Python如何爬取外文翻译文献主要包括:使用requests库获取网页内容、使用BeautifulSoup库解析HTML、处理动态加载的网页内容、翻译网页内容。接下来,我们将详细介绍其中使用requests库获取网页内容这一点。
使用requests库获取网页内容是爬取外文翻译文献的第一步。requests库是Python中一个简单而强大的HTTP库,能够方便地发送HTTP请求。通过requests库,我们可以轻松地获取网页的HTML内容。下面是一个基本的示例:
import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print("Failed to retrieve the webpage")
在上面的示例中,我们首先导入requests库,然后使用requests.get()
方法发送GET请求获取网页内容。如果请求成功(即状态码为200),我们就可以通过response.text
属性获取网页的HTML内容。否则,我们输出失败信息。
接下来,我们将详细介绍Python爬取外文翻译文献的具体步骤。
一、使用requests库获取网页内容
requests库是一个非常流行的HTTP库,能够方便地发送HTTP请求并处理响应。下面是一些常见的用法:
1.1 发送GET请求
GET请求是最常见的HTTP请求,用于获取网页内容。可以使用requests.get()
方法发送GET请求,并获取响应对象。
import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print("Failed to retrieve the webpage")
1.2 发送POST请求
POST请求通常用于提交数据,例如登录表单。可以使用requests.post()
方法发送POST请求,并传递数据。
import requests
url = "http://example.com/login"
data = {
"username": "your_username",
"password": "your_password"
}
response = requests.post(url, data=data)
if response.status_code == 200:
content = response.text
print(content)
else:
print("Failed to retrieve the webpage")
1.3 处理请求头
有时需要自定义请求头,例如设置User-Agent、Cookie等。可以使用requests.get()
或requests.post()
方法的headers
参数传递请求头。
import requests
url = "http://example.com"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
content = response.text
print(content)
else:
print("Failed to retrieve the webpage")
二、使用BeautifulSoup库解析HTML
BeautifulSoup是一个用于解析HTML和XML文档的Python库,能够方便地提取数据。下面是一些常见的用法:
2.1 创建BeautifulSoup对象
首先,需要安装BeautifulSoup库,可以使用以下命令安装:
pip install beautifulsoup4
然后,可以使用BeautifulSoup库解析HTML内容。
from bs4 import BeautifulSoup
html_content = "<html><head><title>Example</title></head><body><h1>Hello, world!</h1></body></html>"
soup = BeautifulSoup(html_content, "html.parser")
2.2 查找元素
可以使用find()
和find_all()
方法查找元素。例如,查找标题和所有段落:
title = soup.find("title").text
paragraphs = soup.find_all("p")
print("Title:", title)
for p in paragraphs:
print("Paragraph:", p.text)
2.3 查找属性
可以使用get()
方法查找元素的属性。例如,查找所有链接的URL:
links = soup.find_all("a")
for link in links:
href = link.get("href")
print("Link:", href)
三、处理动态加载的网页内容
有些网页内容是通过JavaScript动态加载的,requests库无法直接获取这些内容。可以使用Selenium库模拟浏览器操作,处理动态加载的网页内容。
3.1 安装Selenium和浏览器驱动
首先,需要安装Selenium库和浏览器驱动。例如,安装Selenium库和Chrome浏览器驱动:
pip install selenium
下载Chrome浏览器驱动,并将其路径添加到系统环境变量中。
3.2 使用Selenium获取动态内容
可以使用Selenium模拟浏览器操作,获取动态加载的网页内容。例如,获取动态加载的网页内容:
from selenium import webdriver
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
content = driver.page_source
print(content)
driver.quit()
四、翻译网页内容
可以使用Google Translate API或其他翻译API翻译网页内容。下面是一个使用Google Translate API的示例:
from googletrans import Translator
translator = Translator()
text = "Hello, world!"
translated = translator.translate(text, src="en", dest="zh-cn")
print("Translated text:", translated.text)
五、综合示例
下面是一个综合示例,演示如何使用requests库获取网页内容,使用BeautifulSoup库解析HTML,使用Selenium处理动态加载的网页内容,并使用Google Translate API翻译内容:
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
from googletrans import Translator
Step 1: Use requests to get static content
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
content = response.text
else:
content = ""
Step 2: Use BeautifulSoup to parse HTML
soup = BeautifulSoup(content, "html.parser")
title = soup.find("title").text
paragraphs = soup.find_all("p")
Step 3: Use Selenium to get dynamic content
driver = webdriver.Chrome()
driver.get(url)
dynamic_content = driver.page_source
driver.quit()
Step 4: Use Google Translate API to translate content
translator = Translator()
translated_title = translator.translate(title, src="en", dest="zh-cn").text
translated_paragraphs = [translator.translate(p.text, src="en", dest="zh-cn").text for p in paragraphs]
Print translated content
print("Translated Title:", translated_title)
for translated_paragraph in translated_paragraphs:
print("Translated Paragraph:", translated_paragraph)
以上是如何使用Python爬取外文翻译文献的详细步骤,包括使用requests库获取网页内容、使用BeautifulSoup库解析HTML、处理动态加载的网页内容、翻译网页内容。希望这些内容对您有所帮助。
相关问答FAQs:
如何使用Python获取外文翻译文献的有效途径?
获取外文翻译文献的有效途径包括使用Python中的爬虫框架如Scrapy、BeautifulSoup或Requests库,这些工具能够帮助你抓取网页内容并提取所需信息。此外,利用API接口(如Google翻译API)可以直接进行翻译和获取文献的相关信息。确保遵循网站的使用条款和机器人协议,以避免法律问题。
在爬取外文翻译文献时,如何处理反爬虫机制?
许多网站实施反爬虫机制以保护其内容。为应对这一挑战,可以通过伪装用户代理、使用代理IP、调整请求频率和间隔时间等方式来避免被检测。同时,使用动态请求工具如Selenium也可以有效绕过一些简单的反爬虫措施。
如何确保爬取到的外文翻译文献的准确性和完整性?
确保翻译文献的准确性和完整性可以通过多种方式实现。首先,可以在爬取过程中进行数据清洗,去除冗余信息和错误数据。其次,利用翻译工具或服务时,建议通过多种工具进行交叉验证,以提高翻译结果的准确性。此外,检查文献的来源和作者背景,以确保内容的权威性和可靠性也非常重要。
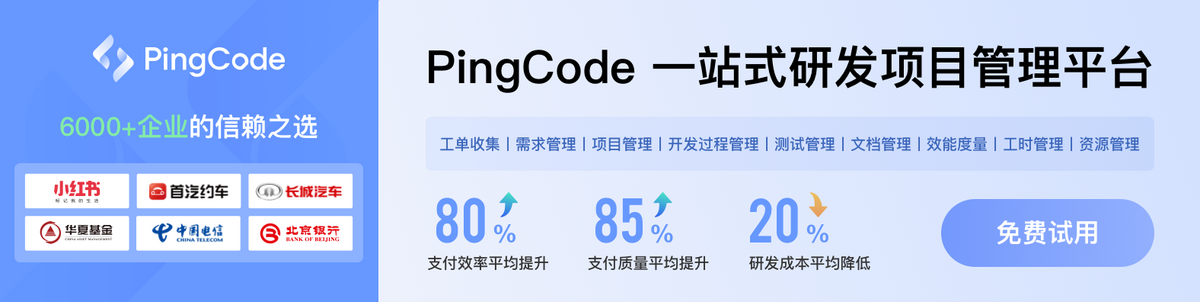