Python保存当时运行进度的方法包括:使用日志记录、持久化保存、检查点文件保存。这些方法可以确保您的程序在意外中断后能够从中断点继续运行。持久化保存是一种常用且高效的方法,下面将详细介绍。
持久化保存涉及将程序运行的状态数据保存到文件中,在程序重新启动时读取这些数据,以恢复之前的运行状态。这种方法适用于需要长时间运行且可能在中途被中断的程序,如数据处理、机器学习模型训练等。
一、日志记录
日志记录是一种常见的保存运行进度的方法,适用于需要记录程序执行的详细信息。Python的logging
模块提供了强大的日志记录功能,可以将程序的运行进度记录到日志文件中。
使用logging模块
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, filename='progress.log', filemode='w',
format='%(asctime)s - %(levelname)s - %(message)s')
def process_data(data):
for i, item in enumerate(data):
logging.info(f'Processing item {i}/{len(data)}: {item}')
# 模拟处理
time.sleep(1)
data = ['item1', 'item2', 'item3']
process_data(data)
通过这种方式,我们可以在日志文件progress.log
中查看程序的运行进度。
二、持久化保存
持久化保存是通过将程序的运行状态数据保存到文件中,以便在程序重新启动时能够恢复之前的状态。常用的方法有使用pickle模块保存对象状态和使用数据库保存进度。
使用pickle模块
import pickle
def save_progress(data, filename='progress.pkl'):
with open(filename, 'wb') as f:
pickle.dump(data, f)
def load_progress(filename='progress.pkl'):
try:
with open(filename, 'rb') as f:
return pickle.load(f)
except FileNotFoundError:
return None
data = load_progress() or {'current_index': 0, 'data': ['item1', 'item2', 'item3']}
for i in range(data['current_index'], len(data['data'])):
print(f'Processing item {i}: {data["data"][i]}')
# 模拟处理
time.sleep(1)
data['current_index'] = i + 1
save_progress(data)
通过这种方式,我们可以在程序重新启动时恢复之前的运行进度。
使用数据库保存进度
import sqlite3
def init_db():
conn = sqlite3.connect('progress.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS progress (current_index INTEGER)''')
cursor.execute('''INSERT INTO progress (current_index) VALUES (0)''')
conn.commit()
conn.close()
def get_progress():
conn = sqlite3.connect('progress.db')
cursor = conn.cursor()
cursor.execute('SELECT current_index FROM progress')
current_index = cursor.fetchone()[0]
conn.close()
return current_index
def save_progress(current_index):
conn = sqlite3.connect('progress.db')
cursor = conn.cursor()
cursor.execute('UPDATE progress SET current_index = ?', (current_index,))
conn.commit()
conn.close()
初始化数据库
init_db()
data = ['item1', 'item2', 'item3']
current_index = get_progress()
for i in range(current_index, len(data)):
print(f'Processing item {i}: {data[i]}')
# 模拟处理
time.sleep(1)
save_progress(i + 1)
通过这种方式,我们可以将程序的运行进度保存在数据库中,在程序重新启动时恢复之前的运行进度。
三、检查点文件保存
检查点文件保存是一种保存程序执行状态的方法,特别适用于需要定期保存运行进度的长时间运行的任务。检查点文件可以是文本文件、二进制文件或者数据库文件。
使用文本文件保存检查点
def save_checkpoint(current_index, filename='checkpoint.txt'):
with open(filename, 'w') as f:
f.write(str(current_index))
def load_checkpoint(filename='checkpoint.txt'):
try:
with open(filename, 'r') as f:
return int(f.read())
except FileNotFoundError:
return 0
data = ['item1', 'item2', 'item3']
current_index = load_checkpoint()
for i in range(current_index, len(data)):
print(f'Processing item {i}: {data[i]}')
# 模拟处理
time.sleep(1)
save_checkpoint(i + 1)
通过这种方式,我们可以将程序的运行进度保存在文本文件中,在程序重新启动时恢复之前的运行进度。
使用二进制文件保存检查点
import struct
def save_checkpoint(current_index, filename='checkpoint.bin'):
with open(filename, 'wb') as f:
f.write(struct.pack('I', current_index))
def load_checkpoint(filename='checkpoint.bin'):
try:
with open(filename, 'rb') as f:
return struct.unpack('I', f.read())[0]
except FileNotFoundError:
return 0
data = ['item1', 'item2', 'item3']
current_index = load_checkpoint()
for i in range(current_index, len(data)):
print(f'Processing item {i}: {data[i]}')
# 模拟处理
time.sleep(1)
save_checkpoint(i + 1)
通过这种方式,我们可以将程序的运行进度保存在二进制文件中,在程序重新启动时恢复之前的运行进度。
四、使用数据库保存复杂状态
对于较为复杂的程序状态,我们可以使用数据库保存进度,这样可以更方便地管理和查询程序的运行状态。常用的数据库有SQLite、MySQL、PostgreSQL等。
使用SQLite保存复杂状态
import sqlite3
def init_db():
conn = sqlite3.connect('complex_progress.db')
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS progress (
current_index INTEGER,
additional_info TEXT
)
''')
cursor.execute('INSERT INTO progress (current_index, additional_info) VALUES (0, "")')
conn.commit()
conn.close()
def get_progress():
conn = sqlite3.connect('complex_progress.db')
cursor = conn.cursor()
cursor.execute('SELECT current_index, additional_info FROM progress')
current_index, additional_info = cursor.fetchone()
conn.close()
return current_index, additional_info
def save_progress(current_index, additional_info):
conn = sqlite3.connect('complex_progress.db')
cursor = conn.cursor()
cursor.execute('UPDATE progress SET current_index = ?, additional_info = ?', (current_index, additional_info))
conn.commit()
conn.close()
初始化数据库
init_db()
data = ['item1', 'item2', 'item3']
current_index, additional_info = get_progress()
for i in range(current_index, len(data)):
print(f'Processing item {i}: {data[i]} with additional info: {additional_info}')
# 模拟处理
time.sleep(1)
additional_info = f'Processed {data[i]}'
save_progress(i + 1, additional_info)
通过这种方式,我们可以将程序的复杂状态保存在数据库中,在程序重新启动时恢复之前的运行进度。
五、使用Redis保存运行状态
Redis是一种高性能的键值数据库,适用于保存程序的运行状态。我们可以使用Redis保存程序的运行进度,以便在程序重新启动时恢复之前的状态。
使用Redis保存运行状态
import redis
def save_progress(current_index, additional_info):
r = redis.Redis(host='localhost', port=6379, db=0)
r.set('current_index', current_index)
r.set('additional_info', additional_info)
def load_progress():
r = redis.Redis(host='localhost', port=6379, db=0)
current_index = int(r.get('current_index') or 0)
additional_info = r.get('additional_info') or b''
return current_index, additional_info.decode('utf-8')
data = ['item1', 'item2', 'item3']
current_index, additional_info = load_progress()
for i in range(current_index, len(data)):
print(f'Processing item {i}: {data[i]} with additional info: {additional_info}')
# 模拟处理
time.sleep(1)
additional_info = f'Processed {data[i]}'
save_progress(i + 1, additional_info)
通过这种方式,我们可以将程序的运行状态保存在Redis中,在程序重新启动时恢复之前的运行进度。
总结
在本文中,我们讨论了Python保存当时运行进度的几种方法,包括日志记录、持久化保存、检查点文件保存、使用数据库保存复杂状态和使用Redis保存运行状态。这些方法可以帮助我们在程序意外中断后恢复运行进度,确保程序的连续性和可靠性。
不同的方法适用于不同的场景,日志记录适用于记录详细的运行信息,持久化保存适用于保存简单的运行状态,检查点文件保存适用于定期保存进度,使用数据库保存复杂状态适用于需要管理复杂状态的数据,使用Redis保存运行状态适用于需要高性能的场景。根据具体需求选择合适的方法,可以有效地保存程序的运行进度,提高程序的健壮性和可靠性。
相关问答FAQs:
如何在Python程序中保存运行进度?
在Python中,可以通过多种方式保存程序的运行进度,例如使用文件、数据库或序列化工具。常见的方法包括将进度信息写入文本文件或使用JSON格式保存,以便在下次运行时读取这些信息,恢复到之前的状态。利用Python的pickle
模块也可以方便地序列化对象并保存到文件中。
使用哪种数据结构最适合存储运行进度?
在Python中,字典(dict)是一种非常灵活的数据结构,适合用来存储运行进度的各种信息。字典可以保存键值对,允许你以清晰的方式组织和访问进度信息。此外,列表(list)也可以用于跟踪多个步骤的完成状态,具体选择应根据项目需求而定。
如何确保保存的进度数据在程序崩溃时不会丢失?
为了确保在程序崩溃时不会丢失保存的进度数据,建议在每个重要步骤之后立即保存当前进度。这可以通过定期写入文件来实现。例如,可以设置一个定时器或使用条件语句在完成特定任务后保存状态。这样即便程序意外退出,最近的进度也会被保留下来。
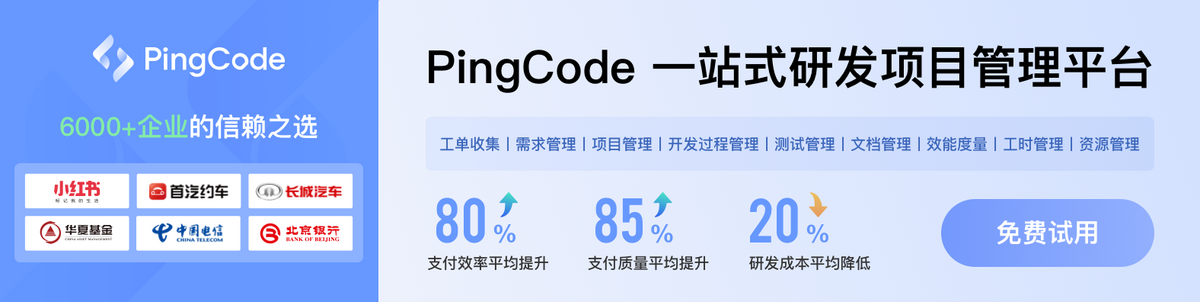