Python可以通过编写脚本来创建一个工资计算器,具体步骤包括收集用户输入、计算工资、处理税收和扣款、输出结果。其中,最重要的一点是确保输入数据的准确性,以便程序能够正确计算出员工的工资。下面将详细介绍如何在Python中创建一个工资计算器。
一、收集用户输入
在计算工资之前,需要收集一些基本信息,例如工作时间、时薪或月薪、税率、其他扣款等。可以使用Python的input()
函数来获取这些信息。
hours_worked = float(input("请输入工作小时数: "))
hourly_rate = float(input("请输入小时工资率: "))
tax_rate = float(input("请输入税率(例如20代表20%): ")) / 100
other_deductions = float(input("请输入其他扣款: "))
二、计算总工资
总工资的计算取决于工资的类型。对于按小时计算的员工,总工资等于工作时间乘以小时工资率;对于按月计算的员工,总工资等于月工资。
gross_pay = hours_worked * hourly_rate
print(f"总工资为: {gross_pay}")
三、处理税收和扣款
在计算出总工资后,需要扣除税款和其他扣款。税款可以通过总工资乘以税率计算得出。
tax_deduction = gross_pay * tax_rate
net_pay = gross_pay - tax_deduction - other_deductions
print(f"税后工资为: {net_pay}")
四、输出结果
最后,将计算结果输出给用户。可以格式化输出,使信息更易读。
print(f"总工资: {gross_pay:.2f}")
print(f"税款: {tax_deduction:.2f}")
print(f"其他扣款: {other_deductions:.2f}")
print(f"税后工资: {net_pay:.2f}")
五、完整代码示例
def get_user_input():
hours_worked = float(input("请输入工作小时数: "))
hourly_rate = float(input("请输入小时工资率: "))
tax_rate = float(input("请输入税率(例如20代表20%): ")) / 100
other_deductions = float(input("请输入其他扣款: "))
return hours_worked, hourly_rate, tax_rate, other_deductions
def calculate_gross_pay(hours_worked, hourly_rate):
return hours_worked * hourly_rate
def calculate_deductions(gross_pay, tax_rate, other_deductions):
tax_deduction = gross_pay * tax_rate
total_deductions = tax_deduction + other_deductions
return tax_deduction, total_deductions
def calculate_net_pay(gross_pay, total_deductions):
return gross_pay - total_deductions
def display_pay_information(gross_pay, tax_deduction, other_deductions, net_pay):
print(f"总工资: {gross_pay:.2f}")
print(f"税款: {tax_deduction:.2f}")
print(f"其他扣款: {other_deductions:.2f}")
print(f"税后工资: {net_pay:.2f}")
def main():
hours_worked, hourly_rate, tax_rate, other_deductions = get_user_input()
gross_pay = calculate_gross_pay(hours_worked, hourly_rate)
tax_deduction, total_deductions = calculate_deductions(gross_pay, tax_rate, other_deductions)
net_pay = calculate_net_pay(gross_pay, total_deductions)
display_pay_information(gross_pay, tax_deduction, other_deductions, net_pay)
if __name__ == "__main__":
main()
六、添加更多功能
为了让工资计算器更实用,可以考虑添加更多功能。例如:
- 处理加班费:如果员工的工作时间超过某个阈值(如40小时),可以计算加班费。
- 支持多种税率:根据员工的收入级别,采用不同的税率。
- 处理奖金和津贴:包括年度奖金、全勤奖等。
def calculate_overtime_pay(hours_worked, hourly_rate):
overtime_rate = 1.5 * hourly_rate
regular_hours = 40
if hours_worked > regular_hours:
overtime_hours = hours_worked - regular_hours
overtime_pay = overtime_hours * overtime_rate
return overtime_pay
return 0
def main():
hours_worked, hourly_rate, tax_rate, other_deductions = get_user_input()
gross_pay = calculate_gross_pay(hours_worked, hourly_rate)
overtime_pay = calculate_overtime_pay(hours_worked, hourly_rate)
total_gross_pay = gross_pay + overtime_pay
tax_deduction, total_deductions = calculate_deductions(total_gross_pay, tax_rate, other_deductions)
net_pay = calculate_net_pay(total_gross_pay, total_deductions)
display_pay_information(total_gross_pay, tax_deduction, other_deductions, net_pay)
if __name__ == "__main__":
main()
七、测试和调试
在开发完成后,务必进行充分的测试和调试,以确保工资计算器的准确性。可以使用不同的输入数据进行测试,查看输出结果是否符合预期。
八、用户界面
如果希望提供更好的用户体验,可以使用图形用户界面(GUI)库,例如Tkinter或PyQt,来创建一个更友好的界面。
import tkinter as tk
def calculate_pay():
hours_worked = float(hours_entry.get())
hourly_rate = float(rate_entry.get())
tax_rate = float(tax_entry.get()) / 100
other_deductions = float(deduction_entry.get())
gross_pay = hours_worked * hourly_rate
tax_deduction = gross_pay * tax_rate
net_pay = gross_pay - tax_deduction - other_deductions
result_var.set(f"总工资: {gross_pay:.2f}\n税款: {tax_deduction:.2f}\n其他扣款: {other_deductions:.2f}\n税后工资: {net_pay:.2f}")
root = tk.Tk()
root.title("工资计算器")
tk.Label(root, text="工作小时数:").grid(row=0, column=0)
tk.Label(root, text="小时工资率:").grid(row=1, column=0)
tk.Label(root, text="税率(%):").grid(row=2, column=0)
tk.Label(root, text="其他扣款:").grid(row=3, column=0)
hours_entry = tk.Entry(root)
rate_entry = tk.Entry(root)
tax_entry = tk.Entry(root)
deduction_entry = tk.Entry(root)
hours_entry.grid(row=0, column=1)
rate_entry.grid(row=1, column=1)
tax_entry.grid(row=2, column=1)
deduction_entry.grid(row=3, column=1)
tk.Button(root, text="计算", command=calculate_pay).grid(row=4, column=0, columnspan=2)
result_var = tk.StringVar()
tk.Label(root, textvariable=result_var).grid(row=5, column=0, columnspan=2)
root.mainloop()
九、持续改进
工资计算器的功能可以不断扩展和改进。例如,可以添加对不同货币的支持、生成工资单、保存和导出数据等功能。通过不断的优化和改进,使其更符合实际工作中的需求。
总结来说,使用Python创建一个工资计算器不仅能够帮助我们快速准确地计算工资,还可以通过不断扩展功能,提升计算器的使用体验和实用性。关键在于确保输入数据的准确性,处理好税收和扣款,并提供友好的用户界面。
相关问答FAQs:
如何使用Python创建一个简单的工资计算器?
要创建一个简单的工资计算器,可以使用Python的基本输入输出功能。首先,您需要收集员工的基本信息,如小时工资、工作时间等。接着,您可以通过简单的数学运算计算出总工资,并考虑加班费、税收等因素。可以利用函数来封装计算逻辑,使代码更整洁和可复用。
有哪些Python库可以帮助我构建工资计算器?
在构建工资计算器时,可以考虑使用一些Python库来提高效率。例如,Pandas库能够帮助您处理和分析数据,NumPy库可以用于数学计算。若需创建图形用户界面(GUI),Tkinter库是一个不错的选择,它可以使程序更加用户友好。
如何在工资计算器中实现税率和扣款的计算?
在工资计算器中实现税率和扣款的计算,可以通过设置不同的税率变量来处理。根据员工的工资水平,您可以应用不同的税率。计算完税前工资后,再进行扣款的计算。可以将这些逻辑封装在函数中,以便随时调用和修改。确保清晰地向用户解释各项扣款和税费的计算方法,以增强透明度。
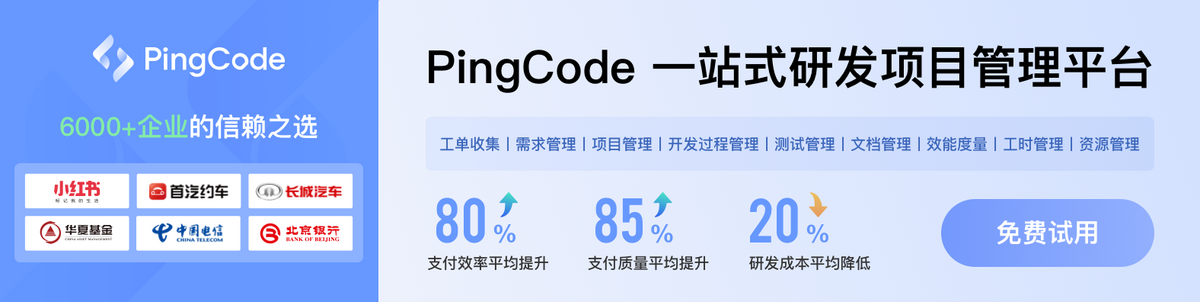