查看 Python 字符串的方法,可以使用内置的 dir()
函数、查阅官方文档、使用 help()
函数、查看第三方资源。最常用的是 dir()
函数,它会列出所有可用的方法,方便我们快速了解并使用。接下来,我们详细介绍 dir()
函数的使用方法。
Python 提供了许多字符串操作方法,这些方法可以帮助开发者处理和操作字符串。使用 dir()
函数可以快速列出字符串对象的所有方法。比如,可以在交互式解释器中输入 dir(str)
,就能看到字符串对象的所有方法。这些方法包括但不限于:capitalize()
, casefold()
, center()
, count()
, encode()
, endswith()
, expandtabs()
, find()
, format()
, format_map()
, index()
, isalnum()
, isalpha()
, isascii()
, isdecimal()
, isdigit()
, isidentifier()
, islower()
, isnumeric()
, isprintable()
, isspace()
, istitle()
, isupper()
, join()
, ljust()
, lower()
, lstrip()
, maketrans()
, partition()
, removeprefix()
, removesuffix()
, replace()
, rfind()
, rindex()
, rjust()
, rpartition()
, rsplit()
, rstrip()
, split()
, splitlines()
, startswith()
, strip()
, swapcase()
, title()
, translate()
, upper()
, zfill()
。
下面将详细介绍几个常用的方法及其用法。
一、capitalize()
和 casefold()
capitalize()
capitalize()
方法用于将字符串的首字符转换为大写,其他字符转换为小写。例如:
s = "hello world"
print(s.capitalize()) # 输出: Hello world
casefold()
casefold()
方法返回字符串的副本,所有字符都转换为小写。这个方法通常用于忽略大小写的比较。例如:
s = "Hello World"
print(s.casefold()) # 输出: hello world
二、center()
和 count()
center()
center(width, fillchar)
方法将字符串居中,并使用指定的填充字符(默认为空格)填充到指定的宽度。例如:
s = "hello"
print(s.center(10, '-')) # 输出: --hello---
count()
count(sub, start=0, end=len(string))
方法返回子字符串在指定范围内出现的次数。例如:
s = "hello world"
print(s.count('o')) # 输出: 2
三、encode()
和 endswith()
encode()
encode(encoding="utf-8", errors="strict")
方法将字符串编码为字节对象。例如:
s = "hello world"
print(s.encode()) # 输出: b'hello world'
endswith()
endswith(suffix, start=0, end=len(string))
方法判断字符串是否以指定的后缀结尾。例如:
s = "hello world"
print(s.endswith('world')) # 输出: True
四、expandtabs()
和 find()
expandtabs()
expandtabs(tabsize=8)
方法将字符串中的制表符转换为空格。例如:
s = "hello\tworld"
print(s.expandtabs(4)) # 输出: hello world
find()
find(sub, start=0, end=len(string))
方法返回子字符串在指定范围内第一次出现的索引,如果找不到则返回 -1。例如:
s = "hello world"
print(s.find('world')) # 输出: 6
五、format()
和 format_map()
format()
format(*args, kwargs)
方法用于格式化字符串。例如:
s = "Hello, {}!"
print(s.format("world")) # 输出: Hello, world!
format_map()
format_map(mapping)
方法类似于 format()
,但它接受一个字典作为参数。例如:
s = "Hello, {name}!"
print(s.format_map({'name': 'world'})) # 输出: Hello, world!
六、index()
和 isalnum()
index()
index(sub, start=0, end=len(string))
方法返回子字符串在指定范围内第一次出现的索引,如果找不到则抛出 ValueError
。例如:
s = "hello world"
print(s.index('world')) # 输出: 6
isalnum()
isalnum()
方法判断字符串是否全部由字母和数字组成。例如:
s = "hello123"
print(s.isalnum()) # 输出: True
七、isalpha()
和 isascii()
isalpha()
isalpha()
方法判断字符串是否全部由字母组成。例如:
s = "hello"
print(s.isalpha()) # 输出: True
isascii()
isascii()
方法判断字符串是否全部由 ASCII 字符组成。例如:
s = "hello"
print(s.isascii()) # 输出: True
八、isdecimal()
和 isdigit()
isdecimal()
isdecimal()
方法判断字符串是否全部由十进制数字组成。例如:
s = "123"
print(s.isdecimal()) # 输出: True
isdigit()
isdigit()
方法判断字符串是否全部由数字组成。例如:
s = "123"
print(s.isdigit()) # 输出: True
九、isidentifier()
和 islower()
isidentifier()
isidentifier()
方法判断字符串是否是合法的标识符。例如:
s = "hello"
print(s.isidentifier()) # 输出: True
islower()
islower()
方法判断字符串是否全部由小写字母组成。例如:
s = "hello"
print(s.islower()) # 输出: True
十、isnumeric()
和 isprintable()
isnumeric()
isnumeric()
方法判断字符串是否全部由数字组成,包括罗马数字等。例如:
s = "123"
print(s.isnumeric()) # 输出: True
isprintable()
isprintable()
方法判断字符串是否全部是可打印字符。例如:
s = "hello"
print(s.isprintable()) # 输出: True
十一、isspace()
和 istitle()
isspace()
isspace()
方法判断字符串是否全部由空白字符组成。例如:
s = " "
print(s.isspace()) # 输出: True
istitle()
istitle()
方法判断字符串是否是标题化的(每个单词的首字母大写)。例如:
s = "Hello World"
print(s.istitle()) # 输出: True
十二、isupper()
和 join()
isupper()
isupper()
方法判断字符串是否全部由大写字母组成。例如:
s = "HELLO"
print(s.isupper()) # 输出: True
join()
join(iterable)
方法用于将可迭代对象的元素连接成一个字符串。例如:
s = "-"
print(s.join(["hello", "world"])) # 输出: hello-world
十三、ljust()
和 lower()
ljust()
ljust(width, fillchar)
方法返回一个左对齐的字符串,并使用指定的填充字符(默认为空格)填充到指定的宽度。例如:
s = "hello"
print(s.ljust(10, '-')) # 输出: hello-----
lower()
lower()
方法返回一个将字符串中的所有字符转换为小写的副本。例如:
s = "HELLO"
print(s.lower()) # 输出: hello
十四、lstrip()
和 maketrans()
lstrip()
lstrip(chars)
方法返回一个移除字符串左侧指定字符的副本。例如:
s = " hello"
print(s.lstrip()) # 输出: hello
maketrans()
maketrans(x, y=None, z=None)
方法返回一个用于字符替换的映射表。例如:
s = "hello"
trans = str.maketrans("h", "H")
print(s.translate(trans)) # 输出: Hello
十五、partition()
和 removeprefix()
partition()
partition(sep)
方法将字符串拆分为一个 3 元组 (head, sep, tail),其中 sep 是分隔符。例如:
s = "hello world"
print(s.partition(" ")) # 输出: ('hello', ' ', 'world')
removeprefix()
removeprefix(prefix)
方法返回一个移除指定前缀的字符串副本。例如:
s = "hello world"
print(s.removeprefix("hello ")) # 输出: world
十六、removesuffix()
和 replace()
removesuffix()
removesuffix(suffix)
方法返回一个移除指定后缀的字符串副本。例如:
s = "hello world"
print(s.removesuffix(" world")) # 输出: hello
replace()
replace(old, new, count=-1)
方法返回一个将指定子字符串替换为新子字符串的副本。例如:
s = "hello world"
print(s.replace("world", "Python")) # 输出: hello Python
十七、rfind()
和 rindex()
rfind()
rfind(sub, start=0, end=len(string))
方法返回子字符串在指定范围内最后一次出现的索引,如果找不到则返回 -1。例如:
s = "hello world"
print(s.rfind('o')) # 输出: 7
rindex()
rindex(sub, start=0, end=len(string))
方法返回子字符串在指定范围内最后一次出现的索引,如果找不到则抛出 ValueError
。例如:
s = "hello world"
print(s.rindex('o')) # 输出: 7
十八、rjust()
和 rpartition()
rjust()
rjust(width, fillchar)
方法返回一个右对齐的字符串,并使用指定的填充字符(默认为空格)填充到指定的宽度。例如:
s = "hello"
print(s.rjust(10, '-')) # 输出: -----hello
rpartition()
rpartition(sep)
方法将字符串拆分为一个 3 元组 (head, sep, tail),其中 sep 是最后一个分隔符。例如:
s = "hello world"
print(s.rpartition(" ")) # 输出: ('hello', ' ', 'world')
十九、rsplit()
和 rstrip()
rsplit()
rsplit(sep=None, maxsplit=-1)
方法从字符串的右边开始拆分,返回一个列表。例如:
s = "hello world"
print(s.rsplit()) # 输出: ['hello', 'world']
rstrip()
rstrip(chars)
方法返回一个移除字符串右侧指定字符的副本。例如:
s = "hello "
print(s.rstrip()) # 输出: hello
二十、split()
和 splitlines()
split()
split(sep=None, maxsplit=-1)
方法拆分字符串,返回一个列表。例如:
s = "hello world"
print(s.split()) # 输出: ['hello', 'world']
splitlines()
splitlines(keepends=False)
方法按行拆分字符串,返回一个包含各行作为元素的列表。例如:
s = "hello\nworld"
print(s.splitlines()) # 输出: ['hello', 'world']
二十一、startswith()
和 strip()
startswith()
startswith(prefix, start=0, end=len(string))
方法判断字符串是否以指定前缀开始。例如:
s = "hello world"
print(s.startswith('hello')) # 输出: True
strip()
strip(chars)
方法返回一个移除字符串两侧指定字符的副本。例如:
s = " hello "
print(s.strip()) # 输出: hello
二十二、swapcase()
和 title()
swapcase()
swapcase()
方法返回一个将字符串中的大小写字母转换为相反大小写的副本。例如:
s = "Hello World"
print(s.swapcase()) # 输出: hELLO wORLD
title()
title()
方法返回一个标题化的字符串(每个单词的首字母大写)。例如:
s = "hello world"
print(s.title()) # 输出: Hello World
二十三、translate()
和 upper()
translate()
translate(table)
方法返回一个根据给定表替换后的字符串。例如:
s = "hello"
trans = str.maketrans("h", "H")
print(s.translate(trans)) # 输出: Hello
upper()
upper()
方法返回一个将字符串中的所有字符转换为大写的副本。例如:
s = "hello"
print(s.upper()) # 输出: HELLO
二十四、zfill()
zfill()
zfill(width)
方法返回一个长度为指定宽度的字符串,原字符串右对齐,前面填充0。例如:
s = "42"
print(s.zfill(5)) # 输出: 00042
这些字符串方法提供了丰富的功能,帮助我们在编写 Python 程序时更高效地处理字符串。通过使用 dir()
函数,我们可以轻松查看这些方法并加以利用,从而提升代码的可读性和可维护性。
相关问答FAQs:
如何获取Python字符串的所有可用方法和属性?
您可以使用内置的dir()
函数来获取Python字符串对象的所有可用方法和属性。只需在Python解释器中输入dir(str)
,即可查看字符串类的所有方法列表。此外,结合help()
函数,可以获取每个方法的详细说明。例如,help(str.method_name)
将提供特定方法的文档。
字符串方法有哪些常用的功能?
Python字符串提供了多种实用的方法,例如lower()
、upper()
、strip()
、replace()
和split()
等。这些方法能够帮助用户进行大小写转换、去除空白字符、替换子字符串以及分割字符串等操作。了解这些方法的作用,可以使字符串处理更加高效。
如何查找某个具体字符串方法的使用示例?
查找特定字符串方法的使用示例,可以通过访问Python官方文档或相关编程网站来获得。在Python官方文档中,每个字符串方法都有详细的说明和示例代码。此外,在线编程社区如Stack Overflow,也可以找到大量的实际应用案例和讨论,帮助您更好地理解和使用这些方法。
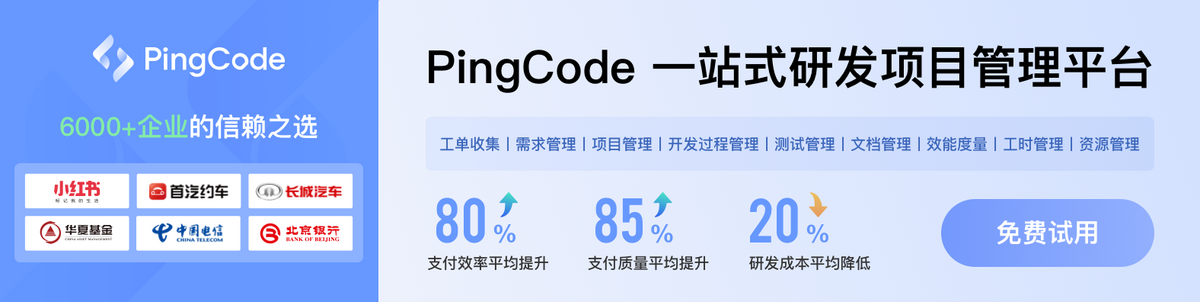