Python计算程序运行时间的方法有多种:使用time模块、使用datetime模块、使用timeit模块。其中,使用time模块是最常见和简单的方法。
一、使用time模块
time模块提供了time()函数可以获取当前时间戳,通过在程序开始和结束时分别调用time()函数,计算它们的差值即可得到程序的运行时间。
import time
start_time = time.time()
Your code here
end_time = time.time()
execution_time = end_time - start_time
print(f"Program execution time: {execution_time} seconds")
在上面的代码中,我们首先使用time.time()记录程序开始的时间戳,然后在程序结束时再次调用time.time()记录结束的时间戳,最后通过相减得到程序的运行时间。
二、使用datetime模块
datetime模块提供了更高层次的时间处理功能,我们可以通过获取当前时间的datetime对象来计算时间差。
from datetime import datetime
start_time = datetime.now()
Your code here
end_time = datetime.now()
execution_time = end_time - start_time
print(f"Program execution time: {execution_time}")
在上面的代码中,我们使用datetime.now()获取当前时间的datetime对象,通过相减得到程序的运行时间。datetime对象的相减会返回一个timedelta对象,timedelta对象自动处理秒和微秒的转换。
三、使用timeit模块
timeit模块专门用于计时小段代码的执行时间,它提供了一个简单的方式来测试代码的性能。timeit模块通过多次执行代码片段来消除偶然误差。
import timeit
code_to_test = """
a = 10
b = 20
c = a + b
"""
execution_time = timeit.timeit(code_to_test, number=1000000)
print(f"Program execution time: {execution_time} seconds")
在上面的代码中,我们将要测试的代码片段作为字符串传递给timeit.timeit()函数,参数number指定代码片段要执行的次数。timeit.timeit()函数返回多次执行的总时间。
四、使用装饰器记录函数运行时间
装饰器是Python中一个非常强大的功能,可以用来修改函数的行为。我们可以定义一个装饰器来记录函数的运行时间。
import time
def time_decorator(func):
def wrapper(*args, kwargs):
start_time = time.time()
result = func(*args, kwargs)
end_time = time.time()
execution_time = end_time - start_time
print(f"Function {func.__name__} execution time: {execution_time} seconds")
return result
return wrapper
@time_decorator
def example_function():
time.sleep(2)
example_function()
在上面的代码中,我们定义了一个名为time_decorator的装饰器,装饰器内部记录了函数开始和结束的时间,计算并打印函数的运行时间。通过使用@time_decorator语法糖,我们将example_function函数装饰起来,调用example_function时会自动记录并打印其运行时间。
五、在大型项目中管理时间记录
在大型项目中,管理和记录程序运行时间可能会变得复杂。我们可以使用Python的日志模块来更好地管理和记录时间。
import time
import logging
Configure logging
logging.basicConfig(level=logging.INFO)
def log_time_decorator(func):
def wrapper(*args, kwargs):
start_time = time.time()
result = func(*args, kwargs)
end_time = time.time()
execution_time = end_time - start_time
logging.info(f"Function {func.__name__} execution time: {execution_time} seconds")
return result
return wrapper
@log_time_decorator
def example_function():
time.sleep(2)
example_function()
在上面的代码中,我们使用Python的logging模块配置了日志记录,通过日志记录函数的运行时间,这样可以在大型项目中更好地管理和查看时间记录。
六、使用性能分析工具
对于复杂的程序,简单的时间记录可能无法提供足够的信息。我们可以使用一些性能分析工具,如cProfile模块,来详细分析程序的运行时间。
import cProfile
def example_function():
for i in range(1000000):
pass
cProfile.run('example_function()')
在上面的代码中,我们使用cProfile.run()函数来运行example_function并记录其性能数据,cProfile模块会提供详细的函数调用和运行时间信息。
总结
Python提供了多种方法来计算程序运行时间,包括使用time模块、datetime模块、timeit模块、装饰器、日志模块和性能分析工具。根据不同的需求和场景,我们可以选择合适的方法来记录和分析程序的运行时间。通过合理地记录和分析程序的运行时间,我们可以更好地优化和提高程序的性能。
相关问答FAQs:
如何在Python中测量代码块的执行时间?
在Python中,可以使用time
模块来测量代码块的执行时间。通过在代码开始时记录当前时间,然后在代码结束时再次记录当前时间,最后计算两者之间的差值来获得执行时间。例如:
import time
start_time = time.time()
# 你的代码块
end_time = time.time()
execution_time = end_time - start_time
print(f"执行时间: {execution_time}秒")
使用哪些库可以更精确地测量程序运行时间?
除了time
模块,Python的timeit
模块提供了一种更精确的方法来测量小代码段的执行时间。timeit
可以自动多次执行代码并计算平均运行时间,减少了单次执行时的随机波动。使用示例如下:
import timeit
execution_time = timeit.timeit('your_code_here', number=1000)
print(f"平均执行时间: {execution_time}秒")
在Python中如何测量整个程序的运行时间?
如果想测量整个程序的运行时间,可以在程序的开始和结束处使用time
模块记录时间。这种方法适合于较大的程序或脚本。以下是一个简单的示例:
import time
start_time = time.time()
# 整个程序的代码
end_time = time.time()
total_execution_time = end_time - start_time
print(f"程序总执行时间: {total_execution_time}秒")
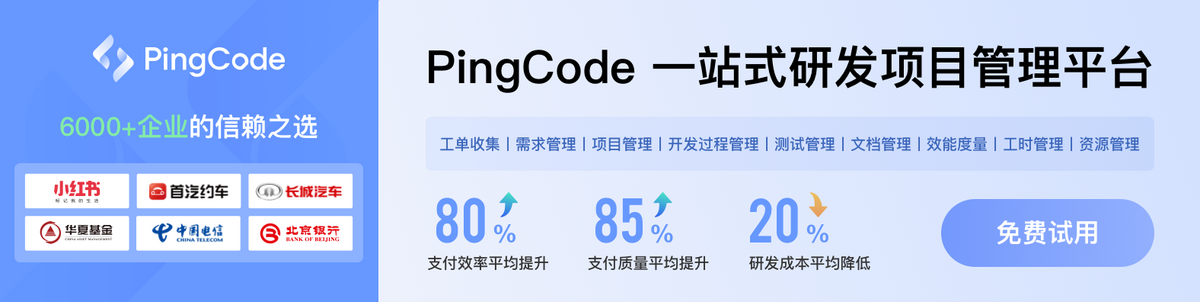