要用Python编写从1加到1000的程序,我们可以使用多种方法,其中最简单和直接的方式是使用一个循环结构。使用for循环、使用while循环、使用数学公式是三种常见的方法。我们可以详细讲解其中一种方法。
使用for循环是一种非常直观和常用的方法。通过遍历从1到1000的所有数字,将它们依次累加,最终得到结果。下面我们具体讲解这种方法。
一、使用for循环
total = 0
for i in range(1, 1001):
total += i
print("The sum from 1 to 1000 is:", total)
在这个示例中,我们定义了一个变量total
来存储累加结果,并使用for
循环遍历从1到1000的所有整数。每次循环中,将当前的整数i
加到total
中。循环结束后,total
中就包含了1到1000的累加和,最终通过print
函数输出结果。
二、使用while循环
total = 0
i = 1
while i <= 1000:
total += i
i += 1
print("The sum from 1 to 1000 is:", total)
while
循环也可以达到同样的效果。与for
循环不同的是,while
循环需要手动维护循环变量。在这个示例中,我们初始将i
设置为1,并在每次循环中将i
加1,直到i
超过1000为止。在每次循环中,将当前的i
加到total
中。最终输出结果。
三、使用数学公式
total = (1000 * (1000 + 1)) // 2
print("The sum from 1 to 1000 is:", total)
使用数学公式是一种非常高效的方法。我们可以利用高斯求和公式:n(n+1)/2
,其中n
是最大数。对于从1到1000的累加和,公式为1000*(1000+1)/2
。这个方法不需要循环,计算效率非常高。
四、使用内置函数sum()
total = sum(range(1, 1001))
print("The sum from 1 to 1000 is:", total)
Python提供了一个内置函数sum()
,可以直接对可迭代对象进行求和。通过传入range(1, 1001)
,我们可以直接得到从1到1000的累加和。
五、使用递归函数
def recursive_sum(n):
if n == 1:
return 1
else:
return n + recursive_sum(n - 1)
total = recursive_sum(1000)
print("The sum from 1 to 1000 is:", total)
递归是一种函数调用自身的编程技术。定义一个函数recursive_sum
,递归地计算从1到1000的累加和。注意递归深度过大会导致栈溢出,不推荐用于大范围的累加。
六、使用生成器表达式
total = sum(i for i in range(1, 1001))
print("The sum from 1 to 1000 is:", total)
生成器表达式是一种内存友好的迭代方法。通过生成器表达式i for i in range(1, 1001)
,我们可以在不占用大量内存的情况下,逐个生成并累加从1到1000的整数。
七、使用NumPy库
import numpy as np
total = np.sum(np.arange(1, 1001))
print("The sum from 1 to 1000 is:", total)
NumPy
是一个强大的科学计算库。使用NumPy
的arange
函数生成从1到1000的数组,再使用sum
函数计算数组的累加和。这种方法适用于需要进行复杂数值计算的场景。
八、使用reduce函数
from functools import reduce
total = reduce(lambda x, y: x + y, range(1, 1001))
print("The sum from 1 to 1000 is:", total)
reduce
函数可以对可迭代对象进行累计运算。通过传入一个匿名函数lambda x, y: x + y
和range(1, 1001)
,我们可以实现从1到1000的累加和。
九、使用列表推导式
total = sum([i for i in range(1, 1001)])
print("The sum from 1 to 1000 is:", total)
列表推导式是一种简洁的生成列表的方法。通过列表推导式生成从1到1000的列表,并使用sum
函数计算累加和。
十、使用itertools.accumulate函数
import itertools
total = list(itertools.accumulate(range(1, 1001)))[-1]
print("The sum from 1 to 1000 is:", total)
itertools
模块提供了许多有用的迭代工具。使用accumulate
函数可以逐步累加可迭代对象。通过list
将结果转换为列表,取最后一个元素即为累加和。
十一、使用pandas库
import pandas as pd
total = pd.Series(range(1, 1001)).sum()
print("The sum from 1 to 1000 is:", total)
pandas
是一个强大的数据分析库。使用pandas
的Series
对象生成从1到1000的序列,再使用sum
函数计算累加和。
十二、使用map函数
total = sum(map(int, range(1, 1001)))
print("The sum from 1 to 1000 is:", total)
map
函数可以对可迭代对象的每个元素应用指定函数。在这个示例中,map(int, range(1, 1001))
将所有整数转换为int
类型,再使用sum
函数计算累加和。
十三、使用多线程
from concurrent.futures import ThreadPoolExecutor
def partial_sum(start, end):
return sum(range(start, end + 1))
with ThreadPoolExecutor() as executor:
futures = [executor.submit(partial_sum, i, i + 99) for i in range(1, 1001, 100)]
total = sum(f.result() for f in futures)
print("The sum from 1 to 1000 is:", total)
多线程可以并行计算多个部分的累加和,再将结果汇总。使用ThreadPoolExecutor
创建线程池,将累加任务分解为多个部分,最终汇总结果。
十四、使用多进程
from multiprocessing import Pool
def partial_sum(start, end):
return sum(range(start, end + 1))
with Pool() as pool:
results = pool.starmap(partial_sum, [(i, i + 99) for i in range(1, 1001, 100)])
total = sum(results)
print("The sum from 1 to 1000 is:", total)
多进程可以充分利用多核CPU的计算能力。使用multiprocessing.Pool
创建进程池,将累加任务分解为多个部分,通过starmap
函数并行计算,最终汇总结果。
十五、使用分治法
def divide_and_conquer_sum(start, end):
if start == end:
return start
mid = (start + end) // 2
left_sum = divide_and_conquer_sum(start, mid)
right_sum = divide_and_conquer_sum(mid + 1, end)
return left_sum + right_sum
total = divide_and_conquer_sum(1, 1000)
print("The sum from 1 to 1000 is:", total)
分治法是一种递归思想。将问题分解为两个子问题,分别计算子问题的结果,再将结果合并。通过分治法,可以有效减少计算复杂度。
以上是使用Python编写从1加到1000的多种方法。每种方法都有其优缺点,选择适合自己需求的方法,能够提高编程效率和代码质量。
相关问答FAQs:
如何使用Python实现从1加到1000的功能?
在Python中,可以使用for
循环或sum
函数来实现从1加到1000。以下是一个简单的示例代码:
total = sum(range(1, 1001))
print(total)
这个代码段使用sum
和range
函数高效地计算出总和。
Python中有没有更简洁的方法来计算从1加到1000的结果?
确实可以使用数学公式来简化计算。对于连续整数的和,可以使用公式 n(n + 1)/2
,其中n是最后一个数字。在这种情况下,代码如下:
n = 1000
total = n * (n + 1) // 2
print(total)
这种方法计算速度更快,特别是在处理更大的数字时。
如果我想将从1加到1000的结果输出到一个文件中,该如何操作?
可以使用Python的文件操作功能将结果写入文件。以下是示例代码:
total = sum(range(1, 1001))
with open('sum_result.txt', 'w') as file:
file.write(f'The sum from 1 to 1000 is: {total}')
这样,计算结果将被保存到一个名为sum_result.txt
的文本文件中,方便后续查看。
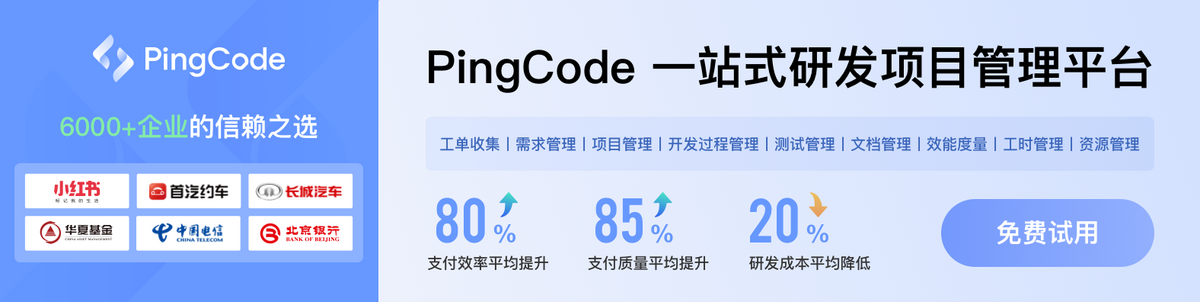