Python通过使用字符串方法、读取和写入文件内容、以及处理文件路径来改变文件的大小写。 在这篇文章中,我们将详细介绍如何使用Python实现文件内容和文件路径的大小写转换,并提供相应的代码示例。
一、文件内容的大小写转换
在处理文件内容时,我们通常需要读取文件内容、转换内容的大小写,然后将其写回文件。下面是实现这一过程的详细步骤。
读取文件内容
首先,我们需要读取文件的内容。可以使用Python的内置open
函数来打开文件,并使用read
方法读取文件的内容。
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
转换内容的大小写
接下来,我们可以使用Python字符串的内置方法lower()
、upper()
和capitalize()
来转换内容的大小写。
def to_lowercase(content):
return content.lower()
def to_uppercase(content):
return content.upper()
def to_capitalize(content):
return content.capitalize()
写回文件
最后,我们需要将转换后的内容写回文件。可以使用open
函数以写模式打开文件,并使用write
方法将内容写回文件。
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
完整示例
下面是一个完整的示例,演示如何将文件内容转换为小写并写回文件。
def change_file_content_case(file_path, case='lower'):
content = read_file(file_path)
if case == 'lower':
content = to_lowercase(content)
elif case == 'upper':
content = to_uppercase(content)
elif case == 'capitalize':
content = to_capitalize(content)
write_file(file_path, content)
使用示例
file_path = 'example.txt'
change_file_content_case(file_path, case='lower')
二、文件路径的大小写转换
除了文件内容,我们有时还需要改变文件路径(包括文件名和扩展名)的大小写。下面是实现这一过程的详细步骤。
获取文件路径
我们可以使用os
模块来获取文件路径,并使用字符串的内置方法来转换路径的大小写。
import os
def change_file_path_case(file_path, case='lower'):
directory, filename = os.path.split(file_path)
name, ext = os.path.splitext(filename)
if case == 'lower':
new_name = name.lower()
new_ext = ext.lower()
elif case == 'upper':
new_name = name.upper()
new_ext = ext.upper()
elif case == 'capitalize':
new_name = name.capitalize()
new_ext = ext.capitalize()
new_filename = new_name + new_ext
new_file_path = os.path.join(directory, new_filename)
os.rename(file_path, new_file_path)
使用示例
file_path = 'Example.TXT'
change_file_path_case(file_path, case='lower')
将文件路径写回文件
在某些情况下,我们可能需要将新的文件路径写回文件中。可以使用上述的写文件方法来实现这一点。
def write_new_file_path(file_path, new_file_path):
with open(file_path, 'a') as file:
file.write(f'\nNew file path: {new_file_path}')
使用示例
file_path = 'Example.TXT'
new_file_path = 'example.txt'
write_new_file_path(file_path, new_file_path)
三、结合文件内容和文件路径的大小写转换
有时,我们可能需要同时转换文件内容和文件路径的大小写。下面是一个实现这一过程的完整示例。
完整示例
def change_file_case(file_path, content_case='lower', path_case='lower'):
# 转换文件内容大小写
change_file_content_case(file_path, case=content_case)
# 转换文件路径大小写
directory, filename = os.path.split(file_path)
name, ext = os.path.splitext(filename)
if path_case == 'lower':
new_name = name.lower()
new_ext = ext.lower()
elif path_case == 'upper':
new_name = name.upper()
new_ext = ext.upper()
elif path_case == 'capitalize':
new_name = name.capitalize()
new_ext = ext.capitalize()
new_filename = new_name + new_ext
new_file_path = os.path.join(directory, new_filename)
os.rename(file_path, new_file_path)
# 将新的文件路径写回文件
write_new_file_path(new_file_path, new_file_path)
使用示例
file_path = 'Example.TXT'
change_file_case(file_path, content_case='lower', path_case='lower')
通过上述步骤和示例代码,我们可以轻松地使用Python实现文件内容和文件路径的大小写转换。无论是处理单个文件还是批量处理多个文件,掌握这些方法都可以极大地提高我们的工作效率。希望这篇文章对你有所帮助,让你在处理文件大小写转换时更加得心应手。
相关问答FAQs:
如何使用Python将文本文件中的所有字母转换为大写?
在Python中,可以使用str.upper()
方法来将文件中的所有字母转换为大写。首先,读取文件内容,将其转换为大写后,再写回文件。以下是一个简单的示例代码:
with open('example.txt', 'r') as file:
content = file.read().upper()
with open('example.txt', 'w') as file:
file.write(content)
这段代码会将example.txt
文件中的所有文本转换为大写字母。
如何将文本文件中的所有字母转换为小写?
要将文件中的所有字母转换为小写,可以使用str.lower()
方法。示例代码如下:
with open('example.txt', 'r') as file:
content = file.read().lower()
with open('example.txt', 'w') as file:
file.write(content)
该代码会将example.txt
文件中的所有文本转换为小写字母。
是否可以使用Python同时将文件中的字母大小写互换?
可以通过使用str.swapcase()
方法来实现大小写互换。以下是相关示例代码:
with open('example.txt', 'r') as file:
content = file.read().swapcase()
with open('example.txt', 'w') as file:
file.write(content)
这段代码会将example.txt
文件中的所有大写字母转换为小写字母,同时将小写字母转换为大写字母。
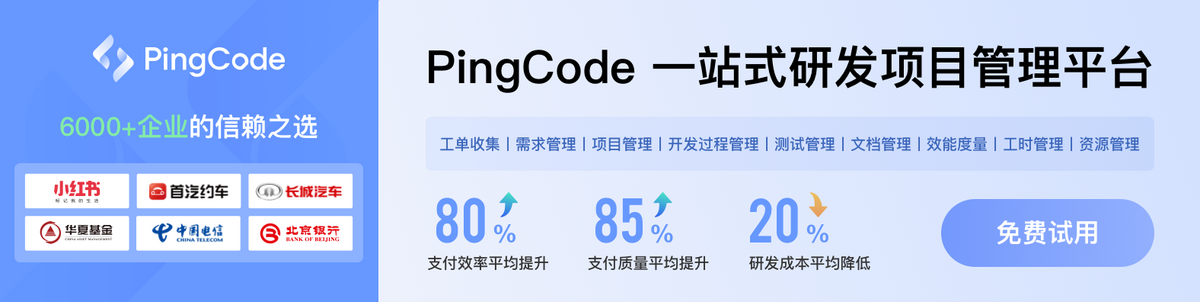