Python将ANSI码转为文本的方法有很多种,主要包括使用内置库、第三方库等方式。
在本文中,我们将深入探讨Python如何将ANSI码转为文本,具体方法包括使用内置库codecs
、第三方库chardet
以及手动处理。内置库codecs
的使用最为便捷,我们将详细介绍其使用方法。
一、使用codecs
库
Python的codecs
库提供了编码和解码的功能,支持多种编码方式,包括ANSI。我们可以使用它来将ANSI码转为文本。
1.1 codecs.open
方法
codecs.open
方法类似于内置的open
方法,但它允许我们指定文件的编码方式。以下是具体示例:
import codecs
def read_ansi_file(file_path):
with codecs.open(file_path, 'r', 'mbcs') as file:
content = file.read()
return content
file_path = 'path_to_your_ansi_file.txt'
text = read_ansi_file(file_path)
print(text)
在这个示例中,我们使用codecs.open
来打开文件,并指定编码方式为mbcs
,它是Windows系统中的默认编码方式,通常用于处理ANSI编码的文件。
1.2 codecs.decode
方法
如果你已经读取了一个包含ANSI编码的字节字符串,可以使用codecs.decode
方法来解码:
import codecs
def decode_ansi_bytes(byte_string):
return codecs.decode(byte_string, 'mbcs')
byte_string = b'\x41\x4e\x53\x49' # Example ANSI byte string
text = decode_ansi_bytes(byte_string)
print(text)
在这个示例中,codecs.decode
方法将ANSI编码的字节字符串解码为文本。
二、使用chardet
库
chardet
是一个强大的第三方库,用于检测未知编码的文本。我们可以用它来检测文件的编码,然后再进行解码。
2.1 安装chardet
首先,安装chardet
库:
pip install chardet
2.2 检测并解码
以下是使用chardet
库检测编码并解码的示例:
import chardet
def read_file_with_chardet(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
return raw_data.decode(encoding)
file_path = 'path_to_your_ansi_file.txt'
text = read_file_with_chardet(file_path)
print(text)
在这个示例中,chardet.detect
方法会返回一个字典,其中包含检测到的编码。然后,我们使用该编码来解码字节字符串。
三、手动处理
在某些情况下,可能需要手动处理ANSI编码,尤其是当文件包含混合编码或其他特殊字符时。以下是一个简单的手动处理示例:
def manual_decode_ansi(byte_string):
return byte_string.decode('latin1') # 'latin1' is often used as a fallback for ANSI
byte_string = b'\x41\x4e\x53\x49' # Example ANSI byte string
text = manual_decode_ansi(byte_string)
print(text)
在这个示例中,我们使用latin1
编码作为回退,这在某些情况下可以处理ANSI编码。
四、处理包含ANSI编码的文件
在实际项目中,处理包含ANSI编码的文件可能需要更多的步骤和错误处理。以下是一个更为全面的示例:
import codecs
import chardet
def read_ansi_file(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
if encoding is None:
encoding = 'mbcs' # Fallback to 'mbcs' if encoding detection fails
try:
text = raw_data.decode(encoding)
except UnicodeDecodeError:
text = raw_data.decode('latin1') # Fallback to 'latin1' if decoding fails
return text
file_path = 'path_to_your_ansi_file.txt'
text = read_ansi_file(file_path)
print(text)
在这个示例中,我们首先使用chardet
检测编码,如果检测失败,我们回退到mbcs
编码。如果解码过程中发生UnicodeDecodeError
,我们再回退到latin1
编码。这种方法可以提高代码的鲁棒性。
五、处理不同操作系统上的ANSI编码
不同操作系统对ANSI编码的处理方式可能有所不同,因此在编写跨平台代码时需要特别注意。以下是一些跨平台处理ANSI编码的建议:
5.1 Windows系统
在Windows系统上,mbcs
编码通常用于处理ANSI编码。以下是一个示例:
import codecs
def read_ansi_file_windows(file_path):
with codecs.open(file_path, 'r', 'mbcs') as file:
content = file.read()
return content
file_path = 'path_to_your_ansi_file.txt'
text = read_ansi_file_windows(file_path)
print(text)
5.2 Linux和macOS系统
在Linux和macOS系统上,ANSI编码通常使用latin1
或iso-8859-1
编码。以下是一个示例:
import codecs
def read_ansi_file_unix(file_path):
with codecs.open(file_path, 'r', 'latin1') as file:
content = file.read()
return content
file_path = 'path_to_your_ansi_file.txt'
text = read_ansi_file_unix(file_path)
print(text)
5.3 跨平台处理
为了编写跨平台代码,可以结合使用os
模块来检测操作系统,并选择适当的编码方式。以下是一个跨平台处理的示例:
import os
import codecs
def read_ansi_file_cross_platform(file_path):
if os.name == 'nt': # Windows
encoding = 'mbcs'
else: # Unix-like systems
encoding = 'latin1'
with codecs.open(file_path, 'r', encoding) as file:
content = file.read()
return content
file_path = 'path_to_your_ansi_file.txt'
text = read_ansi_file_cross_platform(file_path)
print(text)
在这个示例中,我们使用os.name
来检测操作系统,并选择适当的编码方式。
六、处理混合编码文件
有时文件中可能包含多种编码,处理这种文件需要更加复杂的方法。以下是一个处理混合编码文件的示例:
import chardet
def read_mixed_encoding_file(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
decoded_chunks = []
current_position = 0
while current_position < len(raw_data):
result = chardet.detect(raw_data[current_position:])
encoding = result['encoding']
if encoding is None:
break
chunk_size = result['confidence'] * 100
try:
decoded_chunk = raw_data[current_position:current_position+chunk_size].decode(encoding)
decoded_chunks.append(decoded_chunk)
current_position += chunk_size
except UnicodeDecodeError:
current_position += 1 # Skip problematic byte
text = ''.join(decoded_chunks)
return text
file_path = 'path_to_your_mixed_encoding_file.txt'
text = read_mixed_encoding_file(file_path)
print(text)
在这个示例中,我们使用chardet
库检测每个块的编码,并尝试逐块解码。如果解码失败,我们跳过有问题的字节。这种方法适用于处理包含多种编码的文件。
七、总结
将ANSI码转为文本在Python中有多种方法,包括使用内置库codecs
、第三方库chardet
以及手动处理。根据具体需求,可以选择适当的方法进行处理。在实际项目中,可能需要结合多种方法来处理复杂的编码情况,并注意跨平台兼容性。通过本文的详细介绍,希望能够帮助你更好地理解和处理ANSI编码的文件。
相关问答FAQs:
如何在Python中识别ANSI码并将其转换为文本?
在Python中,可以使用正则表达式库(如re
)来识别ANSI码并提取文本。通过编写匹配ANSI码的正则表达式,可以过滤掉ANSI控制字符,留下纯文本。例如,可以使用re.sub()
方法来替换ANSI码为一个空字符串,从而实现提取文本的目的。
是否有现成的库可以简化ANSI码转换的过程?
是的,Python中有一些库可以帮助简化ANSI码的转换过程,例如colorama
和ansi2html
等。这些库提供了方便的API,可以轻松地将包含ANSI码的字符串转换为相应的文本格式,或将其转换为HTML格式,便于在网页上显示。
在处理ANSI码时,有哪些常见的错误需要避免?
在处理ANSI码时,一些常见的错误包括未正确识别ANSI序列、未考虑到不同终端的ANSI码实现差异,以及未进行充分的错误处理。例如,确保在处理输入时,清楚地了解所使用的ANSI码格式,并在代码中实现适当的异常捕获,以防止程序因意外输入而崩溃。
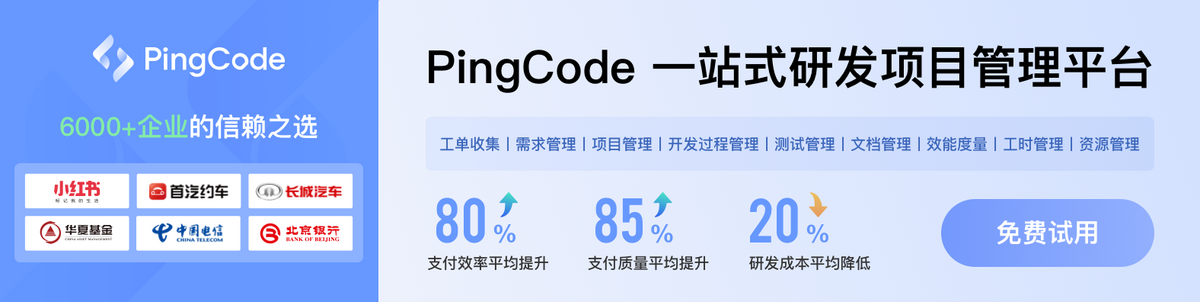