如何利用Python做一个象棋
利用Python制作一个象棋游戏可以通过以下几个关键步骤:选择适当的图形库、设计棋盘和棋子、实现象棋规则、处理用户交互、实现AI对手。选择适当的图形库、设计棋盘和棋子、实现象棋规则、处理用户交互、实现AI对手。本文将详细介绍如何使用Python和Pygame库来完成一个基本的象棋游戏,并对其中的“实现象棋规则”进行详细描述。
一、选择适当的图形库
为了实现象棋的图形界面,我们需要选择一个图形库。Pygame是一个非常流行的Python库,它提供了简单的界面来处理图形和用户输入,非常适合制作2D游戏。你可以通过以下命令来安装Pygame:
pip install pygame
二、设计棋盘和棋子
在设计棋盘时,我们需要考虑棋盘的大小和每个格子的大小。象棋棋盘是一个9×10的矩形,我们可以用Pygame来绘制它。首先,我们需要定义一些全局变量,比如棋盘的宽度和高度、每个格子的大小等。
import pygame
棋盘的宽度和高度
WIDTH, HEIGHT = 900, 1000
ROWS, COLS = 10, 9
SQUARE_SIZE = WIDTH // COLS
定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
初始化Pygame
pygame.init()
WIN = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Chinese Chess")
接下来,我们需要绘制棋盘和棋子。我们可以创建一个函数来绘制棋盘,并在主循环中调用这个函数。
def draw_board(win):
win.fill(WHITE)
for row in range(ROWS):
for col in range(COLS):
pygame.draw.rect(win, BLACK, (col * SQUARE_SIZE, row * SQUARE_SIZE, SQUARE_SIZE, SQUARE_SIZE), 1)
主循环
run = True
while run:
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
draw_board(WIN)
pygame.display.update()
pygame.quit()
三、实现象棋规则
实现象棋规则是制作象棋游戏的核心部分。象棋的规则相对复杂,包括各种棋子的移动规则、吃子规则、将军和将死规则等。我们可以通过面向对象编程的方式来实现这些规则。
首先,我们需要定义一个基类Piece
,然后为每种棋子创建子类。每个子类将实现其特有的移动规则。
class Piece:
def __init__(self, row, col, color):
self.row = row
self.col = col
self.color = color
def move(self, row, col):
self.row = row
self.col = col
def valid_moves(self, board):
pass
class Rook(Piece):
def valid_moves(self, board):
moves = []
# 横向和纵向移动逻辑
return moves
class Knight(Piece):
def valid_moves(self, board):
moves = []
# 马走日逻辑
return moves
其他棋子类:Bishop, Queen, King, Pawn
然后,我们需要创建一个Board
类来管理棋盘和棋子的状态。
class Board:
def __init__(self):
self.board = [[None for _ in range(COLS)] for _ in range(ROWS)]
self.create_pieces()
def create_pieces(self):
# 初始化棋子
pass
def move_piece(self, piece, row, col):
self.board[piece.row][piece.col] = None
self.board[row][col] = piece
piece.move(row, col)
def get_piece(self, row, col):
return self.board[row][col]
def draw(self, win):
draw_board(win)
for row in range(ROWS):
for col in range(COLS):
piece = self.board[row][col]
if piece:
piece.draw(win)
四、处理用户交互
为了让玩家能够与游戏进行交互,我们需要处理鼠标点击事件。我们可以在主循环中检查鼠标点击事件,并根据点击的位置来选择和移动棋子。
selected_piece = None
board = Board()
def get_row_col_from_mouse(pos):
x, y = pos
row = y // SQUARE_SIZE
col = x // SQUARE_SIZE
return row, col
while run:
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
if event.type == pygame.MOUSEBUTTONDOWN:
pos = pygame.mouse.get_pos()
row, col = get_row_col_from_mouse(pos)
if selected_piece:
board.move_piece(selected_piece, row, col)
selected_piece = None
else:
selected_piece = board.get_piece(row, col)
board.draw(WIN)
pygame.display.update()
五、实现AI对手
为了增加游戏的挑战性,我们可以为游戏添加一个AI对手。AI可以使用简单的搜索算法,如迷你最大算法(Minimax)和alpha-beta剪枝(Alpha-Beta Pruning),来决定最佳移动。
首先,我们需要创建一个AI
类,并实现一个用于计算最佳移动的方法。
class AI:
def __init__(self, board, color):
self.board = board
self.color = color
def minimax(self, depth, maximizing_player):
if depth == 0:
return self.evaluate()
if maximizing_player:
max_eval = float('-inf')
for move in self.get_all_moves(self.color):
self.make_move(move)
eval = self.minimax(depth-1, False)
self.undo_move(move)
max_eval = max(max_eval, eval)
return max_eval
else:
min_eval = float('inf')
for move in self.get_all_moves(self.get_opponent_color(self.color)):
self.make_move(move)
eval = self.minimax(depth-1, True)
self.undo_move(move)
min_eval = min(min_eval, eval)
return min_eval
def get_all_moves(self, color):
# 获取所有可能的移动
pass
def make_move(self, move):
# 执行移动
pass
def undo_move(self, move):
# 撤销移动
pass
def evaluate(self):
# 评估当前棋盘状态
pass
def get_opponent_color(self, color):
return 'black' if color == 'red' else 'red'
然后,我们可以在主循环中调用AI的方法来决定AI的移动。
ai = AI(board, 'black')
while run:
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
if event.type == pygame.MOUSEBUTTONDOWN:
pos = pygame.mouse.get_pos()
row, col = get_row_col_from_mouse(pos)
if selected_piece:
board.move_piece(selected_piece, row, col)
selected_piece = None
ai_move = ai.minimax(3, True)
board.move_piece(ai_move.piece, ai_move.row, ai_move.col)
else:
selected_piece = board.get_piece(row, col)
board.draw(WIN)
pygame.display.update()
通过上述步骤,我们可以使用Python和Pygame制作一个基本的象棋游戏。虽然这个游戏可能还不够完善,但它为我们提供了一个良好的起点。根据需要,我们可以进一步优化和扩展游戏功能,如添加更多的规则处理、更复杂的AI算法、更丰富的图形界面等。希望这篇文章对你有所帮助!
相关问答FAQs:
如何开始使用Python创建象棋游戏?
在创建象棋游戏之前,了解Python的基本语法和面向对象编程是非常重要的。可以通过学习模块和类来组织代码,确保游戏逻辑清晰。选择合适的图形库如Pygame或Tkinter,能够帮助你创建游戏界面。建议从简单的棋盘绘制开始,然后逐步添加棋子和游戏规则。
在象棋游戏中,如何实现棋子移动的逻辑?
实现棋子移动的逻辑需要定义每种棋子的移动规则,比如马的“日”字形、象的斜走等。这可以通过创建一个棋子类,并在类中定义一个方法来检查移动是否合法。此外,使用一个二维数组来表示棋盘状态,可以帮助你更新和验证棋子的移动。
如何处理象棋游戏中的用户输入?
用户输入在游戏中至关重要,通常可以通过键盘事件或鼠标点击来实现。在Pygame中,可以捕捉这些事件并根据用户的选择更新棋盘状态。确保在处理输入时添加有效性检查,以防止用户进行非法移动或其他不当操作。这将提高游戏的用户体验,使其更加流畅和有趣。
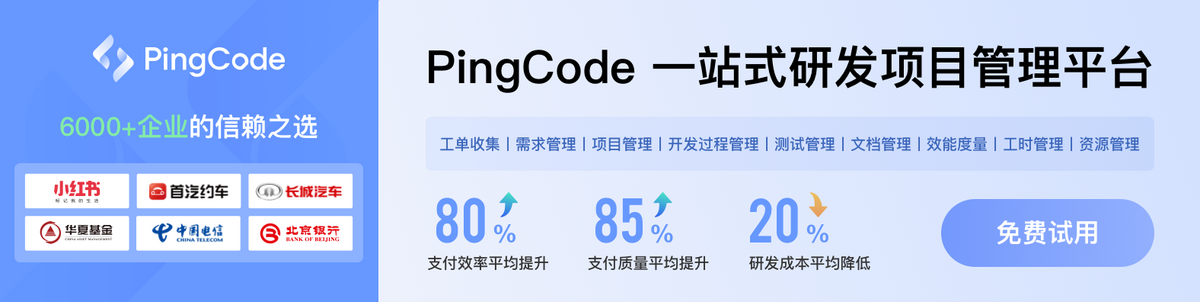