Python格式化输入输出的几种方法包括:使用百分号 (%) 操作符、str.format() 方法、f-strings (格式化字符串字面量)、以及format() 函数。 其中,f-strings 是 Python 3.6 及更高版本中的新特性,因其简洁和直观的语法而广受欢迎。接下来,我们将详细介绍这些方法的使用方式和特点。
一、百分号 (%) 操作符
百分号 (%) 操作符是 Python 中最早期的字符串格式化方法。它类似于 C 语言中的 printf 格式化。通过在字符串中使用 % 进行占位,并在后面提供一个值或元组来填充占位符,可以实现字符串的格式化。
例如:
name = "John"
age = 30
print("My name is %s and I am %d years old." % (name, age))
在这个例子中,%s
占位符将会被字符串 name
替代,而 %d
占位符将会被整数 age
替代。
二、str.format() 方法
Python 2.6 引入了 str.format()
方法,它比百分号操作符更强大和灵活。通过在字符串中使用 {}
作为占位符,并在 format()
方法中传递相应的值,可以实现字符串的格式化。
例如:
name = "John"
age = 30
print("My name is {} and I am {} years old.".format(name, age))
str.format() 方法的优点之一是可以通过位置或名字进行替换。
例如:
name = "John"
age = 30
print("My name is {0} and I am {1} years old.".format(name, age))
print("My name is {name} and I am {age} years old.".format(name=name, age=age))
三、f-strings (格式化字符串字面量)
f-strings 是 Python 3.6 引入的一种新的字符串格式化方法。它使用前缀 f
或 F
,并在字符串内部使用 {}
进行占位,括号内可以直接书写表达式。
例如:
name = "John"
age = 30
print(f"My name is {name} and I am {age} years old.")
f-strings 提供了更简洁和直观的语法,且可以在字符串中直接嵌入表达式。
例如:
x = 10
y = 20
print(f"The sum of {x} and {y} is {x + y}.")
四、format() 函数
虽然 str.format()
和 f-strings 是比较常用的字符串格式化方法,但 Python 还有一个 format()
函数,它可以用于格式化数值类型的数据,如浮点数和整数。
例如:
pi = 3.141592653589793
print("Pi rounded to 2 decimal places: {:.2f}".format(pi))
在这个例子中,{:.2f}
表示将浮点数 pi
格式化为小数点后两位。
五、输入格式化
在 Python 中,可以使用 input()
函数来获取用户输入,并通过上述方法对输入进行格式化。例如:
name = input("Enter your name: ")
age = int(input("Enter your age: "))
print(f"Your name is {name} and you are {age} years old.")
通过 input()
获取用户输入后,可以使用 f-strings 或其他格式化方法将输入格式化为所需的输出形式。
六、综合示例
下面是一个综合示例,展示了如何结合上述多种方法进行输入输出格式化:
def main():
name = input("Enter your name: ")
age = int(input("Enter your age: "))
height = float(input("Enter your height in meters: "))
weight = float(input("Enter your weight in kilograms: "))
bmi = weight / (height 2)
print("\nUsing % operator:")
print("Name: %s, Age: %d, Height: %.2f meters, Weight: %.2f kg, BMI: %.2f" % (name, age, height, weight, bmi))
print("\nUsing str.format():")
print("Name: {}, Age: {}, Height: {:.2f} meters, Weight: {:.2f} kg, BMI: {:.2f}".format(name, age, height, weight, bmi))
print("\nUsing f-strings:")
print(f"Name: {name}, Age: {age}, Height: {height:.2f} meters, Weight: {weight:.2f} kg, BMI: {bmi:.2f}")
if __name__ == "__main__":
main()
在这个示例中,我们首先通过 input()
获取用户输入,然后分别使用 %
操作符、str.format()
方法和 f-strings 对输入进行格式化并输出。
七、进阶格式化选项
在实际应用中,可能需要对输出进行更复杂的格式化,例如对齐、填充、设置宽度等。Python 提供了一些进阶的格式化选项,以下是一些常见的用法:
1. 对齐和填充
可以在 {}
中使用冒号 :
指定对齐和填充选项:
<
左对齐>
右对齐^
居中对齐=
将符号放在填充内容的左边
例如:
print(f"{'left':<10} | {'right':>10} | {'center':^10}")
print(f"{123:<10} | {456:>10} | {789:^10}")
2. 设置宽度
可以在对齐符号后面指定宽度:
print(f"{123:<10} | {456:>10} | {789:^10}")
3. 填充字符
可以在宽度和对齐符号之间添加填充字符:
print(f"{123:*<10} | {456:*>10} | {789:*^10}")
4. 数字格式化
可以使用逗号 ,
对数字进行分组:
number = 1000000
print(f"{number:,}")
可以使用 b
、o
、x
、X
格式化数字为二进制、八进制、十六进制(小写和大写):
number = 255
print(f"{number:b} (binary), {number:o} (octal), {number:x} (hex), {number:X} (HEX)")
八、总结
Python 提供了多种字符串格式化方法,包括百分号 (%) 操作符、str.format()
方法、f-strings (格式化字符串字面量) 和 format()
函数。这些方法各有特点,适用于不同的场景和需求。在实际应用中,可以根据具体情况选择合适的格式化方法,以提高代码的可读性和维护性。
通过掌握这些字符串格式化方法,能够更灵活地处理输入输出,并将数据以美观和易读的方式展示给用户。希望本文对你理解和应用 Python 格式化输入输出有所帮助。
相关问答FAQs:
如何在Python中使用格式化字符串?
在Python中,格式化字符串可以通过多种方式实现。最常用的方法是使用f-strings(格式化字符串字面量),它允许你在字符串前加上字母f
,并在花括号中插入变量。例如:name = "Alice"; age = 30; print(f"My name is {name} and I am {age} years old.")
。这样可以让输出更加简洁和易读。
Python中常用的格式化输出方法有哪些?
除了f-strings,Python还支持其他几种格式化输出的方法。str.format()
方法可以用于更复杂的格式化需求,例如:"Hello, {0}. You are {1} years old.".format(name, age)
。此外,使用百分号(%)也是一种传统方法,如:"Hello, %s. You are %d years old." % (name, age)
。
如何控制输出的数字格式?
在Python中,可以使用格式规范来控制数字的输出格式。例如,使用:.2f
可以将浮点数格式化为两位小数:pi = 3.14159; print(f"Pi is approximately {pi:.2f}")
。对于整数,可以使用:,
来添加千位分隔符,如:number = 1000000; print(f"Number: {number:,}")
,这会输出Number: 1,000,000
。
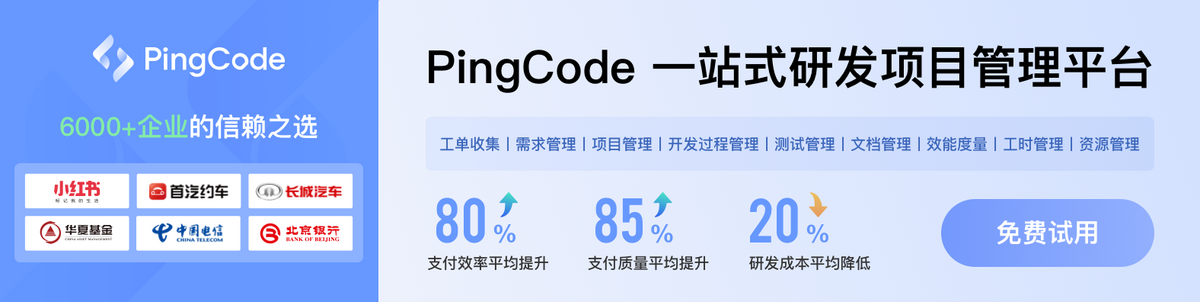