在Python中判断字符串类型的方法有很多种,使用内置的isinstance()
函数、使用type()
函数、使用正则表达式,其中最常用和推荐的方法是使用内置的isinstance()
函数,因为它最为简洁且高效。使用isinstance()
函数可以方便地判断一个变量是否为字符串类型,方法是将变量和str
类作为参数传入isinstance()
函数中,如果变量是字符串类型,则返回True
,否则返回False
。
一、使用 isinstance()
函数
isinstance()
是 Python 内置的一个函数,用于判断一个对象是否是某个类型。它的用法非常简单,可以判断一个变量是否为字符串类型。示例如下:
variable = "Hello, World!"
if isinstance(variable, str):
print("The variable is a string.")
else:
print("The variable is not a string.")
这个方法的好处在于,它不仅能判断字符串,还能判断其他数据类型。可以同时检查多个类型,只需要将类型放在一个元组中即可。
二、使用 type()
函数
type()
函数返回对象的类型,可以通过比较返回值与 str
类来判断字符串类型。示例如下:
variable = "Hello, World!"
if type(variable) == str:
print("The variable is a string.")
else:
print("The variable is not a string.")
虽然这种方法也能判断字符串类型,但不如 isinstance()
函数直观和灵活。type()
只适用于检查单个类型,不支持多类型检查。
三、使用正则表达式
在某些特定情况下,可以使用正则表达式来判断字符串类型,特别是当需要判断字符串是否符合特定格式时。这种方法适合对字符串内容进行模式匹配,而不是单纯判断数据类型。
import re
def is_string(variable):
if isinstance(variable, str) and re.match("^[a-zA-Z0-9_]*$", variable):
return True
return False
variable = "HelloWorld123"
if is_string(variable):
print("The variable is a string and matches the pattern.")
else:
print("The variable is not a string or does not match the pattern.")
这种方法对字符串内容的要求更为严格,因此不适合用于一般的字符串类型判断。
四、字符串类型判断的应用场景
1、输入验证
在开发过程中,经常需要对用户输入的数据进行类型验证,以确保数据的合法性和正确性。通过判断字符串类型,可以有效防止错误输入导致的程序崩溃或异常。
def input_is_string(user_input):
return isinstance(user_input, str)
user_input = input("Please enter a string: ")
if input_is_string(user_input):
print("Valid input!")
else:
print("Invalid input! Please enter a string.")
2、数据处理
在处理数据时,有时需要根据数据类型采取不同的处理方式。判断字符串类型可以帮助我们对不同类型的数据进行分类处理,提高程序的健壮性和可维护性。
def process_data(data):
if isinstance(data, str):
print(f"Processing string data: {data}")
else:
print("Data is not a string, skipping processing.")
data_list = ["Hello", 123, "World", 456]
for data in data_list:
process_data(data)
3、函数参数类型检查
在编写函数时,可以对参数类型进行检查,确保传入的参数符合预期的类型,从而避免函数执行过程中出现类型错误。
def concatenate_strings(str1, str2):
if isinstance(str1, str) and isinstance(str2, str):
return str1 + str2
else:
raise TypeError("Both arguments must be strings.")
try:
result = concatenate_strings("Hello", "World")
print(result)
except TypeError as e:
print(e)
五、判断字符串类型的注意事项
1、兼容 Python 2 和 Python 3
在一些项目中,可能需要兼容 Python 2 和 Python 3 两个版本。由于这两个版本在字符串类型上存在差异,特别是在 Python 2 中,str
和 unicode
是不同的类型,而在 Python 3 中,str
类型代表 Unicode 字符串。因此,在编写代码时,需要考虑到这种差异。
import sys
if sys.version_info[0] < 3:
# Python 2.x
basestring = basestring
else:
# Python 3.x
basestring = str
def is_string(variable):
return isinstance(variable, basestring)
variable = "Hello, World!"
if is_string(variable):
print("The variable is a string.")
else:
print("The variable is not a string.")
2、避免类型误判
在判断字符串类型时,需要确保判断的准确性,避免误判。例如,在某些情况下,可能会将数字或其他类型的数据错误地识别为字符串。因此,在编写代码时,需要充分考虑各种可能的情况,确保判断逻辑的严谨性。
def is_string(variable):
return isinstance(variable, str) and not isinstance(variable, (int, float, complex))
variable = "123"
if is_string(variable):
print("The variable is a string.")
else:
print("The variable is not a string.")
六、总结
通过本文的介绍,我们了解了在 Python 中判断字符串类型的多种方法,包括使用 isinstance()
函数、type()
函数和正则表达式等。其中,最推荐的方法是使用 isinstance()
函数,因为它最为简洁且高效。我们还讨论了判断字符串类型的应用场景和注意事项,包括输入验证、数据处理、函数参数类型检查等。希望这些内容能够帮助你在实际开发中更好地判断和处理字符串类型。
相关问答FAQs:
在Python中如何确认一个变量是否为字符串?
要确认一个变量是否为字符串,可以使用内置的isinstance()
函数。例如,如果你有一个变量my_var
,可以使用isinstance(my_var, str)
来判断。如果返回True
,则my_var
是字符串类型,反之则不是。
Python中字符串和其他数据类型的区别是什么?
字符串在Python中是由字符组成的序列,通常用于表示文本数据。与整数、浮点数或列表等其他数据类型相比,字符串具有不同的操作和方法。例如,字符串可以使用切片、连接和格式化等操作,而数字则不支持这些操作。
如何在Python中处理字符串类型的异常情况?
在处理字符串时,可能会遇到一些常见的异常情况,例如输入为None
或其他非字符串类型。可以通过条件判断或try-except
语句来捕获和处理这些异常。例如,使用if my_var is not None and isinstance(my_var, str)
来确保处理的是有效的字符串,避免程序崩溃。
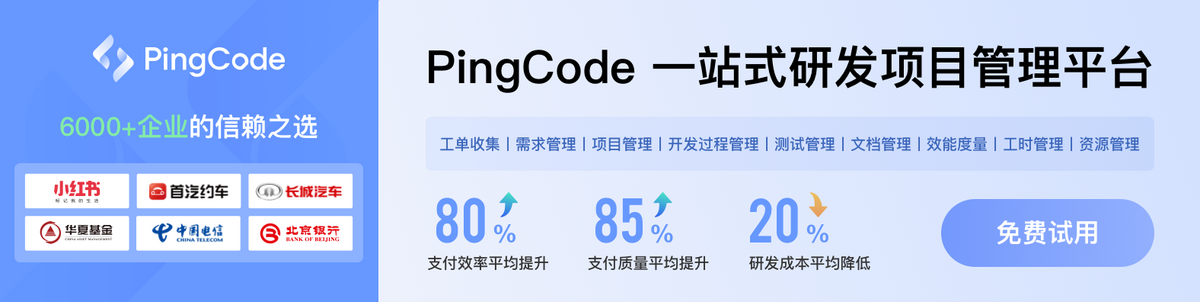