要批量复制多个文件夹,您可以使用以下步骤:导入所需的模块、定义源文件夹和目标文件夹、获取所有文件夹的列表、使用循环复制文件夹。
在Python中,您可以使用shutil模块中的copytree函数来实现这一点。下面是一个详细的示例。
一、导入所需的模块
在Python中,您需要使用os和shutil模块来处理文件和文件夹的操作。os模块提供了与操作系统交互的功能,而shutil模块则提供了高级的文件操作功能,包括复制文件和文件夹。
import os
import shutil
二、定义源文件夹和目标文件夹
首先,您需要定义源文件夹和目标文件夹的路径。源文件夹是包含您要复制的文件夹的目录,目标文件夹是您要将这些文件夹复制到的目录。
source_directory = '/path/to/source_directory'
destination_directory = '/path/to/destination_directory'
三、获取所有文件夹的列表
接下来,您需要获取源文件夹中所有子文件夹的列表。可以使用os.listdir()函数来获取源文件夹中的所有项目,并使用os.path.isdir()函数来过滤出文件夹。
folders = [f for f in os.listdir(source_directory) if os.path.isdir(os.path.join(source_directory, f))]
四、使用循环复制文件夹
最后,您可以使用一个循环来遍历文件夹列表,并使用shutil.copytree()函数将每个文件夹复制到目标文件夹。
for folder in folders:
src_folder = os.path.join(source_directory, folder)
dest_folder = os.path.join(destination_directory, folder)
shutil.copytree(src_folder, dest_folder)
完整代码示例
下面是完整的代码示例,演示如何批量复制多个文件夹:
import os
import shutil
定义源文件夹和目标文件夹的路径
source_directory = '/path/to/source_directory'
destination_directory = '/path/to/destination_directory'
获取源文件夹中所有子文件夹的列表
folders = [f for f in os.listdir(source_directory) if os.path.isdir(os.path.join(source_directory, f))]
遍历文件夹列表并复制每个文件夹到目标文件夹
for folder in folders:
src_folder = os.path.join(source_directory, folder)
dest_folder = os.path.join(destination_directory, folder)
shutil.copytree(src_folder, dest_folder)
五、处理文件夹重名问题
在实际操作中,可能会遇到目标文件夹中已经存在同名文件夹的情况。为了解决这个问题,您可以在复制文件夹之前检查目标文件夹是否存在,并为其生成一个唯一的名称。
import os
import shutil
def get_unique_name(destination_folder, folder_name):
counter = 1
new_folder_name = folder_name
while os.path.exists(os.path.join(destination_folder, new_folder_name)):
new_folder_name = f"{folder_name}_{counter}"
counter += 1
return new_folder_name
source_directory = '/path/to/source_directory'
destination_directory = '/path/to/destination_directory'
folders = [f for f in os.listdir(source_directory) if os.path.isdir(os.path.join(source_directory, f))]
for folder in folders:
src_folder = os.path.join(source_directory, folder)
unique_folder_name = get_unique_name(destination_directory, folder)
dest_folder = os.path.join(destination_directory, unique_folder_name)
shutil.copytree(src_folder, dest_folder)
通过这种方式,您可以确保在目标文件夹中不会出现重名的文件夹。
六、处理大文件和文件夹
如果需要复制的文件夹包含大量文件,或者文件夹本身非常大,使用shutil.copytree()函数可能会非常耗时。为了提高性能,您可以使用多线程或多进程来并行复制文件夹。
使用多线程
下面是一个示例,演示如何使用concurrent.futures.ThreadPoolExecutor来并行复制文件夹:
import os
import shutil
from concurrent.futures import ThreadPoolExecutor
def copy_folder(src_folder, dest_folder):
shutil.copytree(src_folder, dest_folder)
def get_unique_name(destination_folder, folder_name):
counter = 1
new_folder_name = folder_name
while os.path.exists(os.path.join(destination_folder, new_folder_name)):
new_folder_name = f"{folder_name}_{counter}"
counter += 1
return new_folder_name
source_directory = '/path/to/source_directory'
destination_directory = '/path/to/destination_directory'
folders = [f for f in os.listdir(source_directory) if os.path.isdir(os.path.join(source_directory, f))]
with ThreadPoolExecutor() as executor:
for folder in folders:
src_folder = os.path.join(source_directory, folder)
unique_folder_name = get_unique_name(destination_directory, folder)
dest_folder = os.path.join(destination_directory, unique_folder_name)
executor.submit(copy_folder, src_folder, dest_folder)
使用多进程
同样,您也可以使用concurrent.futures.ProcessPoolExecutor来并行复制文件夹:
import os
import shutil
from concurrent.futures import ProcessPoolExecutor
def copy_folder(src_folder, dest_folder):
shutil.copytree(src_folder, dest_folder)
def get_unique_name(destination_folder, folder_name):
counter = 1
new_folder_name = folder_name
while os.path.exists(os.path.join(destination_folder, new_folder_name)):
new_folder_name = f"{folder_name}_{counter}"
counter += 1
return new_folder_name
source_directory = '/path/to/source_directory'
destination_directory = '/path/to/destination_directory'
folders = [f for f in os.listdir(source_directory) if os.path.isdir(os.path.join(source_directory, f))]
with ProcessPoolExecutor() as executor:
for folder in folders:
src_folder = os.path.join(source_directory, folder)
unique_folder_name = get_unique_name(destination_directory, folder)
dest_folder = os.path.join(destination_directory, unique_folder_name)
executor.submit(copy_folder, src_folder, dest_folder)
通过使用多线程或多进程,您可以显著提高批量复制多个文件夹的性能,特别是在处理大文件和文件夹时。
七、处理错误和异常
在批量复制文件夹的过程中,可能会遇到各种错误和异常,例如权限问题、磁盘空间不足等。为了确保程序能够稳健地运行,您需要添加错误处理机制。
import os
import shutil
from concurrent.futures import ThreadPoolExecutor
def copy_folder(src_folder, dest_folder):
try:
shutil.copytree(src_folder, dest_folder)
except Exception as e:
print(f"Error copying {src_folder} to {dest_folder}: {e}")
def get_unique_name(destination_folder, folder_name):
counter = 1
new_folder_name = folder_name
while os.path.exists(os.path.join(destination_folder, new_folder_name)):
new_folder_name = f"{folder_name}_{counter}"
counter += 1
return new_folder_name
source_directory = '/path/to/source_directory'
destination_directory = '/path/to/destination_directory'
folders = [f for f in os.listdir(source_directory) if os.path.isdir(os.path.join(source_directory, f))]
with ThreadPoolExecutor() as executor:
for folder in folders:
src_folder = os.path.join(source_directory, folder)
unique_folder_name = get_unique_name(destination_directory, folder)
dest_folder = os.path.join(destination_directory, unique_folder_name)
executor.submit(copy_folder, src_folder, dest_folder)
通过添加错误处理机制,您可以确保在遇到错误时程序不会崩溃,并且可以继续复制其他文件夹。
八、日志记录
为了更好地跟踪批量复制文件夹的过程,您可以添加日志记录功能。Python的logging模块提供了强大的日志记录功能。
import os
import shutil
import logging
from concurrent.futures import ThreadPoolExecutor
def copy_folder(src_folder, dest_folder):
try:
shutil.copytree(src_folder, dest_folder)
logging.info(f"Successfully copied {src_folder} to {dest_folder}")
except Exception as e:
logging.error(f"Error copying {src_folder} to {dest_folder}: {e}")
def get_unique_name(destination_folder, folder_name):
counter = 1
new_folder_name = folder_name
while os.path.exists(os.path.join(destination_folder, new_folder_name)):
new_folder_name = f"{folder_name}_{counter}"
counter += 1
return new_folder_name
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
source_directory = '/path/to/source_directory'
destination_directory = '/path/to/destination_directory'
folders = [f for f in os.listdir(source_directory) if os.path.isdir(os.path.join(source_directory, f))]
with ThreadPoolExecutor() as executor:
for folder in folders:
src_folder = os.path.join(source_directory, folder)
unique_folder_name = get_unique_name(destination_directory, folder)
dest_folder = os.path.join(destination_directory, unique_folder_name)
executor.submit(copy_folder, src_folder, dest_folder)
通过添加日志记录功能,您可以更好地监控和调试批量复制文件夹的过程。
九、总结
通过上述步骤,您可以使用Python批量复制多个文件夹。首先,您需要导入所需的模块,并定义源文件夹和目标文件夹。然后,您可以获取所有文件夹的列表,并使用循环来复制每个文件夹。为了处理文件夹重名问题,您可以在复制文件夹之前生成一个唯一的名称。对于大文件和文件夹,您可以使用多线程或多进程来提高性能。此外,您还可以添加错误处理机制和日志记录功能,以确保程序的稳健性和可调试性。
通过这些方法,您可以轻松地使用Python批量复制多个文件夹,并处理实际操作中可能遇到的各种问题。
相关问答FAQs:
如何使用Python脚本批量复制文件夹?
要批量复制多个文件夹,您可以使用Python的shutil
模块。这个模块提供了一个简单的接口来处理文件和文件夹操作。您可以编写一个脚本,遍历要复制的文件夹列表,然后使用shutil.copytree()
函数来实现复制。确保在复制时指定目标路径,避免覆盖已有的文件夹。
在复制文件夹时,如何处理文件夹中存在的重复文件?
在使用shutil.copytree()
进行复制时,如果目标文件夹已经存在,会抛出一个FileExistsError
。为了避免这个问题,可以在复制前检查目标文件夹是否存在,若存在,可以选择删除现有文件夹或重命名新文件夹。您也可以使用dirs_exist_ok=True
参数,这样可以在目标文件夹存在的情况下进行合并。
是否可以使用Python的其他库来复制文件夹?
除了shutil
,还可以使用os
和pathlib
库来实现文件夹的复制。例如,您可以使用os.walk()
函数遍历文件夹,并结合os.makedirs()
和shutil.copy2()
来手动复制每个文件。pathlib
也提供了更现代化的方式来处理文件路径和文件操作,尤其适合处理复杂的文件系统任务。
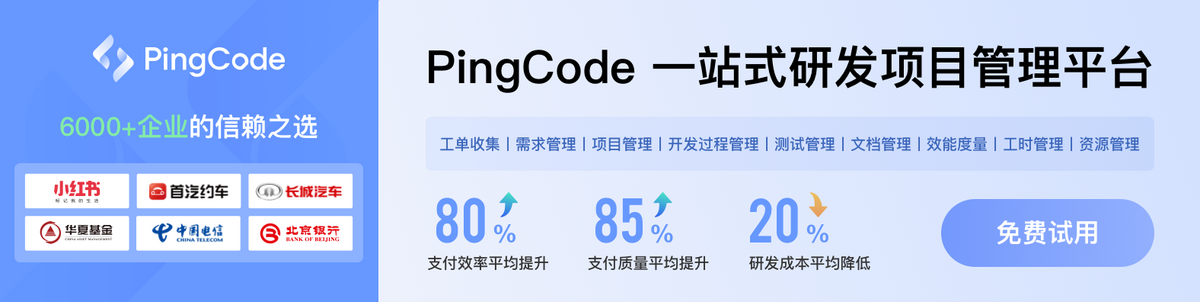