使用Python3获取a标签属性的方法有多种,主要包括使用BeautifulSoup、lxml、以及正则表达式等方式。 其中,BeautifulSoup 是最常用且最易于使用的库之一,因为它提供了简单的API来解析HTML文档,提取数据。下面将详细介绍如何使用这些方法来获取a标签的属性。
一、使用BeautifulSoup库
BeautifulSoup是一个用于解析HTML和XML文档的Python库。它创建一个解析树,使得用户可以轻松地导航、搜索和修改解析树。以下是使用BeautifulSoup获取a标签属性的步骤:
安装BeautifulSoup和相关库
首先,需要安装BeautifulSoup库及其依赖的解析器库 lxml
或 html.parser
。可以使用以下命令进行安装:
pip install beautifulsoup4
pip install lxml
使用BeautifulSoup解析HTML并获取a标签属性
以下是一个示例代码,展示如何使用BeautifulSoup来解析HTML并获取a标签的href属性:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head>
<title>Test Page</title>
</head>
<body>
<p><a href="http://example.com" id="link1">Example</a></p>
<p><a href="http://example.org" id="link2">Example Org</a></p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'lxml')
获取所有a标签
a_tags = soup.find_all('a')
遍历所有a标签并获取其href属性
for a_tag in a_tags:
href = a_tag.get('href')
print(f"Link: {href}")
详细说明
- 解析HTML文档: 使用
BeautifulSoup
创建一个解析树。 - 查找a标签: 使用
find_all
方法查找所有的a标签。 - 获取属性: 使用
get
方法获取a标签的href属性。
二、使用lxml库
lxml是另一个强大的库,用于处理HTML和XML。它比BeautifulSoup更快,但使用起来稍微复杂一些。以下是使用lxml获取a标签属性的示例:
安装lxml库
pip install lxml
使用lxml解析HTML并获取a标签属性
from lxml import etree
html_doc = """
<html>
<head>
<title>Test Page</title>
</head>
<body>
<p><a href="http://example.com" id="link1">Example</a></p>
<p><a href="http://example.org" id="link2">Example Org</a></p>
</body>
</html>
"""
解析HTML文档
tree = etree.HTML(html_doc)
获取所有a标签
a_tags = tree.xpath('//a')
遍历所有a标签并获取其href属性
for a_tag in a_tags:
href = a_tag.get('href')
print(f"Link: {href}")
详细说明
- 解析HTML文档: 使用
etree.HTML
创建一个解析树。 - 查找a标签: 使用
xpath
方法查找所有的a标签。 - 获取属性: 使用
get
方法获取a标签的href属性。
三、使用正则表达式
正则表达式是一个强大的工具,可以用来匹配复杂的字符串模式。虽然正则表达式在解析HTML时不如BeautifulSoup和lxml方便,但它们在处理简单的字符串模式时非常有效。以下是使用正则表达式获取a标签属性的示例:
使用正则表达式解析HTML并获取a标签属性
import re
html_doc = """
<html>
<head>
<title>Test Page</title>
</head>
<body>
<p><a href="http://example.com" id="link1">Example</a></p>
<p><a href="http://example.org" id="link2">Example Org</a></p>
</body>
</html>
"""
正则表达式模式
pattern = r'<a [^>]*href="([^"]+)"'
查找所有匹配项
matches = re.findall(pattern, html_doc)
输出所有匹配的href属性
for match in matches:
print(f"Link: {match}")
详细说明
- 定义正则表达式模式: 使用正则表达式模式匹配a标签的href属性。
- 查找匹配项: 使用
re.findall
方法查找所有匹配项。 - 输出匹配结果: 遍历所有匹配项并输出结果。
四、总结
通过以上三种方法,我们可以轻松地获取HTML文档中a标签的属性。使用BeautifulSoup和lxml库是最常见和推荐的方式,因为它们提供了强大的功能和易用的API。 相比之下,正则表达式适用于简单的字符串匹配,但在处理复杂的HTML文档时可能不太可靠。
总之,根据具体需求选择合适的方法,可以高效地提取HTML文档中的a标签属性。
相关问答FAQs:
如何在Python3中使用BeautifulSoup获取a标签的属性?
使用BeautifulSoup库可以方便地解析HTML文档并提取a标签的属性。首先,确保你已安装BeautifulSoup和requests库。然后,可以通过requests获取网页内容,并使用BeautifulSoup解析。获取a标签属性时,可以使用.get()
方法。例如:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for a in soup.find_all('a'):
href = a.get('href') # 获取href属性
title = a.get('title') # 获取title属性
print(f'链接: {href}, 标题: {title}')
在Python3中,是否可以获取多个a标签的所有属性?
绝对可以。你可以遍历所有的a标签,并使用.attrs
属性获取所有的属性字典。这种方式将返回一个包含所有属性及其值的字典。例如:
for a in soup.find_all('a'):
attributes = a.attrs # 获取所有属性
print(attributes)
使用Python3获取a标签属性时,如何处理缺失的属性?
在获取属性时,如果某个属性不存在,.get()
方法将返回None
,而使用.attrs
会返回一个空字典。你可以在访问属性前检查其是否存在,以避免抛出异常。例如:
for a in soup.find_all('a'):
href = a.get('href')
if href:
print(f'链接: {href}')
else:
print('该链接没有href属性')
以上内容将帮助你在Python3中有效获取和处理a标签的属性,满足各种需求。
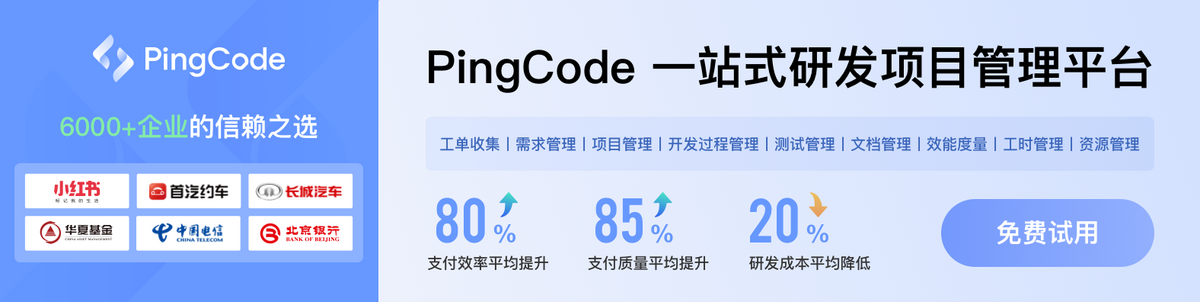