使用相对路径在Python编程中有许多好处,例如使代码更具可移植性、提高代码的可读性、便于项目的组织和管理等。要在Python中编写相对路径,可以使用内置的 os
模块、pathlib
模块等。下面将详细介绍如何在Python中使用相对路径,并给出一些实际的例子。
相对路径是指相对于当前工作目录或脚本所在目录的路径。使用相对路径可以使代码在不同的环境中运行时更加灵活。
一、使用os模块
1、获取当前工作目录
在Python中,可以使用 os.getcwd()
函数获取当前工作目录。当前工作目录是指当前执行程序的目录。
import os
current_directory = os.getcwd()
print(f"当前工作目录: {current_directory}")
2、连接路径
可以使用 os.path.join()
函数将多个路径连接在一起,生成一个新的路径。这样可以避免手动拼接路径时的错误。
import os
relative_path = "subdir/file.txt"
full_path = os.path.join(os.getcwd(), relative_path)
print(f"完整路径: {full_path}")
3、改变当前工作目录
可以使用 os.chdir()
函数改变当前工作目录。这对于需要在不同目录之间切换的程序很有用。
import os
os.chdir("/path/to/new/directory")
print(f"新的工作目录: {os.getcwd()}")
二、使用pathlib模块
1、创建路径对象
pathlib
模块提供了一个面向对象的路径处理方法。可以使用 Path
类创建路径对象。
from pathlib import Path
current_directory = Path.cwd()
print(f"当前工作目录: {current_directory}")
2、连接路径
可以使用 /
操作符连接路径,这使得代码更加简洁和易读。
from pathlib import Path
relative_path = Path("subdir/file.txt")
full_path = Path.cwd() / relative_path
print(f"完整路径: {full_path}")
3、改变当前工作目录
可以使用 Path
类的 chdir()
方法改变当前工作目录。
from pathlib import Path
new_directory = Path("/path/to/new/directory")
new_directory.chdir()
print(f"新的工作目录: {Path.cwd()}")
三、相对路径的实际应用
1、读取文件
在实际应用中,经常需要读取相对路径下的文件。可以使用上述方法获取文件的完整路径,然后使用 open()
函数读取文件内容。
import os
relative_path = "subdir/file.txt"
full_path = os.path.join(os.getcwd(), relative_path)
with open(full_path, "r") as file:
content = file.read()
print(content)
2、写入文件
同样,可以使用相对路径写入文件。
import os
relative_path = "subdir/output.txt"
full_path = os.path.join(os.getcwd(), relative_path)
with open(full_path, "w") as file:
file.write("Hello, World!")
3、列出目录内容
可以使用相对路径列出目录中的文件和子目录。
import os
relative_path = "subdir"
full_path = os.path.join(os.getcwd(), relative_path)
for item in os.listdir(full_path):
print(item)
四、处理跨平台问题
不同操作系统的路径分隔符可能不同。为了编写跨平台的代码,可以使用 os.path
或 pathlib
模块。
1、使用os.path
import os
relative_path = os.path.join("subdir", "file.txt")
full_path = os.path.join(os.getcwd(), relative_path)
print(f"完整路径: {full_path}")
2、使用pathlib
from pathlib import Path
relative_path = Path("subdir") / "file.txt"
full_path = Path.cwd() / relative_path
print(f"完整路径: {full_path}")
五、在项目中使用相对路径
在实际项目中,通常会有多个文件和子目录。为了更好地组织和管理项目,可以使用相对路径。
1、项目结构
假设有以下项目结构:
project/
│
├── src/
│ ├── main.py
│ └── utils.py
│
├── data/
│ └── input.txt
│
└── output/
└── result.txt
2、读取数据文件
在 src/main.py
中读取 data/input.txt
文件。
from pathlib import Path
data_path = Path(__file__).resolve().parent.parent / "data" / "input.txt"
with open(data_path, "r") as file:
content = file.read()
print(content)
3、写入结果文件
在 src/main.py
中写入 output/result.txt
文件。
from pathlib import Path
output_path = Path(__file__).resolve().parent.parent / "output" / "result.txt"
with open(output_path, "w") as file:
file.write("Hello, World!")
4、导入模块
在 src/main.py
中导入 src/utils.py
中的函数。
from pathlib import Path
import sys
添加src目录到sys.path
sys.path.append(str(Path(__file__).resolve().parent))
from utils import some_function
some_function()
总结
使用相对路径可以使Python代码更具可移植性和可读性。在实际项目中,建议使用 pathlib
模块,因为它提供了更简洁和强大的路径处理方法。通过合理组织项目结构和使用相对路径,可以提高项目的可维护性和可扩展性。
相关问答FAQs:
相对路径在Python中是什么意思?
相对路径是指相对于当前工作目录的文件或目录的位置。在Python中,当你使用相对路径时,代码会根据当前工作目录来查找文件,这样可以使得代码在不同环境下更具灵活性。
如何在Python中获取当前工作目录?
可以使用os
模块中的getcwd()
函数来获取当前的工作目录。示例如下:
import os
current_directory = os.getcwd()
print(current_directory)
这段代码会打印出当前的工作目录,你可以据此决定相对路径的起点。
使用相对路径时需要注意哪些事项?
在使用相对路径时,确保正确理解当前工作目录的位置。如果在一个脚本中使用相对路径,运行该脚本时的工作目录可能会影响路径的解析。此外,避免在代码中硬编码绝对路径,以提高代码的可移植性和可维护性。建议使用os.path.join()
函数来构建路径,以确保在不同操作系统上的兼容性。
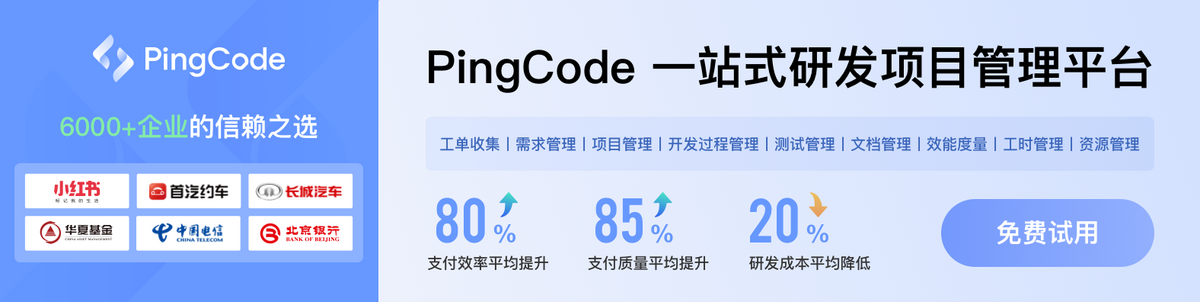