Python识别字符串的方法有很多,常见的包括使用内置函数、正则表达式、字符串方法等。其中,使用内置函数如isalpha()
、isdigit()
等是最简单直接的方式,而使用正则表达式则可以进行更复杂的模式匹配。正则表达式强大且灵活,适用于复杂的字符串处理需求,例如从一个文本中提取特定格式的信息。接下来将详细讲解这些方法。
一、使用内置函数识别字符串
Python 提供了一些内置函数用于检测字符串的属性,这些函数非常便捷并且高效。
1、isalpha()
isalpha()
方法用于检查字符串是否只包含字母。它返回布尔值 True
或 False
。
string = "HelloWorld"
print(string.isalpha()) # 输出: True
string_with_number = "Hello123"
print(string_with_number.isalpha()) # 输出: False
2、isdigit()
isdigit()
方法用于检查字符串是否只包含数字。它返回布尔值 True
或 False
。
numeric_string = "12345"
print(numeric_string.isdigit()) # 输出: True
alphanumeric_string = "123abc"
print(alphanumeric_string.isdigit()) # 输出: False
3、isalnum()
isalnum()
方法用于检查字符串是否只包含字母和数字。它返回布尔值 True
或 False
。
alphanumeric_string = "abc123"
print(alphanumeric_string.isalnum()) # 输出: True
special_char_string = "abc123!"
print(special_char_string.isalnum()) # 输出: False
4、isspace()
isspace()
方法用于检查字符串是否只包含空白字符(如空格、制表符、换行符)。它返回布尔值 True
或 False
。
space_string = " "
print(space_string.isspace()) # 输出: True
non_space_string = " abc "
print(non_space_string.isspace()) # 输出: False
二、使用正则表达式识别字符串
正则表达式是一个强大的字符串匹配工具,适用于更复杂的字符串处理需求。Python 提供了 re
模块来处理正则表达式。
1、基本使用
import re
pattern = r"\d+" # 匹配一个或多个数字
string = "The year is 2023"
match = re.search(pattern, string)
if match:
print(f"Found a match: {match.group()}") # 输出: Found a match: 2023
2、匹配特定格式
假设需要匹配电子邮件地址,可以使用如下正则表达式:
email_pattern = r"[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}"
email_string = "Please contact us at support@example.com"
email_match = re.search(email_pattern, email_string)
if email_match:
print(f"Found an email: {email_match.group()}") # 输出: Found an email: support@example.com
3、提取多个匹配项
如果需要提取字符串中所有符合特定模式的部分,可以使用 re.findall
方法。
pattern = r"\b\w+\b" # 匹配所有单词
text = "Python is great. Regular expressions are powerful."
words = re.findall(pattern, text)
print(words) # 输出: ['Python', 'is', 'great', 'Regular', 'expressions', 'are', 'powerful']
三、使用字符串方法识别字符串
Python 的字符串方法可以用来处理字符串并进行各种操作。
1、startswith()
和 endswith()
这些方法用于检查字符串是否以特定的子字符串开头或结尾。
string = "Hello, world!"
print(string.startswith("Hello")) # 输出: True
print(string.endswith("world!")) # 输出: True
2、find()
和 index()
这些方法用于查找子字符串在字符串中的位置。
string = "Hello, world!"
print(string.find("world")) # 输出: 7
print(string.index("world")) # 输出: 7
3、count()
count()
方法用于统计子字符串在字符串中出现的次数。
string = "banana"
print(string.count("a")) # 输出: 3
4、replace()
replace()
方法用于替换字符串中的子字符串。
string = "Hello, world!"
new_string = string.replace("world", "Python")
print(new_string) # 输出: Hello, Python!
四、实际应用场景
1、数据清洗
在数据科学中,清洗数据是一个重要的步骤。可以使用上述方法来识别和处理数据中的字符串。
data = ["123", "abc", "456", "def", "!@#"]
cleaned_data = [item for item in data if item.isalnum()]
print(cleaned_data) # 输出: ['123', 'abc', '456', 'def']
2、文本处理
处理文本文件时,经常需要识别和提取特定的内容。
with open("example.txt", "r") as file:
content = file.read()
emails = re.findall(email_pattern, content)
print(emails)
3、验证用户输入
在开发应用程序时,验证用户输入是一个常见需求。
username = input("Enter your username: ")
if username.isalnum():
print("Valid username")
else:
print("Invalid username")
通过以上方法,Python 提供了丰富的工具来识别和处理字符串。无论是简单的字符串属性检测,还是复杂的模式匹配,Python 都能提供高效的解决方案。
相关问答FAQs:
如何在Python中检查一个字符串是否包含特定的子字符串?
在Python中,可以使用in
运算符来检查一个字符串是否包含另一个字符串。比如,if '子字符串' in '目标字符串':
这种方式可以轻松实现这一功能。此外,str.find()
方法和str.index()
方法也可以用于查找子字符串的位置,返回-1或引发异常表示未找到。
Python中如何判断字符串的长度?
要获取字符串的长度,可以使用内置的len()
函数。例如,len('你的字符串')
将返回字符串中字符的数量。这个方法对于处理用户输入和验证数据非常有用。
在Python中如何将字符串转换为小写或大写?
Python提供了str.lower()
和str.upper()
方法来将字符串转换为小写或大写。示例:'你的字符串'.lower()
将返回小写形式,而'你的字符串'.upper()
将返回大写形式。这些方法对于标准化用户输入和输出非常实用。
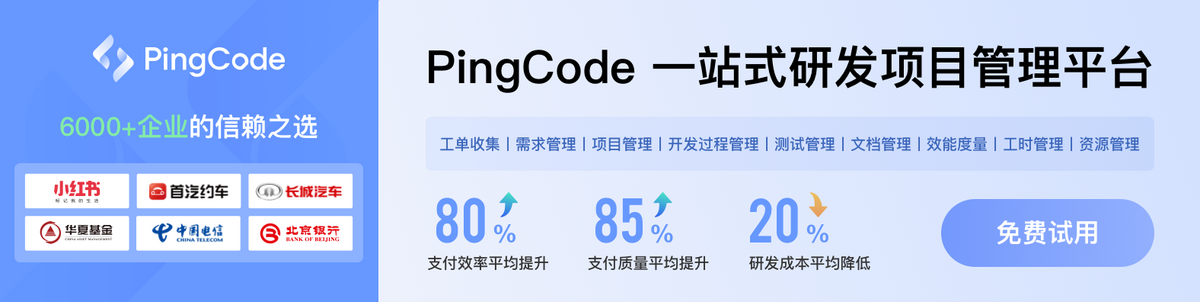