在Python中,有多种方法可以查看有多少个函数。可以使用内置模块、第三方库,甚至编写自定义代码。以下是一些常用的方法:使用inspect模块、使用dir()函数、使用ast模块。其中,使用inspect模块是最详细和灵活的方法。
使用inspect模块
Python的inspect模块提供了多种函数来获取有关对象的信息。我们可以使用它来获取一个模块中的所有函数。以下是一个示例代码:
import inspect
import module_name
def get_functions(module):
return [name for name, obj in inspect.getmembers(module) if inspect.isfunction(obj)]
functions = get_functions(module_name)
print(f"Number of functions: {len(functions)}")
print(f"Functions: {functions}")
在这个示例中,我们首先导入inspect模块和目标模块。然后,我们定义了一个名为get_functions的函数,该函数获取模块中所有函数的名称。最后,我们打印出函数的数量和名称。
使用dir()函数
dir()函数返回指定对象的属性和方法列表。我们可以使用它来获取模块中的所有属性和方法,然后筛选出函数。以下是一个示例代码:
import module_name
def get_functions(module):
return [attr for attr in dir(module) if callable(getattr(module, attr))]
functions = get_functions(module_name)
print(f"Number of functions: {len(functions)}")
print(f"Functions: {functions}")
在这个示例中,我们首先导入目标模块。然后,我们定义了一个名为get_functions的函数,该函数获取模块中所有可调用的属性。最后,我们打印出函数的数量和名称。
使用ast模块
ast模块用于处理抽象语法树。我们可以使用它来解析Python源代码并提取函数定义。以下是一个示例代码:
import ast
def get_functions_from_code(code):
tree = ast.parse(code)
return [node.name for node in ast.walk(tree) if isinstance(node, ast.FunctionDef)]
with open("module_name.py", "r") as file:
code = file.read()
functions = get_functions_from_code(code)
print(f"Number of functions: {len(functions)}")
print(f"Functions: {functions}")
在这个示例中,我们首先导入ast模块。然后,我们定义了一个名为get_functions_from_code的函数,该函数解析Python源代码并提取函数定义。最后,我们读取目标模块的源代码,并打印出函数的数量和名称。
一、使用inspect模块
inspect模块提供了多种函数来获取有关对象的信息。它可以用于获取模块中的所有函数、类和方法。以下是详细的介绍:
1、获取模块中的所有函数
我们可以使用inspect.getmembers()函数来获取模块中的所有成员,然后使用inspect.isfunction()函数筛选出函数。以下是示例代码:
import inspect
import module_name
def get_functions(module):
return [name for name, obj in inspect.getmembers(module) if inspect.isfunction(obj)]
functions = get_functions(module_name)
print(f"Number of functions: {len(functions)}")
print(f"Functions: {functions}")
在这个示例中,inspect.getmembers()函数返回模块中的所有成员,包括函数、类和变量。我们使用列表推导式和inspect.isfunction()函数筛选出函数。
2、获取类中的所有方法
我们可以使用类似的方法来获取类中的所有方法。以下是示例代码:
import inspect
import module_name
def get_methods(cls):
return [name for name, obj in inspect.getmembers(cls) if inspect.isfunction(obj)]
methods = get_methods(module_name.ClassName)
print(f"Number of methods: {len(methods)}")
print(f"Methods: {methods}")
在这个示例中,我们使用inspect.getmembers()函数获取类中的所有成员,然后使用inspect.isfunction()函数筛选出方法。
3、获取对象的所有属性和方法
我们还可以使用inspect模块来获取对象的所有属性和方法。以下是示例代码:
import inspect
def get_attributes_and_methods(obj):
return [name for name, obj in inspect.getmembers(obj) if not name.startswith("__")]
obj = module_name.ClassName()
attributes_and_methods = get_attributes_and_methods(obj)
print(f"Attributes and methods: {attributes_and_methods}")
在这个示例中,我们使用inspect.getmembers()函数获取对象的所有成员,并排除以双下划线开头的特殊属性和方法。
二、使用dir()函数
dir()函数返回指定对象的属性和方法列表。我们可以使用它来获取模块中的所有属性和方法,然后筛选出函数。以下是详细的介绍:
1、获取模块中的所有函数
我们可以使用dir()函数获取模块中的所有属性和方法,然后使用callable()函数筛选出函数。以下是示例代码:
import module_name
def get_functions(module):
return [attr for attr in dir(module) if callable(getattr(module, attr))]
functions = get_functions(module_name)
print(f"Number of functions: {len(functions)}")
print(f"Functions: {functions}")
在这个示例中,dir()函数返回模块中的所有属性和方法。我们使用列表推导式和callable()函数筛选出函数。
2、获取类中的所有方法
我们可以使用类似的方法来获取类中的所有方法。以下是示例代码:
import module_name
def get_methods(cls):
return [attr for attr in dir(cls) if callable(getattr(cls, attr))]
methods = get_methods(module_name.ClassName)
print(f"Number of methods: {len(methods)}")
print(f"Methods: {methods}")
在这个示例中,dir()函数返回类中的所有属性和方法。我们使用列表推导式和callable()函数筛选出方法。
3、获取对象的所有属性和方法
我们还可以使用dir()函数来获取对象的所有属性和方法。以下是示例代码:
import module_name
def get_attributes_and_methods(obj):
return [attr for attr in dir(obj) if not attr.startswith("__")]
obj = module_name.ClassName()
attributes_and_methods = get_attributes_and_methods(obj)
print(f"Attributes and methods: {attributes_and_methods}")
在这个示例中,dir()函数返回对象的所有属性和方法。我们使用列表推导式并排除以双下划线开头的特殊属性和方法。
三、使用ast模块
ast模块用于处理抽象语法树。我们可以使用它来解析Python源代码并提取函数定义。以下是详细的介绍:
1、解析Python源代码
我们可以使用ast.parse()函数来解析Python源代码,并生成抽象语法树。以下是示例代码:
import ast
with open("module_name.py", "r") as file:
code = file.read()
tree = ast.parse(code)
在这个示例中,我们使用ast.parse()函数解析目标模块的源代码,并生成抽象语法树。
2、提取函数定义
我们可以使用ast.walk()函数遍历抽象语法树,并提取函数定义。以下是示例代码:
import ast
def get_functions_from_code(code):
tree = ast.parse(code)
return [node.name for node in ast.walk(tree) if isinstance(node, ast.FunctionDef)]
with open("module_name.py", "r") as file:
code = file.read()
functions = get_functions_from_code(code)
print(f"Number of functions: {len(functions)}")
print(f"Functions: {functions}")
在这个示例中,ast.walk()函数遍历抽象语法树,并返回所有节点。我们使用列表推导式和isinstance()函数筛选出函数定义节点。
3、提取类中的方法定义
我们可以使用类似的方法来提取类中的方法定义。以下是示例代码:
import ast
def get_methods_from_code(code):
tree = ast.parse(code)
methods = []
for node in ast.walk(tree):
if isinstance(node, ast.ClassDef):
for sub_node in node.body:
if isinstance(sub_node, ast.FunctionDef):
methods.append(sub_node.name)
return methods
with open("module_name.py", "r") as file:
code = file.read()
methods = get_methods_from_code(code)
print(f"Number of methods: {len(methods)}")
print(f"Methods: {methods}")
在这个示例中,我们遍历抽象语法树,并提取类定义节点。然后,我们遍历类定义节点的子节点,并筛选出方法定义节点。
四、总结
在Python中,有多种方法可以查看有多少个函数。使用inspect模块是最详细和灵活的方法,它可以获取模块中的所有函数、类和方法。使用dir()函数可以快速获取模块中的所有属性和方法,并筛选出函数。使用ast模块可以解析Python源代码,并提取函数和方法定义。无论使用哪种方法,都可以根据需要选择合适的工具来查看函数数量。
相关问答FAQs:
如何查看Python代码中定义的所有函数?
在Python中,可以使用内置的dir()
和inspect
模块来查看当前模块或对象中定义的所有函数。首先,导入inspect
模块,然后使用inspect.getmembers()
函数,结合inspect.isfunction()
来筛选出所有的函数。例如:
import inspect
def sample_function():
pass
class SampleClass:
def method_one(self):
pass
def method_two(self):
pass
# 查看当前模块的所有函数
functions = inspect.getmembers(SampleClass, inspect.isfunction)
print(functions)
是否可以通过IDLE或其他IDE查看函数数量?
许多集成开发环境(IDE),如PyCharm、VSCode等,提供了代码导航功能,可以轻松查看当前文件或整个项目中定义的函数。通常,IDE的侧边栏会有一个“结构”或“大纲”视图,列出所有函数及其定义位置。使用这些工具,您可以快速了解代码结构和函数数量。
对于大型项目,如何高效统计所有函数?
在大型项目中,可以使用脚本自动化统计函数数量。通过遍历项目中的所有Python文件,结合ast
模块(抽象语法树)来分析代码结构,可以有效获取每个文件中的函数定义。例如:
import ast
import os
def count_functions_in_file(filepath):
with open(filepath, 'r') as file:
node = ast.parse(file.read())
return len([n for n in ast.walk(node) if isinstance(n, ast.FunctionDef)])
def count_functions_in_directory(directory):
total_functions = 0
for filename in os.listdir(directory):
if filename.endswith('.py'):
total_functions += count_functions_in_file(os.path.join(directory, filename))
return total_functions
# 调用函数并传入项目目录
print(count_functions_in_directory('/path/to/your/project'))
通过这种方式,您可以快速获取整个项目中所有函数的数量,节省了手动检查的时间。
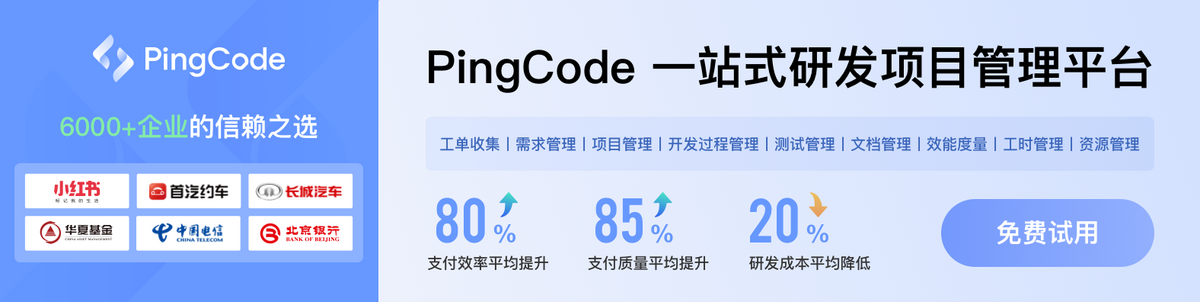