使用Python制作俄罗斯方块的基本步骤包括:设计游戏结构、使用Pygame库、处理游戏逻辑、绘制图形、实现用户输入控制、管理游戏状态。 其中,Pygame库是一个非常强大的工具,特别适合制作2D游戏。接下来,我将详细描述如何使用Python和Pygame库来实现一个简单的俄罗斯方块游戏。
一、安装和设置Pygame
在开始编码之前,确保你已经安装了Pygame库。你可以通过以下命令安装Pygame:
pip install pygame
安装完成后,创建一个新的Python文件,并导入必要的模块:
import pygame
import random
二、定义基本游戏参数
在编写实际代码之前,我们需要定义一些基本参数,比如窗口大小、颜色、方块形状等。
# 游戏窗口大小
WINDOW_WIDTH = 800
WINDOW_HEIGHT = 600
PLAY_WIDTH = 300 # 10 * 30 = 300
PLAY_HEIGHT = 600 # 20 * 30 = 600
BLOCK_SIZE = 30
游戏网格
TOP_LEFT_X = (WINDOW_WIDTH - PLAY_WIDTH) // 2
TOP_LEFT_Y = WINDOW_HEIGHT - PLAY_HEIGHT
定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
CYAN = (0, 255, 255)
MAGENTA = (255, 0, 255)
ORANGE = (255, 165, 0)
定义方块形状
S = [['.....',
'.....',
'..00.',
'.00..',
'.....'],
['.....',
'..0..',
'..00.',
'...0.',
'.....']]
Z = [['.....',
'.....',
'.00..',
'..00.',
'.....'],
['.....',
'..0..',
'.00..',
'.0...',
'.....']]
I = [['..0..',
'..0..',
'..0..',
'..0..',
'.....'],
['.....',
'0000.',
'.....',
'.....',
'.....']]
O = [['.....',
'.....',
'.00..',
'.00..',
'.....']]
J = [['.....',
'.0...',
'.000.',
'.....',
'.....'],
['.....',
'..00.',
'..0..',
'..0..',
'.....'],
['.....',
'.....',
'.000.',
'...0.',
'.....'],
['.....',
'..0..',
'..0..',
'.00..',
'.....']]
L = [['.....',
'...0.',
'.000.',
'.....',
'.....'],
['.....',
'..0..',
'..0..',
'..00.',
'.....'],
['.....',
'.....',
'.000.',
'.0...',
'.....'],
['.....',
'.00..',
'..0..',
'..0..',
'.....']]
T = [['.....',
'..0..',
'.000.',
'.....',
'.....'],
['.....',
'..0..',
'..00.',
'..0..',
'.....'],
['.....',
'.....',
'.000.',
'..0..',
'.....'],
['.....',
'..0..',
'.00..',
'..0..',
'.....']]
SHAPES = [S, Z, I, O, J, L, T]
SHAPE_COLORS = [GREEN, RED, CYAN, YELLOW, BLUE, ORANGE, MAGENTA]
三、定义游戏中的基本类
我们需要定义一些类来处理游戏中的核心对象,比如方块、网格等。
class Piece:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = SHAPE_COLORS[SHAPES.index(shape)]
self.rotation = 0
def create_grid(locked_positions={}):
grid = [[BLACK for _ in range(10)] for _ in range(20)]
for i in range(len(grid)):
for j in range(len(grid[i])):
if (j, i) in locked_positions:
c = locked_positions[(j, i)]
grid[i][j] = c
return grid
def convert_shape_format(piece):
positions = []
format = piece.shape[piece.rotation % len(piece.shape)]
for i, line in enumerate(format):
row = list(line)
for j, column in enumerate(row):
if column == '0':
positions.append((piece.x + j, piece.y + i))
for i, pos in enumerate(positions):
positions[i] = (pos[0] - 2, pos[1] - 4)
return positions
def valid_space(piece, grid):
accepted_positions = [[(j, i) for j in range(10) if grid[i][j] == BLACK] for i in range(20)]
accepted_positions = [j for sub in accepted_positions for j in sub]
formatted = convert_shape_format(piece)
for pos in formatted:
if pos not in accepted_positions:
if pos[1] > -1:
return False
return True
def check_lost(positions):
for pos in positions:
x, y = pos
if y < 1:
return True
return False
def get_shape():
return Piece(5, 0, random.choice(SHAPES))
四、绘制游戏窗口
绘制游戏窗口是游戏开发中的一个重要环节。我们需要定义一些函数来绘制网格、方块、边界等。
def draw_grid(surface, grid):
sx = TOP_LEFT_X
sy = TOP_LEFT_Y
for i in range(len(grid)):
pygame.draw.line(surface, WHITE, (sx, sy + i * BLOCK_SIZE), (sx + PLAY_WIDTH, sy + i * BLOCK_SIZE))
for j in range(len(grid[i])):
pygame.draw.line(surface, WHITE, (sx + j * BLOCK_SIZE, sy), (sx + j * BLOCK_SIZE, sy + PLAY_HEIGHT))
def draw_window(surface, grid, score=0):
surface.fill(BLACK)
pygame.font.init()
font = pygame.font.SysFont('comicsans', 60)
label = font.render('Tetris', 1, WHITE)
surface.blit(label, (TOP_LEFT_X + PLAY_WIDTH / 2 - (label.get_width() / 2), 30))
font = pygame.font.SysFont('comicsans', 30)
label = font.render('Score: ' + str(score), 1, WHITE)
sx = TOP_LEFT_X + PLAY_WIDTH + 50
sy = TOP_LEFT_Y + PLAY_HEIGHT / 2 - 100
surface.blit(label, (sx + 20, sy + 160))
for i in range(len(grid)):
for j in range(len(grid[i])):
pygame.draw.rect(surface, grid[i][j], (TOP_LEFT_X + j * BLOCK_SIZE, TOP_LEFT_Y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 0)
draw_grid(surface, grid)
pygame.draw.rect(surface, RED, (TOP_LEFT_X, TOP_LEFT_Y, PLAY_WIDTH, PLAY_HEIGHT), 5)
五、处理游戏逻辑
我们需要编写代码来处理游戏逻辑,比如方块的移动、旋转、消除行等。
def clear_rows(grid, locked):
increment = 0
for i in range(len(grid)-1, -1, -1):
row = grid[i]
if BLACK not in row:
increment += 1
ind = i
for j in range(len(row)):
try:
del locked[(j, i)]
except:
continue
if increment > 0:
for key in sorted(list(locked), key=lambda x: x[1])[::-1]:
x, y = key
if y < ind:
new_key = (x, y + increment)
locked[new_key] = locked.pop(key)
return increment
def draw_next_shape(piece, surface):
font = pygame.font.SysFont('comicsans', 30)
label = font.render('Next Shape', 1, WHITE)
sx = TOP_LEFT_X + PLAY_WIDTH + 50
sy = TOP_LEFT_Y + PLAY_HEIGHT / 2 - 100
format = piece.shape[piece.rotation % len(piece.shape)]
for i, line in enumerate(format):
row = list(line)
for j, column in enumerate(row):
if column == '0':
pygame.draw.rect(surface, piece.color, (sx + j * BLOCK_SIZE, sy + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 0)
surface.blit(label, (sx + 10, sy - 30))
六、主循环
最后,我们需要编写游戏的主循环来处理游戏的进行。
def main():
locked_positions = {}
grid = create_grid(locked_positions)
change_piece = False
run = True
current_piece = get_shape()
next_piece = get_shape()
clock = pygame.time.Clock()
fall_time = 0
score = 0
while run:
grid = create_grid(locked_positions)
fall_speed = 0.27
fall_time += clock.get_rawtime()
clock.tick()
if fall_time / 1000 >= fall_speed:
fall_time = 0
current_piece.y += 1
if not (valid_space(current_piece, grid)) and current_piece.y > 0:
current_piece.y -= 1
change_piece = True
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
pygame.display.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_piece.x -= 1
if not valid_space(current_piece, grid):
current_piece.x += 1
elif event.key == pygame.K_RIGHT:
current_piece.x += 1
if not valid_space(current_piece, grid):
current_piece.x -= 1
elif event.key == pygame.K_DOWN:
current_piece.y += 1
if not valid_space(current_piece, grid):
current_piece.y -= 1
elif event.key == pygame.K_UP:
current_piece.rotation += 1
if not valid_space(current_piece, grid):
current_piece.rotation -= 1
shape_pos = convert_shape_format(current_piece)
for i in range(len(shape_pos)):
x, y = shape_pos[i]
if y > -1:
grid[y][x] = current_piece.color
if change_piece:
for pos in shape_pos:
p = (pos[0], pos[1])
locked_positions[p] = current_piece.color
current_piece = next_piece
next_piece = get_shape()
change_piece = False
score += clear_rows(grid, locked_positions) * 10
draw_window(win, grid, score)
draw_next_shape(next_piece, win)
pygame.display.update()
if check_lost(locked_positions):
run = False
draw_text_middle("You Lost", 80, WHITE, win)
pygame.display.update()
pygame.time.delay(2000)
def main_menu():
run = True
while run:
win.fill(BLACK)
draw_text_middle('Press Any Key To Play', 60, WHITE, win)
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
if event.type == pygame.KEYDOWN:
main()
pygame.display.quit()
win = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('Tetris')
main_menu()
总结
通过上述步骤,你可以使用Python和Pygame制作一个基本的俄罗斯方块游戏。这个过程包括定义游戏参数、创建必要的类、处理游戏逻辑、绘制游戏界面以及实现主循环等。你可以在此基础上进行扩展和优化,以创建一个更为复杂和有趣的游戏。
相关问答FAQs:
如何使用Python开发一个简单的俄罗斯方块游戏?
开发一个俄罗斯方块游戏可以通过使用Pygame库来实现。Pygame是一个适合初学者的游戏开发库,支持2D游戏制作。首先,你需要安装Pygame,然后通过创建游戏窗口、定义方块形状、处理用户输入以及添加游戏逻辑来逐步构建游戏。可以参考网上的教程和示例代码,帮助你更快地入门。
在Python中实现俄罗斯方块时,如何处理方块的旋转和移动?
处理方块的旋转和移动是俄罗斯方块的核心逻辑。你可以通过定义每种方块的不同形状以及它们的旋转状态来实现。通过捕获键盘事件,可以允许玩家使用方向键来移动方块。旋转可以通过改变方块的坐标数组来实现。例如,在每次按下“上”键时,更新方块的形状数组以实现旋转效果。
如何在Python的俄罗斯方块游戏中实现得分系统?
得分系统通常是游戏体验的重要部分。为了实现得分系统,你可以在每次消除一行方块时增加分数。可以维护一个分数变量,随着玩家的每一次成功消除行数而增加。还可以考虑为连续消除多行的情况设置额外的奖励分数,以激励玩家进行更高难度的操作。
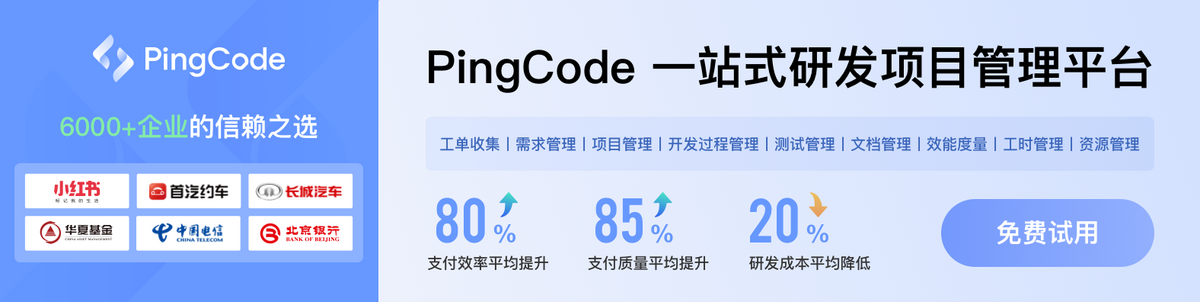