Python爬取软件数据库的基本方法包括:使用网络爬虫技术获取网页数据、利用API接口访问数据、直接连接数据库获取数据。网络爬虫、API接口、数据库连接是主要的方法。我们将详细介绍如何使用Python通过这三种主要方式爬取软件数据库。
一、网络爬虫
网络爬虫是通过模拟浏览器行为访问网页并提取其中的数据。Python中常用的爬虫库有requests
和BeautifulSoup
。
1、使用requests库获取网页内容
requests
库可以发送HTTP请求获取网页内容。以下是一个简单的示例代码:
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
print(html_content)
2、使用BeautifulSoup解析网页内容
BeautifulSoup
库可以解析HTML文档并提取数据。以下是一个示例代码:
from bs4 import BeautifulSoup
html_content = '''
<html>
<head><title>Example</title></head>
<body><p class="title"><b>Example Page</b></p></body>
</html>
'''
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.string
print(title)
3、结合requests和BeautifulSoup爬取数据
将requests
和BeautifulSoup
结合起来,可以实现从网页中提取数据的功能。以下是一个完整的示例代码:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
假设我们要提取所有的标题
titles = soup.find_all('h1')
for title in titles:
print(title.get_text())
二、API接口
许多软件和服务提供API接口,允许开发者通过编程方式访问数据。Python中常用的库有requests
和json
。
1、使用requests库访问API接口
requests
库可以发送HTTP请求,访问API接口。以下是一个简单的示例代码:
import requests
api_url = 'https://api.example.com/data'
response = requests.get(api_url)
data = response.json()
print(data)
2、处理API返回的数据
API返回的数据通常是JSON格式,可以使用json
库解析。以下是一个示例代码:
import requests
import json
api_url = 'https://api.example.com/data'
response = requests.get(api_url)
data = response.json()
假设我们要提取特定字段的数据
for item in data['items']:
print(item['name'])
3、使用API认证
有些API需要认证才能访问,可以使用API密钥或OAuth认证。以下是一个使用API密钥的示例代码:
import requests
api_url = 'https://api.example.com/data'
api_key = 'your_api_key'
headers = {'Authorization': f'Bearer {api_key}'}
response = requests.get(api_url, headers=headers)
data = response.json()
print(data)
三、数据库连接
Python中常用的数据库连接库有sqlite3
、pymysql
、psycopg2
等。
1、连接SQLite数据库
sqlite3
库可以连接SQLite数据库并执行SQL查询。以下是一个简单的示例代码:
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
2、连接MySQL数据库
pymysql
库可以连接MySQL数据库并执行SQL查询。以下是一个简单的示例代码:
import pymysql
conn = pymysql.connect(
host='localhost',
user='user',
password='password',
database='example_db'
)
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
3、连接PostgreSQL数据库
psycopg2
库可以连接PostgreSQL数据库并执行SQL查询。以下是一个简单的示例代码:
import psycopg2
conn = psycopg2.connect(
dbname='example_db',
user='user',
password='password',
host='localhost'
)
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
四、综合应用
在实际应用中,可以将网络爬虫、API接口和数据库连接结合起来,实现复杂的数据爬取和处理任务。
1、从网页爬取数据并存入数据库
以下示例代码展示了如何从网页爬取数据并存入SQLite数据库:
import requests
from bs4 import BeautifulSoup
import sqlite3
爬取数据
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('h1')
存入数据库
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('CREATE TABLE IF NOT EXISTS titles (title TEXT)')
for title in titles:
cursor.execute('INSERT INTO titles (title) VALUES (?)', (title.get_text(),))
conn.commit()
conn.close()
2、从API获取数据并存入数据库
以下示例代码展示了如何从API获取数据并存入MySQL数据库:
import requests
import pymysql
获取数据
api_url = 'https://api.example.com/data'
response = requests.get(api_url)
data = response.json()
存入数据库
conn = pymysql.connect(
host='localhost',
user='user',
password='password',
database='example_db'
)
cursor = conn.cursor()
cursor.execute('CREATE TABLE IF NOT EXISTS items (name TEXT)')
for item in data['items']:
cursor.execute('INSERT INTO items (name) VALUES (%s)', (item['name'],))
conn.commit()
conn.close()
3、从数据库读取数据并进行分析
以下示例代码展示了如何从PostgreSQL数据库读取数据并进行简单的分析:
import psycopg2
连接数据库
conn = psycopg2.connect(
dbname='example_db',
user='user',
password='password',
host='localhost'
)
cursor = conn.cursor()
读取数据
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
简单分析:统计用户数量
user_count = len(rows)
print(f'Total users: {user_count}')
conn.close()
通过以上方法,您可以使用Python爬取软件数据库中的数据,进行数据分析和处理。无论是通过网络爬虫获取网页数据、利用API接口访问数据,还是直接连接数据库获取数据,都能够满足不同场景下的数据爬取需求。希望本文提供的示例代码和方法能够帮助您更好地理解和应用Python进行数据爬取。
相关问答FAQs:
如何使用Python连接到软件的数据库进行爬取?
在使用Python爬取软件数据库之前,您需要确定数据库的类型,例如MySQL、PostgreSQL或SQLite。接着,使用相应的数据库连接库(如mysql-connector-python
、psycopg2
或sqlite3
)来建立连接。确保您具备数据库的访问权限,并了解相关的表结构及数据类型,这样才能有效地提取所需的数据。
在爬取数据库时如何处理数据的格式和编码问题?
数据在存储时可能会使用不同的编码格式,常见的有UTF-8和ISO-8859-1。在使用Python获取数据后,可以通过调用.encode()
和.decode()
方法来处理字符串的编码问题。此外,使用Pandas库可以方便地读取和处理数据,确保数据的格式符合您的需求。
如何确保爬取软件数据库时的数据安全性与合规性?
在进行数据爬取时,遵循相关法律法规非常重要,特别是涉及用户隐私和数据保护的方面。获取数据之前,确保您具有必要的权限和合法的使用依据。此外,使用安全的连接方式(如SSL/TLS)以及对敏感数据进行加密处理,可以有效提高数据传输的安全性,保护用户信息。
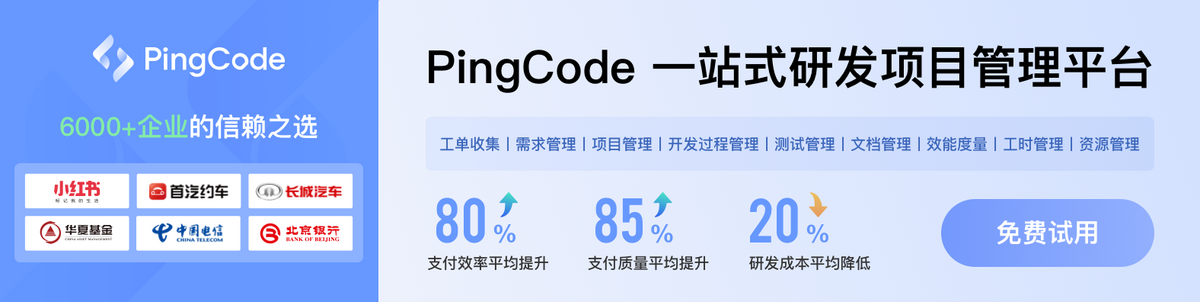