要在Python中将两张图像重叠,可以使用多个库来实现,如Pillow、OpenCV和Matplotlib。主要方法包括使用Pillow库进行图像叠加、使用OpenCV进行图像合并、使用Matplotlib进行图像展示。以下是一个详细的实现方法,使用Pillow库进行图像叠加。
Pillow库提供了简单且强大的图像处理功能,可以方便地实现图像叠加。
一、安装所需库
在开始之前,请确保已经安装了所需的Python库。可以使用以下命令安装Pillow:
pip install pillow
二、使用Pillow库叠加图像
Pillow库是Python Imaging Library (PIL) 的一个分支,提供了图像处理的功能。以下是一个简单的示例,演示如何使用Pillow库将两张图像叠加。
from PIL import Image
打开两张图像
image1 = Image.open("path/to/your/first/image.png")
image2 = Image.open("path/to/your/second/image.png")
确保两张图像的大小相同
image1 = image1.resize((image2.width, image2.height))
叠加图像
blended_image = Image.blend(image1, image2, alpha=0.5)
保存或显示结果
blended_image.save("path/to/save/blended_image.png")
blended_image.show()
在上面的代码中,我们首先打开两张图像,并将第一张图像的大小调整为与第二张图像相同,然后使用Image.blend()
函数将两张图像叠加在一起。参数alpha
控制两张图像的透明度比例。
三、OpenCV库叠加图像
OpenCV是一个强大的计算机视觉库,提供了丰富的图像处理功能。可以使用OpenCV实现图像叠加。以下是一个示例:
import cv2
读取图像
image1 = cv2.imread("path/to/your/first/image.png")
image2 = cv2.imread("path/to/your/second/image.png")
确保两张图像的大小相同
image1 = cv2.resize(image1, (image2.shape[1], image2.shape[0]))
叠加图像
blended_image = cv2.addWeighted(image1, 0.5, image2, 0.5, 0)
保存或显示结果
cv2.imwrite("path/to/save/blended_image.png", blended_image)
cv2.imshow("Blended Image", blended_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
在上面的代码中,使用cv2.addWeighted()
函数将两张图像叠加。参数0.5
表示每张图像的权重。
四、Matplotlib库展示图像
Matplotlib是一个绘图库,通常用于数据可视化。可以使用Matplotlib展示叠加后的图像。以下是一个示例:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
import numpy as np
读取图像
image1 = mpimg.imread("path/to/your/first/image.png")
image2 = mpimg.imread("path/to/your/second/image.png")
确保两张图像的大小相同
image1_resized = np.array(Image.fromarray(image1).resize((image2.shape[1], image2.shape[0])))
叠加图像
blended_image = 0.5 * image1_resized + 0.5 * image2
展示结果
plt.imshow(blended_image.astype(np.uint8))
plt.axis('off') # 不显示坐标轴
plt.show()
在上面的代码中,使用Matplotlib的imshow()
函数展示叠加后的图像。
五、总结
以上介绍了三种使用Python库将两张图像叠加的方法。Pillow库适用于简单的图像处理任务,OpenCV提供了更强大的图像处理功能,Matplotlib则非常适合数据可视化和图像展示。根据不同的需求,可以选择合适的库来实现图像叠加。
相关问答FAQs:
如何在Python中实现图像的重叠效果?
在Python中,可以使用PIL(Pillow)库来处理图像并实现重叠效果。首先,需要安装Pillow库,可以使用命令pip install Pillow
。接着,加载两张图像并使用Image.blend()
函数或Image.alpha_composite()
函数来创建重叠效果,具体取决于图像的透明度需求。
有哪些Python库可以用来处理图像重叠?
除了Pillow外,还有其他几个流行的库可以实现图像处理和重叠效果,例如OpenCV和Matplotlib。OpenCV提供强大的图像处理功能,适合进行复杂的图像操作,而Matplotlib则适合用于展示和可视化图像。
如何调整重叠图像的透明度?
在使用Pillow库进行图像重叠时,可以通过设置图像的透明度来调整重叠效果。可以使用putalpha()
方法设置图像的透明度,或在使用Image.blend()
函数时,调整权重参数以控制两张图像的混合比例。例如,Image.blend(image1, image2, alpha)
中的alpha
值可以设置为0到1之间的浮点数,代表两张图像的混合比例。
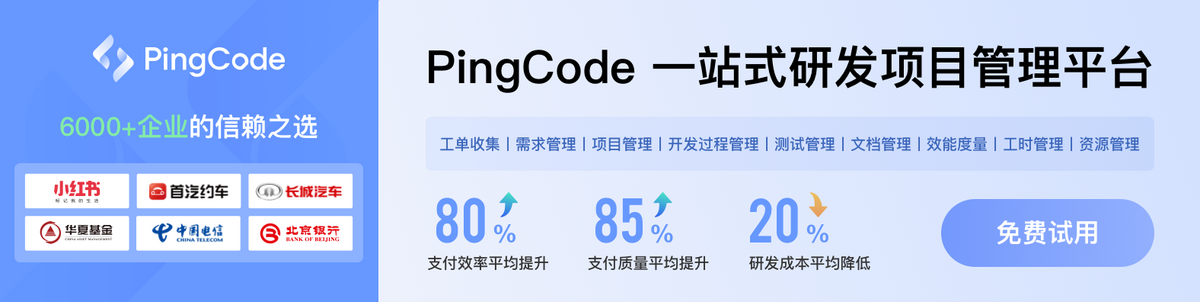