在Python中查看文件的编码格式有多种方法,包括使用chardet库、cchardet库、codecs库等。推荐使用chardet库、codecs库、cchardet库。 chardet库是一个广泛使用的第三方库,它能够自动检测文件的编码格式。codecs库是Python的内置库,它提供了对编码和解码的支持。cchardet库是chardet库的一个高效版本,性能更好。下面将详细介绍如何使用chardet库来查看文件的编码格式。
一、使用chardet库
chardet库是一个强大的工具,可以用来检测文件的编码格式。它支持多种编码格式,包括UTF-8、ISO-8859-1、GB2312等。使用chardet库非常简单,只需要几行代码即可完成。以下是具体步骤:
- 安装chardet库
pip install chardet
- 检测文件编码
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
return encoding
示例
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f"The encoding of the file is: {encoding}")
二、使用codecs库
codecs库是Python的内置库,它提供了对编码和解码的支持。虽然codecs库无法自动检测文件的编码格式,但它可以用来读取文件的BOM(Byte Order Mark)来推断文件的编码格式。以下是具体步骤:
- 读取文件的BOM
import codecs
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read(4)
if raw_data.startswith(codecs.BOM_UTF8):
return 'utf-8-sig'
elif raw_data.startswith(codecs.BOM_UTF16_LE):
return 'utf-16-le'
elif raw_data.startswith(codecs.BOM_UTF16_BE):
return 'utf-16-be'
elif raw_data.startswith(codecs.BOM_UTF32_LE):
return 'utf-32-le'
elif raw_data.startswith(codecs.BOM_UTF32_BE):
return 'utf-32-be'
else:
return 'unknown'
示例
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f"The encoding of the file is: {encoding}")
三、使用cchardet库
cchardet库是chardet库的一个高效版本,它的性能更好,适用于需要处理大量文件的情况。使用cchardet库的方式与chardet库类似。以下是具体步骤:
- 安装cchardet库
pip install cchardet
- 检测文件编码
import cchardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = cchardet.detect(raw_data)
encoding = result['encoding']
return encoding
示例
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f"The encoding of the file is: {encoding}")
四、总结
通过以上方法,可以方便地查看文件的编码格式。在实际应用中,可以根据具体需求选择合适的方法。对于性能要求较高的场合,推荐使用cchardet库;对于一般情况,chardet库已经足够;如果文件包含BOM,可以使用codecs库。无论选择哪种方法,都能有效地解决查看文件编码格式的问题。
相关问答FAQs:
如何确定一个文件的编码格式?
要确定文件的编码格式,可以使用Python的chardet
库。这个库能够分析文件的字节流并提供最可能的编码类型。首先,需要安装该库,然后通过打开文件并读取其内容来检测编码。
在Python中使用哪些方法可以查看文件的编码?
除了chardet
库外,Python标准库中的codecs
模块也可以用来尝试以不同编码打开文件,这样可以通过捕获异常来推断编码格式。另外,使用open
函数时,可以尝试使用errors='replace'
参数来避免因编码错误而导致的程序崩溃。
文件编码不正确时会出现什么问题?
当文件编码不正确时,读取文件内容时可能会出现乱码或抛出UnicodeDecodeError。这样会导致程序无法正常处理文件内容,进而影响数据的处理和分析。因此,了解文件的正确编码是非常重要的。
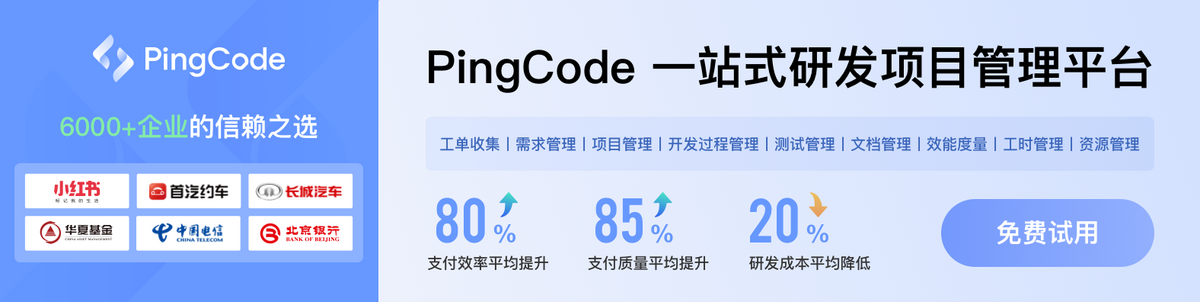