库
conn = psycopg2.connect(
host="localhost",
database="yourdatabase",
user="yourusername",
password="yourpassword"
)
三、创建游标并执行SQL查询
一旦连接到数据库,就可以创建游标对象并执行SQL查询语句。
1. SQLite示例
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS students (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
插入数据
cursor.execute("INSERT INTO students (name, age) VALUES (?, ?)", ('John Doe', 20))
查询数据
cursor.execute("SELECT * FROM students")
rows = cursor.fetchall()
for row in rows:
print(row)
2. MySQL示例
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS students (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), age INT)''')
插入数据
cursor.execute("INSERT INTO students (name, age) VALUES (%s, %s)", ('John Doe', 20))
查询数据
cursor.execute("SELECT * FROM students")
rows = cursor.fetchall()
for row in rows:
print(row)
3. PostgreSQL示例
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS students (id SERIAL PRIMARY KEY, name VARCHAR(255), age INTEGER)''')
插入数据
cursor.execute("INSERT INTO students (name, age) VALUES (%s, %s)", ('John Doe', 20))
查询数据
cursor.execute("SELECT * FROM students")
rows = cursor.fetchall()
for row in rows:
print(row)
四、处理查询结果
执行SQL查询后,通常会返回查询结果。可以使用游标对象的各种方法来处理这些结果。
1. fetchall()
fetchall()
方法返回查询结果的所有行。
rows = cursor.fetchall()
for row in rows:
print(row)
2. fetchone()
fetchone()
方法返回查询结果的下一行。
row = cursor.fetchone()
while row:
print(row)
row = cursor.fetchone()
3. fetchmany()
fetchmany(size)
方法返回查询结果的指定数量行。
rows = cursor.fetchmany(5)
for row in rows:
print(row)
五、提交事务和关闭连接
数据库操作完成后,记得提交事务和关闭连接。
1. 提交事务
对于支持事务的数据库(如MySQL、PostgreSQL),需要显式提交事务。
conn.commit()
2. 关闭游标和连接
操作完成后,关闭游标和连接以释放资源。
cursor.close()
conn.close()
六、完整示例
以下是一个完整的示例,展示如何使用Python连接SQLite数据库、执行SQL查询并处理结果。
import sqlite3
连接到SQLite数据库
conn = sqlite3.connect('example.db')
创建游标
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS students (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
插入数据
cursor.execute("INSERT INTO students (name, age) VALUES (?, ?)", ('John Doe', 20))
cursor.execute("INSERT INTO students (name, age) VALUES (?, ?)", ('Jane Smith', 22))
查询数据
cursor.execute("SELECT * FROM students")
rows = cursor.fetchall()
for row in rows:
print(row)
提交事务
conn.commit()
关闭游标和连接
cursor.close()
conn.close()
七、处理异常
在实际应用中,数据库操作可能会遇到各种异常,建议使用try-except
块进行异常处理。
import sqlite3
try:
# 连接到SQLite数据库
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# 执行数据库操作
cursor.execute('''CREATE TABLE IF NOT EXISTS students (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
cursor.execute("INSERT INTO students (name, age) VALUES (?, ?)", ('John Doe', 20))
cursor.execute("SELECT * FROM students")
rows = cursor.fetchall()
for row in rows:
print(row)
# 提交事务
conn.commit()
except sqlite3.Error as e:
print(f"An error occurred: {e}")
finally:
if cursor:
cursor.close()
if conn:
conn.close()
八、使用ORM(对象关系映射)
除了直接使用SQL语句操作数据库,还可以使用ORM(对象关系映射)库,如SQLAlchemy,以更高效、更优雅地进行数据库操作。
安装SQLAlchemy
pip install sqlalchemy
使用SQLAlchemy
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
创建引擎
engine = create_engine('sqlite:///example.db')
创建基类
Base = declarative_base()
定义模型
class Student(Base):
__tablename__ = 'students'
id = Column(Integer, primary_key=True, autoincrement=True)
name = Column(String)
age = Column(Integer)
创建表
Base.metadata.create_all(engine)
创建会话
Session = sessionmaker(bind=engine)
session = Session()
插入数据
new_student = Student(name='John Doe', age=20)
session.add(new_student)
session.commit()
查询数据
students = session.query(Student).all()
for student in students:
print(student.name, student.age)
关闭会话
session.close()
结论
通过本文的讲解,您应了解了如何使用Python调数据库语句。无论是直接使用数据库连接库,还是使用ORM库如SQLAlchemy,Python都提供了强大的工具来帮助您高效地进行数据库操作。希望这些内容对您有所帮助,并能在实际项目中灵活应用。
相关问答FAQs:
如何在Python中连接到数据库?
在Python中,可以使用多种库来连接数据库,如sqlite3
、MySQL Connector
、psycopg2
等。首先,确保你安装了所需的数据库驱动。然后,使用相应的连接函数,例如sqlite3.connect('database.db')
或mysql.connector.connect(host='localhost', user='yourusername', password='yourpassword', database='yourdatabase')
,来建立连接。
Python中如何执行SQL查询?
在建立数据库连接后,可以使用游标(cursor)对象来执行SQL查询。使用cursor.execute("YOUR SQL QUERY")
来执行查询,接着可以使用fetchall()
或fetchone()
方法来获取查询结果。例如,执行SELECT * FROM your_table
后,通过results = cursor.fetchall()
获取所有结果。
如何处理Python数据库中的异常?
在执行数据库操作时,处理异常是非常重要的。可以使用try...except
语句来捕获可能发生的错误。比如,在执行SQL语句时,如果出现连接失败或语法错误,可以捕获sqlite3.Error
或相应的数据库错误,并进行相应处理,比如记录日志或返回用户友好的错误信息。
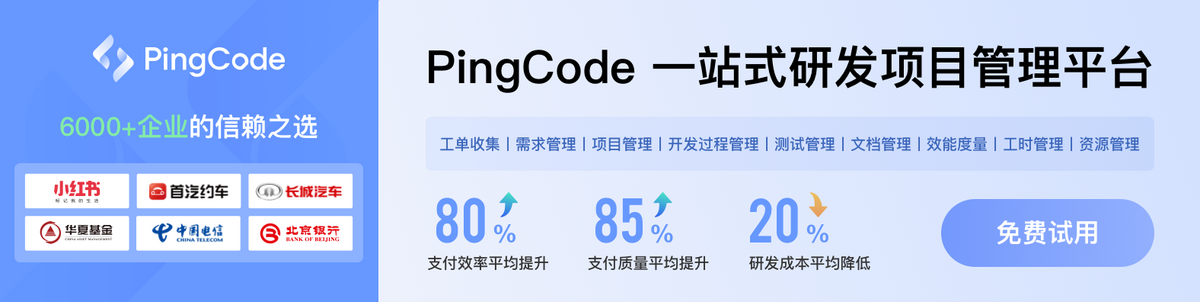