控制台结束无限循环Python的方法有:使用Ctrl+C组合键、使用try-except捕捉KeyboardInterrupt异常、设置条件变量来控制循环。其中,最常用且简单的方式是通过键盘快捷键Ctrl+C来强制终止无限循环。
在Python编程中,有时会编写一个无限循环来执行某些任务,然而在开发或调试过程中,可能需要手动结束这个循环。使用Ctrl+C组合键是最快速、最常见的方法。按下Ctrl+C会触发一个KeyboardInterrupt异常,从而终止正在运行的程序。
一、使用Ctrl+C组合键
在大多数操作系统的命令行终端或控制台中,可以通过按下Ctrl+C组合键来终止正在运行的Python程序。这种方法非常直接有效,尤其是在调试过程中。
例如,下面是一个简单的无限循环程序:
while True:
print("This is an infinite loop. Press Ctrl+C to stop.")
运行该程序后,可以通过按下Ctrl+C来终止它。
二、使用try-except捕捉KeyboardInterrupt异常
在某些情况下,可能希望在捕获到KeyboardInterrupt异常时执行一些清理操作或保存程序状态。这时,可以使用try-except语句来捕捉该异常。
try:
while True:
print("This is an infinite loop. Press Ctrl+C to stop.")
except KeyboardInterrupt:
print("Infinite loop has been interrupted and terminated.")
在上面的代码中,当用户按下Ctrl+C时,程序会捕捉到KeyboardInterrupt异常,并执行except块中的代码,然后正常退出程序。
三、设置条件变量来控制循环
除了手动终止循环,也可以通过设置一个条件变量来控制循环的执行。在需要终止循环时,改变条件变量的值即可。
continue_loop = True
while continue_loop:
print("This is a controlled loop. Type 'stop' to end.")
user_input = input("Enter command: ")
if user_input.lower() == 'stop':
continue_loop = False
print("Loop has been stopped.")
在这个示例中,循环根据用户输入的命令来决定是否继续。当用户输入“stop”时,条件变量continue_loop被设置为False,从而终止循环。
四、使用定时器来控制循环
有时需要在一段时间后自动终止循环,可以使用Python的定时器来实现这一功能。
import threading
def stop_loop():
global continue_loop
continue_loop = False
continue_loop = True
timer = threading.Timer(10, stop_loop) # 设置定时器为10秒
timer.start()
while continue_loop:
print("This is a timed loop. It will stop after 10 seconds.")
print("Loop has been stopped by the timer.")
在这个示例中,使用threading.Timer设置了一个10秒的定时器。当定时器触发时,stop_loop函数会将continue_loop变量设置为False,从而终止循环。
五、使用多线程和事件控制循环
在多线程环境中,可以使用事件对象来控制循环的执行和终止。
import threading
def run_loop(stop_event):
while not stop_event.is_set():
print("This is a loop controlled by threading.Event. Set the event to stop.")
stop_event.wait(1) # 每秒钟检查一次事件状态
stop_event = threading.Event()
loop_thread = threading.Thread(target=run_loop, args=(stop_event,))
loop_thread.start()
等待用户输入命令以终止循环
input("Press Enter to stop the loop.")
stop_event.set()
loop_thread.join()
print("Loop has been stopped by setting the event.")
在这个示例中,使用threading.Event对象来控制循环的终止。主线程等待用户输入,当用户按下Enter键时,设置事件,从而终止循环。
六、使用信号处理来控制循环
在Unix类操作系统中,可以使用信号处理来控制循环的终止。
import signal
import sys
def signal_handler(sig, frame):
print("Signal received, terminating the loop.")
sys.exit(0)
signal.signal(signal.SIGINT, signal_handler)
while True:
print("This is a loop controlled by signal handling. Press Ctrl+C to stop.")
在这个示例中,使用signal模块捕捉SIGINT信号(即Ctrl+C),并在捕捉到信号时执行signal_handler函数,从而终止循环。
七、使用循环计数器来控制循环
有时需要在运行一定次数后自动终止循环,可以使用循环计数器来实现这一功能。
max_iterations = 100
iteration_count = 0
while iteration_count < max_iterations:
print(f"This is iteration {iteration_count + 1}.")
iteration_count += 1
print("Loop has been stopped after reaching the maximum iteration count.")
在这个示例中,循环计数器iteration_count用于控制循环的执行次数。当计数器达到最大值时,循环自动终止。
八、使用线程和队列来控制循环
在多线程环境中,可以使用队列来控制循环的执行和终止。
import threading
import queue
def run_loop(command_queue):
while True:
try:
command = command_queue.get(timeout=1)
if command == 'stop':
break
print("This is a loop controlled by queue. Waiting for 'stop' command.")
except queue.Empty:
continue
command_queue = queue.Queue()
loop_thread = threading.Thread(target=run_loop, args=(command_queue,))
loop_thread.start()
等待用户输入命令以终止循环
input("Press Enter to stop the loop.")
command_queue.put('stop')
loop_thread.join()
print("Loop has been stopped by sending 'stop' command to the queue.")
在这个示例中,使用queue.Queue对象来控制循环的终止。主线程等待用户输入,当用户按下Enter键时,向队列发送“stop”命令,从而终止循环。
总结:在Python中,可以通过多种方法来终止无限循环,包括使用Ctrl+C组合键、try-except捕捉KeyboardInterrupt异常、设置条件变量、多线程和事件、信号处理、循环计数器、定时器以及队列。这些方法各有优劣,可以根据具体需求选择合适的方式来控制循环的执行和终止。
相关问答FAQs:
如何在Python中识别无限循环的迹象?
无限循环通常会导致程序不响应,CPU使用率飙升,或者程序在特定操作后似乎没有反应。常见的迹象包括程序的输出停滞不前、无法接收用户输入或界面完全冻结。监控程序的行为,尤其是在处理大量数据或复杂逻辑时,能帮助识别潜在的无限循环。
在控制台中,如何安全地终止Python程序?
如果你在控制台中运行Python程序并遇到无限循环,可以使用Ctrl + C
组合键来强制终止程序。这会发送一个中断信号,通常会导致Python解释器抛出KeyboardInterrupt
异常,从而停止执行当前的代码。确保在关键操作前保存数据,避免数据丢失。
如何在代码中预防无限循环的发生?
为了避免无限循环,建议在循环中设置合理的退出条件和计数器。可以使用while
循环配合条件判断,确保循环在一定条件下能够退出。此外,添加日志输出或进度指示器,能够帮助监控循环的执行情况,及时发现并处理潜在问题。
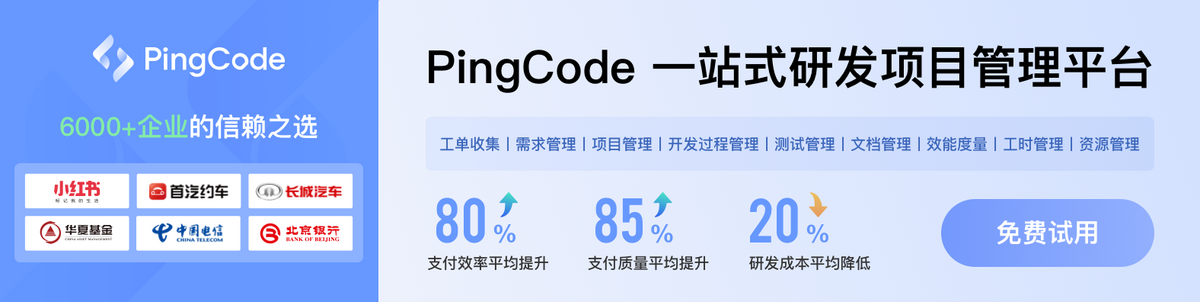