要将时间戳转换为字符串,可以使用Python中的datetime模块、strftime方法、time模块等方法。 其中一种常用的方法是使用datetime模块的fromtimestamp方法将时间戳转换为datetime对象,然后使用strftime方法将其格式化为字符串。接下来,我将详细介绍这种方法的具体步骤。
一、使用datetime模块
-
导入datetime模块:
from datetime import datetime
-
将时间戳转换为datetime对象:
timestamp = 1609459200 # 这是一个示例时间戳
dt_object = datetime.fromtimestamp(timestamp)
-
将datetime对象格式化为字符串:
date_string = dt_object.strftime('%Y-%m-%d %H:%M:%S')
print(date_string) # 输出: 2021-01-01 00:00:00
通过上述步骤,我们可以将时间戳成功转换为字符串格式。接下来我们将详细介绍每个步骤及更多方法。
二、time模块的方法
Python的time模块也提供了将时间戳转换为字符串的方法。以下是使用time模块的方法:
-
导入time模块:
import time
-
使用time.localtime将时间戳转换为struct_time对象:
timestamp = 1609459200 # 这是一个示例时间戳
time_object = time.localtime(timestamp)
-
将struct_time对象格式化为字符串:
date_string = time.strftime('%Y-%m-%d %H:%M:%S', time_object)
print(date_string) # 输出: 2021-01-01 00:00:00
三、pandas模块的方法
如果你正在处理大量数据,pandas库也提供了方便的方法来处理时间戳。以下是使用pandas的方法:
-
导入pandas模块:
import pandas as pd
-
将时间戳转换为pandas的datetime对象:
timestamp = 1609459200 # 这是一个示例时间戳
date_time = pd.to_datetime(timestamp, unit='s')
-
将datetime对象格式化为字符串:
date_string = date_time.strftime('%Y-%m-%d %H:%M:%S')
print(date_string) # 输出: 2021-01-01 00:00:00
四、使用arrow库
Arrow是一个更现代和易用的日期和时间处理库。以下是使用arrow的方法:
-
安装arrow库:
pip install arrow
-
导入arrow库并将时间戳转换为arrow对象:
import arrow
timestamp = 1609459200 # 这是一个示例时间戳
date_time = arrow.get(timestamp)
-
将arrow对象格式化为字符串:
date_string = date_time.format('YYYY-MM-DD HH:mm:ss')
print(date_string) # 输出: 2021-01-01 00:00:00
五、更多时间格式化选项
在上面的示例中,我们使用了'%Y-%m-%d %H:%M:%S'
作为格式化字符串。Python的strftime
方法提供了多种格式化选项,以下是一些常用的格式化选项:
%Y
:四位数的年份(如:2021)%m
:两位数的月份(如:01)%d
:两位数的日期(如:01)%H
:两位数的小时(00-23)%M
:两位数的分钟(00-59)%S
:两位数的秒(00-59)
你可以根据需要组合这些格式化选项。例如:
date_string = dt_object.strftime('%A, %B %d, %Y %I:%M%p')
print(date_string) # 输出: Friday, January 01, 2021 12:00AM
六、处理时区问题
在处理时间戳时,时区也是一个需要注意的问题。Python的datetime模块提供了时区支持。以下是一个带时区的示例:
-
导入datetime和pytz模块:
from datetime import datetime
import pytz
-
将时间戳转换为带时区的datetime对象:
timestamp = 1609459200 # 这是一个示例时间戳
timezone = pytz.timezone('America/New_York')
dt_object = datetime.fromtimestamp(timestamp, timezone)
-
将带时区的datetime对象格式化为字符串:
date_string = dt_object.strftime('%Y-%m-%d %H:%M:%S %Z%z')
print(date_string) # 输出: 2020-12-31 19:00:00 EST-0500
通过以上方法,可以根据你的具体需求,将时间戳转换为不同格式的字符串,并处理时区问题。希望这些方法能帮助你更好地处理时间戳转换任务。
相关问答FAQs:
如何在Python中将时间戳转换为可读的日期格式?
在Python中,可以使用datetime
模块将时间戳转换为可读的日期格式。通过datetime.fromtimestamp()
函数,可以将时间戳转换为本地时间的日期对象,然后使用strftime()
方法将其格式化为字符串。例如,datetime.fromtimestamp(timestamp).strftime('%Y-%m-%d %H:%M:%S')
将返回“年-月-日 时:分:秒”的格式。
时间戳的格式是什么?
时间戳通常是指自1970年1月1日00:00:00 UTC以来经过的秒数。在Python中,时间戳通常是一个浮点数,整数部分表示秒数,小数部分表示毫秒数。理解时间戳的结构有助于更有效地进行时间转换和计算。
如何处理不同的时区在时间戳转换中的影响?
在进行时间戳转换时,时区的处理非常重要。使用pytz
库可以帮助管理时区。可以将时间戳转换为UTC时间,然后根据需要转换为特定时区的本地时间。例如,首先将时间戳转为UTC时间,再通过astimezone()
方法调整为所需的时区,确保时间的准确性。
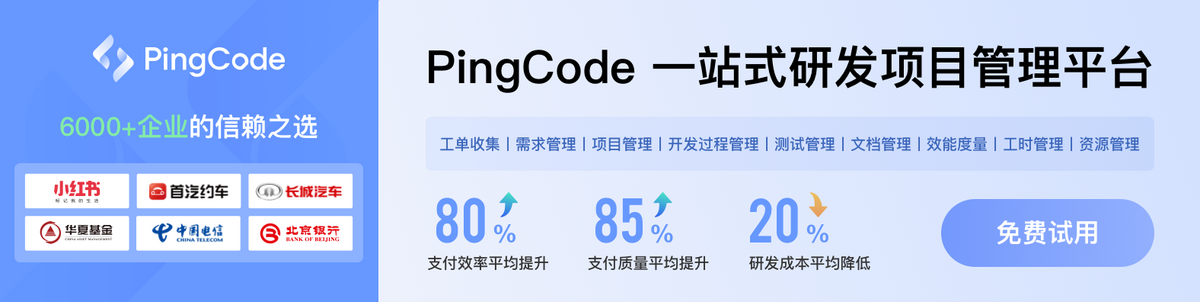