Python如何去除字符串中的空格、特定字符、子字符串
在Python中,去除字符串中的空格、特定字符或子字符串是一个常见的操作。使用strip()
方法、使用replace()
方法、使用正则表达式是实现这些操作的常用方法。下面我们将详细介绍这些方法及其使用场景。
一、使用strip()
方法
strip()
方法用于去除字符串两端的空格或指定字符,但不影响字符串中间的部分。它有两个变体:lstrip()
和rstrip()
,分别用于去除左端和右端的字符。
1.1 去除两端空格
text = " Hello, World! "
clean_text = text.strip()
print(clean_text) # Output: "Hello, World!"
1.2 去除特定字符
text = "###Hello, World!###"
clean_text = text.strip('#')
print(clean_text) # Output: "Hello, World!"
1.3 去除左端字符
text = "###Hello, World!###"
clean_text = text.lstrip('#')
print(clean_text) # Output: "Hello, World!###"
1.4 去除右端字符
text = "###Hello, World!###"
clean_text = text.rstrip('#')
print(clean_text) # Output: "###Hello, World!"
二、使用replace()
方法
replace()
方法用于替换字符串中的某些子字符串。它可以用来去除字符串中的特定字符或子字符串。
2.1 去除所有空格
text = "Hello, World!"
clean_text = text.replace(" ", "")
print(clean_text) # Output: "Hello,World!"
2.2 去除特定字符
text = "Hello, World!"
clean_text = text.replace(",", "")
print(clean_text) # Output: "Hello World!"
2.3 去除子字符串
text = "Hello, World!"
clean_text = text.replace("World", "")
print(clean_text) # Output: "Hello, !"
三、使用正则表达式
正则表达式(regular expressions)是处理字符串的强大工具,Python的re
模块提供了操作正则表达式的功能。
3.1 去除所有空格
import re
text = "Hello, World!"
clean_text = re.sub(r"\s+", "", text)
print(clean_text) # Output: "Hello,World!"
3.2 去除特定字符
import re
text = "Hello, World!"
clean_text = re.sub(r",", "", text)
print(clean_text) # Output: "Hello World!"
3.3 去除子字符串
import re
text = "Hello, World!"
clean_text = re.sub(r"World", "", text)
print(clean_text) # Output: "Hello, !"
四、综合应用
在实际应用中,可能会遇到需要综合使用以上方法的情况。下面是一些综合应用的实例。
4.1 去除字符串两端的空格和中间的特定字符
text = " ##Hello, World!## "
clean_text = text.strip().replace("#", "")
print(clean_text) # Output: "Hello, World!"
4.2 去除字符串中所有的数字和空格
import re
text = "Hello 123, World 456!"
clean_text = re.sub(r"\d+\s*", "", text)
print(clean_text) # Output: "Hello, World!"
4.3 去除字符串中的HTML标签
import re
text = "<p>Hello, <b>World</b>!</p>"
clean_text = re.sub(r"<.*?>", "", text)
print(clean_text) # Output: "Hello, World!"
五、性能比较
在处理大字符串或需要高效处理时,选择合适的方法是至关重要的。strip()
和replace()
方法在处理较小字符串时性能较好,而正则表达式在处理复杂模式匹配时更为强大,但可能会稍微慢一些。
5.1 性能测试
import time
Test data
text = " " * 1000 + "Hello, World!" + " " * 1000
strip() method
start_time = time.time()
clean_text = text.strip()
end_time = time.time()
print(f"strip() method took {end_time - start_time} seconds")
replace() method
start_time = time.time()
clean_text = text.replace(" ", "")
end_time = time.time()
print(f"replace() method took {end_time - start_time} seconds")
re.sub() method
import re
start_time = time.time()
clean_text = re.sub(r"\s+", "", text)
end_time = time.time()
print(f"re.sub() method took {end_time - start_time} seconds")
六、总结
去除字符串中的空格、特定字符或子字符串是Python编程中的常见任务,可以通过strip()
、replace()
和正则表达式等方法实现。选择合适的方法可以提高代码的可读性和执行效率。对于简单的操作,strip()
和replace()
方法非常合适;而对于复杂的字符串处理,正则表达式提供了更强大的功能。
在实际应用中,综合使用这些方法能够有效地解决各种字符串处理问题。希望这篇文章能帮助你更好地理解和应用这些技巧,提高你的Python编程技能。
相关问答FAQs:
如何在Python中高效地去除字符串中的特定字符?
在Python中,可以使用str.replace()
方法来去除特定字符。例如,如果想去除字符串中的某个字符,可以将其替换为空字符串。示例代码如下:
original_string = "Hello, World!"
modified_string = original_string.replace(",", "")
print(modified_string) # 输出: Hello World!
这种方法简洁明了,适用于去除单个字符或多个相同字符。
Python中是否有正则表达式来去除字符串中的字符?
是的,Python的re
模块提供了强大的正则表达式功能,可以用来更灵活地处理字符串。使用re.sub()
可以轻松去除特定字符。例如,要去除所有的非字母字符,可以这样写:
import re
original_string = "Hello, World! 123"
modified_string = re.sub(r'[^a-zA-Z]', '', original_string)
print(modified_string) # 输出: HelloWorld
这种方法适合需要复杂模式匹配的场景。
在Python中去除字符串中特定字符的常见误区有哪些?
许多人在去除字符串中的字符时,可能会误用str.strip()
方法。strip()
方法主要用于去除字符串两端的空白字符或指定字符,而不会影响字符串中间的字符。因此,如果希望去除字符串中间的某个字符,建议使用replace()
或re.sub()
等方法。
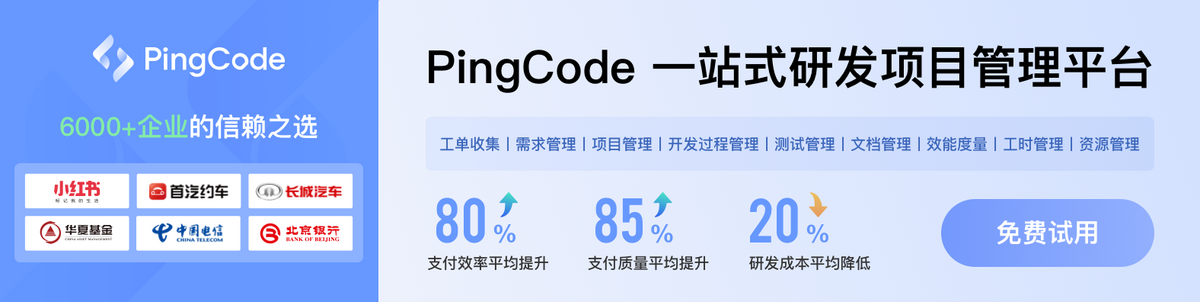