Python连接数据库操作的方法有:使用MySQL Connector/Python、使用SQLAlchemy、使用SQLite、使用Pandas直接连接数据库。其中,使用MySQL Connector/Python是比较常见的一种方法。它是一种数据库驱动程序,允许Python程序与MySQL数据库通信。下面将详细介绍如何使用MySQL Connector/Python来连接和操作数据库。
一、安装MySQL Connector/Python
在开始之前,我们需要确保安装了MySQL Connector/Python库。可以通过以下命令来安装:
pip install mysql-connector-python
二、连接到数据库
要连接到数据库,我们需要提供数据库的相关信息,比如主机名、用户名、密码和数据库名称。以下是一个简单的连接示例:
import mysql.connector
创建数据库连接
connection = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
检查连接是否成功
if connection.is_connected():
print("Successfully connected to the database")
在这里,我们使用mysql.connector.connect()
方法来创建数据库连接,并传入必要的参数。使用is_connected()
方法来检查连接是否成功。
三、执行SQL查询
连接成功后,我们可以使用cursor
对象来执行SQL查询。以下是一些常见的SQL操作示例:
1、创建表
cursor = connection.cursor()
cursor.execute("CREATE TABLE IF NOT EXISTS employees (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), salary INT)")
print("Table created successfully")
2、插入数据
sql = "INSERT INTO employees (name, salary) VALUES (%s, %s)"
values = ("John Doe", 70000)
cursor.execute(sql, values)
connection.commit()
print(cursor.rowcount, "record inserted.")
3、查询数据
cursor.execute("SELECT * FROM employees")
result = cursor.fetchall()
for row in result:
print(row)
4、更新数据
sql = "UPDATE employees SET salary = %s WHERE name = %s"
values = (75000, "John Doe")
cursor.execute(sql, values)
connection.commit()
print(cursor.rowcount, "record(s) affected")
5、删除数据
sql = "DELETE FROM employees WHERE name = %s"
values = ("John Doe",)
cursor.execute(sql, values)
connection.commit()
print(cursor.rowcount, "record(s) deleted")
四、使用SQLAlchemy
SQLAlchemy是Python中一个功能强大的数据库工具包,提供了高层次和低层次的SQL操作。它不仅支持MySQL,还支持SQLite、PostgreSQL等多种数据库。
1、安装SQLAlchemy
可以通过以下命令安装:
pip install SQLAlchemy
2、连接到数据库
from sqlalchemy import create_engine
engine = create_engine('mysql+mysqlconnector://yourusername:yourpassword@localhost/yourdatabase')
connection = engine.connect()
print("Connected to the database")
3、执行SQL查询
result = connection.execute("SELECT * FROM employees")
for row in result:
print(row)
五、使用SQLite
SQLite是一个轻量级的内嵌数据库,适用于小型应用程序。Python内置了SQLite库,因此无需额外安装。
1、连接到SQLite数据库
import sqlite3
connection = sqlite3.connect('example.db')
print("Opened database successfully")
2、执行SQL查询
cursor = connection.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS employees
(id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT NOT NULL,
salary REAL);''')
print("Table created successfully")
插入数据
cursor.execute("INSERT INTO employees (name, salary) VALUES ('John Doe', 70000)")
connection.commit()
print("Record inserted successfully")
查询数据
cursor.execute("SELECT * FROM employees")
result = cursor.fetchall()
for row in result:
print(row)
更新数据
cursor.execute("UPDATE employees SET salary = 75000 WHERE name = 'John Doe'")
connection.commit()
print("Record updated successfully")
删除数据
cursor.execute("DELETE FROM employees WHERE name = 'John Doe'")
connection.commit()
print("Record deleted successfully")
六、使用Pandas直接连接数据库
Pandas是一个强大的数据分析工具,支持直接从数据库读取数据。
1、安装Pandas和SQLAlchemy
pip install pandas sqlalchemy
2、连接到数据库并读取数据
import pandas as pd
from sqlalchemy import create_engine
engine = create_engine('mysql+mysqlconnector://yourusername:yourpassword@localhost/yourdatabase')
df = pd.read_sql('SELECT * FROM employees', con=engine)
print(df)
使用Pandas,可以直接将数据库中的数据读取为DataFrame格式,方便进行数据分析和处理。
总结
通过本文的介绍,我们了解了Python如何连接和操作数据库的方法,包括使用MySQL Connector/Python、SQLAlchemy、SQLite和Pandas。不同的方法有不同的优缺点,选择合适的方法可以大大提高开发效率。在实际应用中,可以根据需求选择合适的数据库连接和操作方法。
相关问答FAQs:
如何使用Python连接MySQL数据库?
要连接MySQL数据库,您需要使用Python的mysql-connector
或PyMySQL
库。首先,确保您已安装所需的库。可以通过运行pip install mysql-connector-python
或pip install PyMySQL
来安装。连接步骤如下:导入库,使用connect()
方法提供数据库的主机名、用户名、密码和数据库名。连接成功后,您可以创建游标对象来执行SQL查询。
Python连接数据库时常见的错误有哪些?
在连接数据库时,可能会遇到各种错误,例如“Access denied for user”(用户访问被拒绝)、"Unknown database"(未知数据库)或“Connection timed out”(连接超时)。这些错误通常与提供的凭据、数据库服务状态或网络连接有关。检查用户名、密码、数据库名称是否正确,并确保数据库服务器正在运行且可访问。
如何使用Python执行SQL语句?
在Python中执行SQL语句通常涉及几个步骤。首先,通过连接对象创建游标(cursor),然后使用游标的execute()
方法传入SQL查询。执行完毕后,可以使用fetchall()
或fetchone()
方法获取查询结果。如果要提交更改(如INSERT、UPDATE或DELETE),请调用连接对象的commit()
方法。确保在完成操作后关闭游标和连接,以释放资源。
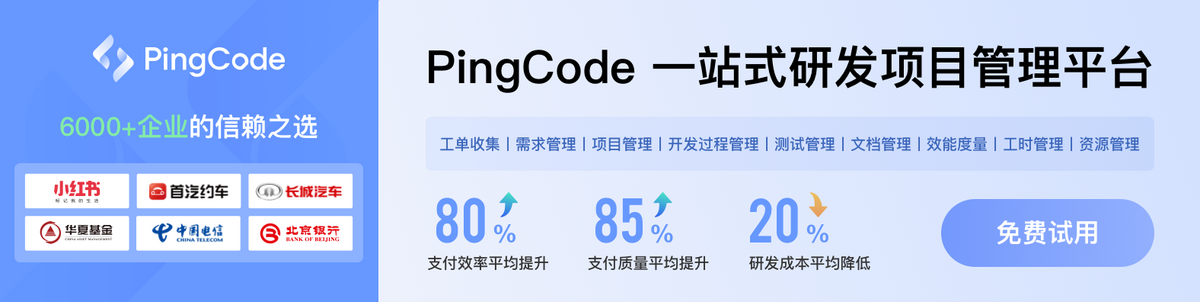