使用Python运行俄罗斯方块有几种方式:使用库如Pygame、编写自己的游戏逻辑、利用现有的开源项目。 其中,使用Pygame库是最常见的方法,因为它提供了丰富的功能来处理游戏开发。Pygame是一种跨平台的Python模块,用于开发视频游戏,包含计算机图形和声音的功能。接下来,我们将详细介绍如何使用Pygame在Python中运行俄罗斯方块。
一、安装Pygame
首先,需要安装Pygame库。可以使用以下命令在命令行或终端中安装:
pip install pygame
确保安装成功后,您可以开始编写俄罗斯方块的代码。
二、初始化Pygame
在编写游戏代码之前,需要初始化Pygame并设置游戏窗口。以下是初始化Pygame和设置窗口的代码示例:
import pygame
import random
初始化Pygame
pygame.init()
设置窗口的大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
设置窗口标题
pygame.display.set_caption("俄罗斯方块")
设置时钟以控制游戏帧率
clock = pygame.time.Clock()
三、定义游戏常量和颜色
在编写游戏逻辑之前,需要定义一些游戏常量和颜色,以便在后续代码中使用:
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
CYAN = (0, 255, 255)
MAGENTA = (255, 0, 255)
定义方块大小
block_size = 30
定义游戏区域大小
cols = 10
rows = 20
定义游戏区域位置
top_left_x = (screen_width - cols * block_size) // 2
top_left_y = screen_height - rows * block_size - 10
四、定义方块形状
俄罗斯方块由不同形状的块组成,通常有7种不同的形状。可以使用二维列表来定义这些形状:
S = [['.....',
'.....',
'..OO.',
'.OO..',
'.....'],
['.....',
'..O..',
'..OO.',
'...O.',
'.....']]
Z = [['.....',
'.....',
'.OO..',
'..OO.',
'.....'],
['.....',
'..O..',
'.OO..',
'.O...',
'.....']]
I = [['.....',
'..O..',
'..O..',
'..O..',
'..O..'],
['.....',
'.....',
'OOOO.',
'.....',
'.....']]
O = [['.....',
'.....',
'.OO..',
'.OO..',
'.....']]
J = [['.....',
'.O...',
'.OOO.',
'.....',
'.....'],
['.....',
'..OO.',
'..O..',
'..O..',
'.....'],
['.....',
'.....',
'.OOO.',
'...O.',
'.....'],
['.....',
'..O..',
'..O..',
'.OO..',
'.....']]
L = [['.....',
'...O.',
'.OOO.',
'.....',
'.....'],
['.....',
'..O..',
'..O..',
'..OO.',
'.....'],
['.....',
'.....',
'.OOO.',
'.O...',
'.....'],
['.....',
'.OO..',
'..O..',
'..O..',
'.....']]
T = [['.....',
'..O..',
'.OOO.',
'.....',
'.....'],
['.....',
'..O..',
'..OO.',
'..O..',
'.....'],
['.....',
'.....',
'.OOO.',
'..O..',
'.....'],
['.....',
'..O..',
'.OO..',
'..O..',
'.....']]
shapes = [S, Z, I, O, J, L, T]
shape_colors = [RED, GREEN, BLUE, YELLOW, CYAN, MAGENTA, WHITE] # 颜色与形状对应
五、定义游戏方块类
为了管理方块状态和行为,可以定义一个类来表示游戏方块:
class Piece(object):
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = shape_colors[shapes.index(shape)]
self.rotation = 0
def get_shape(self):
return self.shape[self.rotation % len(self.shape)]
六、绘制游戏区域和方块
接下来,需要实现绘制游戏区域和方块的功能:
def draw_grid(surface, grid):
for i in range(len(grid)):
for j in range(len(grid[i])):
pygame.draw.rect(surface, grid[i][j], (top_left_x + j * block_size, top_left_y + i * block_size, block_size, block_size), 0)
# 绘制网格线
for i in range(len(grid)):
pygame.draw.line(surface, BLACK, (top_left_x, top_left_y + i * block_size), (top_left_x + cols * block_size, top_left_y + i * block_size))
for j in range(len(grid[i])):
pygame.draw.line(surface, BLACK, (top_left_x + j * block_size, top_left_y), (top_left_x + j * block_size, top_left_y + rows * block_size))
def draw_window(surface, grid):
surface.fill(WHITE)
draw_grid(surface, grid)
pygame.display.update()
七、处理游戏逻辑
游戏的核心逻辑包括处理方块移动、旋转、碰撞检测和行消除等功能:
def create_grid(locked_positions={}):
grid = [[BLACK for _ in range(cols)] for _ in range(rows)]
for i in range(rows):
for j in range(cols):
if (j, i) in locked_positions:
c = locked_positions[(j, i)]
grid[i][j] = c
return grid
def convert_shape_format(shape):
positions = []
format = shape.get_shape()
for i, line in enumerate(format):
row = list(line)
for j, column in enumerate(row):
if column == 'O':
positions.append((shape.x + j, shape.y + i))
for i, pos in enumerate(positions):
positions[i] = (pos[0] - 2, pos[1] - 4)
return positions
def valid_space(shape, grid):
accepted_pos = [[(j, i) for j in range(cols) if grid[i][j] == BLACK] for i in range(rows)]
accepted_pos = [j for sub in accepted_pos for j in sub]
formatted = convert_shape_format(shape)
for pos in formatted:
if pos not in accepted_pos:
if pos[1] >= 0:
return False
return True
def check_lost(positions):
for pos in positions:
x, y = pos
if y < 1:
return True
return False
def get_shape():
return Piece(5, 0, random.choice(shapes))
八、运行游戏
最后,实现主游戏循环来运行俄罗斯方块游戏:
def main():
locked_positions = {}
grid = create_grid(locked_positions)
change_piece = False
run = True
current_piece = get_shape()
next_piece = get_shape()
clock = pygame.time.Clock()
fall_time = 0
while run:
grid = create_grid(locked_positions)
fall_speed = 0.27
fall_time += clock.get_rawtime()
clock.tick()
if fall_time / 1000 >= fall_speed:
fall_time = 0
current_piece.y += 1
if not (valid_space(current_piece, grid)) and current_piece.y > 0:
current_piece.y -= 1
change_piece = True
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
pygame.display.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_piece.x -= 1
if not valid_space(current_piece, grid):
current_piece.x += 1
elif event.key == pygame.K_RIGHT:
current_piece.x += 1
if not valid_space(current_piece, grid):
current_piece.x -= 1
elif event.key == pygame.K_DOWN:
current_piece.y += 1
if not valid_space(current_piece, grid):
current_piece.y -= 1
elif event.key == pygame.K_UP:
current_piece.rotation = current_piece.rotation + 1 % len(current_piece.shape)
if not valid_space(current_piece, grid):
current_piece.rotation = current_piece.rotation - 1 % len(current_piece.shape)
shape_pos = convert_shape_format(current_piece)
for i in range(len(shape_pos)):
x, y = shape_pos[i]
if y > -1:
grid[y][x] = current_piece.color
if change_piece:
for pos in shape_pos:
p = (pos[0], pos[1])
locked_positions[p] = current_piece.color
current_piece = next_piece
next_piece = get_shape()
change_piece = False
if check_lost(locked_positions):
run = False
draw_window(screen, grid)
pygame.display.quit()
main()
以上代码将实现一个基本的俄罗斯方块游戏。通过组合不同的函数和类,我们可以创建一个完整的游戏,从初始化Pygame库到绘制游戏窗口、处理游戏逻辑,再到最终运行游戏。通过不断优化和添加新功能,可以进一步提高游戏的可玩性和体验。
相关问答FAQs:
如何在Python中安装运行俄罗斯方块游戏的依赖库?
要运行俄罗斯方块游戏,您首先需要确保安装了Pygame库,这是一个用于制作游戏的流行Python库。您可以通过在命令行中输入pip install pygame
来安装它。安装完成后,您就可以开始编写或下载俄罗斯方块的代码。
是否有现成的俄罗斯方块游戏代码可以使用?
确实存在很多开源的俄罗斯方块游戏代码,您可以在GitHub等平台上找到这些资源。搜索“Python Tetris”或“Pygame Tetris”可以找到多个项目。下载代码后,确保您已经安装了所有必要的依赖项,然后就可以运行游戏了。
在Python中开发自己的俄罗斯方块游戏需要哪些基本知识?
要开发自己的俄罗斯方块游戏,您需要掌握基本的Python编程知识,特别是面向对象编程。了解如何使用Pygame处理图形、声音和用户输入也是非常重要的。此外,熟悉游戏循环的概念以及如何管理游戏状态和事件将对您的开发过程大有帮助。
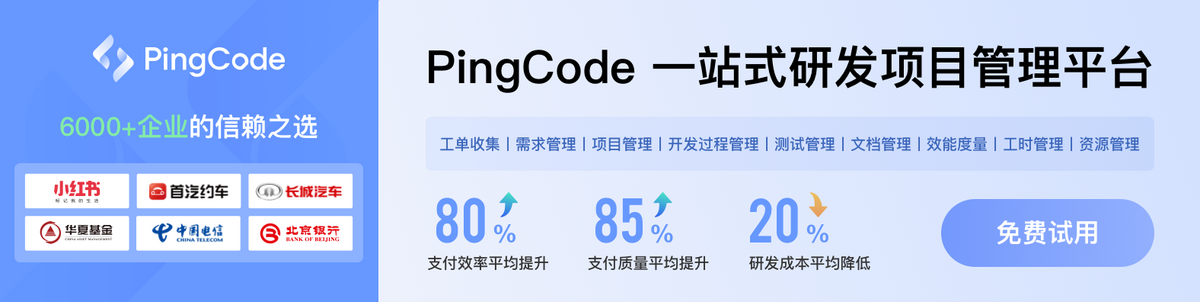