要用Python编写俄罗斯方块,可以使用Pygame库、掌握基本的Python编程技巧、熟悉俄罗斯方块的游戏规则。本文将详细介绍如何用Python编写俄罗斯方块游戏,包括游戏初始化、界面绘制、方块生成和移动、碰撞检测、消行逻辑等关键步骤。下面是具体的实现步骤。
一、安装和导入Pygame库
Pygame是一个跨平台的Python模块,用于编写视频游戏。它包括计算机图形和声音库。首先,你需要安装Pygame库,可以使用以下命令:
pip install pygame
安装完成后,在代码中导入Pygame库:
import pygame
import random
二、游戏初始化
初始化Pygame并设置游戏窗口的大小和标题。
pygame.init()
设置游戏窗口的大小
screen_width = 300
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
设置窗口标题
pygame.display.set_caption("俄罗斯方块")
三、定义常量和全局变量
定义一些必要的常量和全局变量,例如颜色、方块形状和游戏网格。
# 定义颜色
colors = [
(0, 0, 0), # 黑色
(255, 0, 0), # 红色
(0, 255, 0), # 绿色
(0, 0, 255), # 蓝色
(255, 255, 0), # 黄色
(255, 165, 0), # 橙色
(0, 255, 255), # 青色
(128, 0, 128) # 紫色
]
定义方块形状
shapes = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[0, 1, 0], [1, 1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 1], [1, 0, 0]]
]
网格大小
grid_width = 10
grid_height = 20
grid = [[0 for _ in range(grid_width)] for _ in range(grid_height)]
四、定义方块类
定义一个方块类来管理方块的属性和行为。
class Tetromino:
def __init__(self, shape):
self.shape = shape
self.color = random.randint(1, len(colors) - 1)
self.rotation = 0
self.x = grid_width // 2 - len(shape[0]) // 2
self.y = 0
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
def get_blocks(self):
return self.shape[self.rotation]
五、绘制游戏网格和方块
定义绘制游戏网格和方块的函数。
def draw_grid(screen, grid):
for y in range(grid_height):
for x in range(grid_width):
pygame.draw.rect(screen, colors[grid[y][x]], (x * 30, y * 30, 30, 30), 0)
pygame.draw.rect(screen, (255, 255, 255), (x * 30, y * 30, 30, 30), 1)
def draw_tetromino(screen, tetromino):
shape = tetromino.get_blocks()
for y, row in enumerate(shape):
for x, cell in enumerate(row):
if cell:
pygame.draw.rect(screen, colors[tetromino.color],
((tetromino.x + x) * 30, (tetromino.y + y) * 30, 30, 30), 0)
pygame.draw.rect(screen, (255, 255, 255),
((tetromino.x + x) * 30, (tetromino.y + y) * 30, 30, 30), 1)
六、方块移动和碰撞检测
定义方块的移动和碰撞检测函数。
def valid_move(grid, tetromino, dx, dy):
shape = tetromino.get_blocks()
for y, row in enumerate(shape):
for x, cell in enumerate(row):
if cell:
new_x = tetromino.x + x + dx
new_y = tetromino.y + y + dy
if new_x < 0 or new_x >= grid_width or new_y >= grid_height or grid[new_y][new_x]:
return False
return True
def place_tetromino(grid, tetromino):
shape = tetromino.get_blocks()
for y, row in enumerate(shape):
for x, cell in enumerate(row):
if cell:
grid[tetromino.y + y][tetromino.x + x] = tetromino.color
七、消行逻辑
定义消行的逻辑,当一行被填满时,将其移除并在顶部添加一行空行。
def clear_lines(grid):
full_lines = [y for y in range(grid_height) if all(grid[y])]
for y in full_lines:
del grid[y]
grid.insert(0, [0] * grid_width)
return len(full_lines)
八、游戏主循环
实现游戏的主循环,包括处理事件、更新游戏状态和绘制游戏画面。
def main():
clock = pygame.time.Clock()
current_tetromino = Tetromino(random.choice(shapes))
fall_time = 0
fall_speed = 500
running = True
while running:
screen.fill((0, 0, 0))
draw_grid(screen, grid)
draw_tetromino(screen, current_tetromino)
pygame.display.flip()
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and valid_move(grid, current_tetromino, -1, 0):
current_tetromino.x -= 1
elif event.key == pygame.K_RIGHT and valid_move(grid, current_tetromino, 1, 0):
current_tetromino.x += 1
elif event.key == pygame.K_DOWN and valid_move(grid, current_tetromino, 0, 1):
current_tetromino.y += 1
elif event.key == pygame.K_UP:
current_tetromino.rotate()
fall_time += clock.get_rawtime()
clock.tick()
if fall_time > fall_speed:
if valid_move(grid, current_tetromino, 0, 1):
current_tetromino.y += 1
else:
place_tetromino(grid, current_tetromino)
current_tetromino = Tetromino(random.choice(shapes))
if not valid_move(grid, current_tetromino, 0, 0):
running = False
fall_time = 0
clear_lines(grid)
pygame.quit()
if __name__ == "__main__":
main()
总结
通过以上步骤,我们实现了一个基本的俄罗斯方块游戏。我们使用Pygame库进行游戏开发,定义了游戏的初始化、网格和方块的绘制、方块的移动和碰撞检测、消行逻辑等关键功能。通过游戏主循环,我们实现了游戏的基本玩法。你可以在此基础上进行进一步的优化和扩展,例如增加游戏难度、实现更多的方块形状、添加音效等。希望这篇文章对你有所帮助,祝你编程愉快!
相关问答FAQs:
如何选择合适的Python库来开发俄罗斯方块游戏?
在开发俄罗斯方块游戏时,可以选择多个Python库来实现。Pygame是最常用的库之一,它提供了丰富的图形和音频功能,适合2D游戏开发。此外,使用Tkinter也可以创建简单的图形界面,适合初学者。如果你希望使用更高级的功能,可以考虑使用Arcade库,它提供了更现代的API和更好的性能。
编写俄罗斯方块游戏时,需要注意哪些关键的算法?
在编写俄罗斯方块游戏时,几个关键算法至关重要。首先是碰撞检测算法,确保方块在与其他方块或游戏边界接触时能够停止。其次是形状旋转和移动算法,确保玩家能够顺利操作方块。最后,消除填满行的算法也是非常重要的,它需要检查每一行是否已满,并相应地更新游戏状态。
如何优化俄罗斯方块游戏的性能?
为了优化俄罗斯方块游戏的性能,可以采用几种方法。使用双缓冲技术来减少屏幕闪烁,确保游戏画面更加流畅。在绘制方块时,只更新那些发生变化的部分,而不是重绘整个屏幕。此外,尽量减少不必要的计算,尤其是在游戏循环中,使用事件驱动的方法来响应用户输入,这样可以提升游戏的响应速度和整体流畅度。
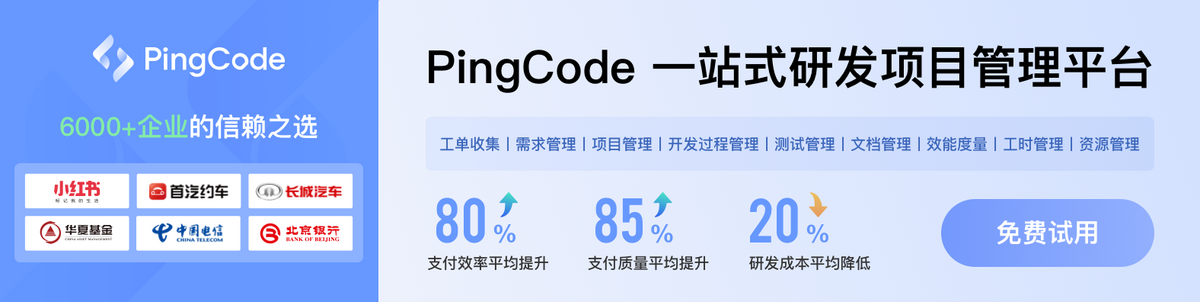