Python将文字转化为数据的方法包括:使用字符串转换函数、使用正则表达式、使用自然语言处理库。 其中,使用自然语言处理库是一种详细、有效且常用的方法,可以实现文本数据的预处理、特征提取以及文本向量化等操作。下面我们将详细探讨这些方法,并介绍如何在实际项目中应用这些技术。
一、字符串转换函数
Python内置了一些函数,可以直接将字符串转换为不同的数据类型。常见的函数包括int()
、float()
、str()
等。通过这些函数,可以将字符串表示的数字、浮点数等转换为相应的数据类型。
例如:
# 将字符串转换为整数
num_str = "123"
num_int = int(num_str)
print(num_int) # 输出:123
将字符串转换为浮点数
float_str = "123.45"
num_float = float(float_str)
print(num_float) # 输出:123.45
这些简单的转换函数在处理基本的数字转换时非常有用,但对于复杂的文本数据处理,可能需要更高级的技术。
二、正则表达式
正则表达式是一种强大的文本处理工具,可以用于查找、替换和拆分字符串。Python的re
库提供了对正则表达式的支持,可以方便地处理文本数据。
例如,使用正则表达式提取字符串中的数字:
import re
text = "The price of the item is $123.45"
提取字符串中的数字
numbers = re.findall(r'\d+\.\d+|\d+', text)
print(numbers) # 输出:['123.45']
通过正则表达式,可以灵活地处理各种文本模式,提取所需的数据。
三、自然语言处理库
自然语言处理(NLP)是处理和分析大量自然语言数据的技术。Python有多个强大的NLP库,如NLTK、spaCy和TextBlob,可以用于文本数据的预处理、特征提取和文本向量化。
以下是使用这些NLP库的一些示例:
- NLTK
NLTK(Natural Language Toolkit)是一个广泛使用的NLP库,提供了丰富的工具和资源,用于处理和分析文本数据。
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
下载必要的资源
nltk.download('punkt')
nltk.download('stopwords')
text = "Natural Language Processing with Python is interesting and useful."
分词
words = word_tokenize(text)
print(words) # 输出:['Natural', 'Language', 'Processing', 'with', 'Python', 'is', 'interesting', 'and', 'useful', '.']
去除停用词
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word.lower() not in stop_words]
print(filtered_words) # 输出:['Natural', 'Language', 'Processing', 'Python', 'interesting', 'useful', '.']
- spaCy
spaCy是另一个流行的NLP库,提供了高效的文本处理功能,并支持多种语言。
import spacy
加载英语模型
nlp = spacy.load('en_core_web_sm')
text = "Natural Language Processing with Python is interesting and useful."
处理文本
doc = nlp(text)
提取词干
stems = [token.lemma_ for token in doc]
print(stems) # 输出:['natural', 'language', 'process', 'with', 'python', 'be', 'interest', 'and', 'useful', '.']
- TextBlob
TextBlob是一个简单易用的NLP库,适用于快速的文本处理和情感分析。
from textblob import TextBlob
text = "Natural Language Processing with Python is interesting and useful."
创建TextBlob对象
blob = TextBlob(text)
分词
words = blob.words
print(words) # 输出:['Natural', 'Language', 'Processing', 'with', 'Python', 'is', 'interesting', 'and', 'useful']
情感分析
sentiment = blob.sentiment
print(sentiment) # 输出:Sentiment(polarity=0.5, subjectivity=0.6)
四、文本向量化
文本向量化是将文本数据转换为数值表示的过程,是自然语言处理中的重要步骤。常见的文本向量化方法包括词袋模型(Bag of Words)、TF-IDF和词嵌入(Word Embedding)。
- 词袋模型(Bag of Words)
词袋模型是最简单的文本向量化方法,通过统计文本中每个词的出现次数来表示文本。可以使用scikit-learn
库中的CountVectorizer
实现词袋模型。
from sklearn.feature_extraction.text import CountVectorizer
texts = ["Natural Language Processing with Python is interesting and useful.",
"Python is a powerful programming language."]
创建CountVectorizer对象
vectorizer = CountVectorizer()
进行词袋模型转换
X = vectorizer.fit_transform(texts)
查看特征名称
print(vectorizer.get_feature_names_out())
输出:['and', 'interesting', 'is', 'language', 'natural', 'processing', 'python', 'useful', 'with', 'a', 'powerful', 'programming']
查看转换后的稀疏矩阵
print(X.toarray())
输出:[[1 1 1 1 1 1 1 1 1 0 0 0]
[0 0 1 1 0 0 1 0 0 1 1 1]]
- TF-IDF
TF-IDF(Term Frequency-Inverse Document Frequency)是另一种常用的文本向量化方法,通过衡量词的重要性来表示文本。可以使用scikit-learn
库中的TfidfVectorizer
实现TF-IDF。
from sklearn.feature_extraction.text import TfidfVectorizer
texts = ["Natural Language Processing with Python is interesting and useful.",
"Python is a powerful programming language."]
创建TfidfVectorizer对象
vectorizer = TfidfVectorizer()
进行TF-IDF转换
X = vectorizer.fit_transform(texts)
查看特征名称
print(vectorizer.get_feature_names_out())
输出:['and', 'interesting', 'is', 'language', 'natural', 'processing', 'python', 'useful', 'with', 'a', 'powerful', 'programming']
查看转换后的稀疏矩阵
print(X.toarray())
输出:[[0. 0.40052315 0.23107084 0.23107084 0.40052315 0.40052315
0.23107084 0.40052315 0.40052315 0. 0. 0. ]
[0. 0. 0.27230147 0.27230147 0. 0.
0.27230147 0. 0. 0.53999499 0.53999499 0.53999499]]
- 词嵌入(Word Embedding)
词嵌入是一种将词表示为连续向量的方法,能够捕捉词与词之间的语义关系。常用的词嵌入方法有Word2Vec、GloVe和FastText。可以使用gensim
库来实现Word2Vec词嵌入。
from gensim.models import Word2Vec
from nltk.tokenize import word_tokenize
准备文本数据
texts = ["Natural Language Processing with Python is interesting and useful.",
"Python is a powerful programming language."]
分词
tokenized_texts = [word_tokenize(text) for text in texts]
训练Word2Vec模型
model = Word2Vec(sentences=tokenized_texts, vector_size=100, window=5, min_count=1, workers=4)
查看词向量
word_vector = model.wv['Python']
print(word_vector)
输出:[-0.00588213 0.01087461 0.00511367 ...] # 词向量的具体值
五、总结
通过上述方法,Python可以将文字转化为数据,便于进一步的分析和处理。字符串转换函数、正则表达式、自然语言处理库等方法可以解决不同场景下的文本处理需求。文本向量化方法如词袋模型、TF-IDF和词嵌入等,可以将文本数据转换为数值表示,为机器学习和数据分析提供基础。
在实际项目中,根据具体需求选择合适的方法和工具,可以大大提高文本处理的效率和效果。希望本篇文章能够帮助你更好地理解和应用Python进行文本数据处理。
相关问答FAQs:
如何使用Python将文本数据转换为数字格式?
在Python中,可以使用多种库和方法将文本转换为数字格式。常用的库包括Pandas和NumPy。Pandas提供了强大的数据处理功能,可以通过pd.to_numeric()
函数将字符串格式的数字转换为实际的数字类型。此外,NumPy的astype()
方法也能实现类似的功能,将数组中的数据类型进行转换。
如何处理文本中的非数字字符以便转换为数据?
在文本中,可能会有非数字字符,这会影响转换。可以使用正则表达式(re
模块)来清洗数据,删除非数字字符。例如,使用re.sub(r'\D', '', text)
可以将文本中的所有非数字字符替换为空。清洗后的文本可以更容易地转换为数字。
如何将文本数据转化为机器学习模型可用的格式?
在机器学习中,文本数据通常需要进行向量化处理。可以使用CountVectorizer
或TfidfVectorizer
这两个类来自sklearn库,将文本数据转换为数值特征。这些方法能够将文本转换为稀疏矩阵的形式,便于后续的模型训练和预测。通过设置不同的参数,还可以控制向量化的方式和结果。
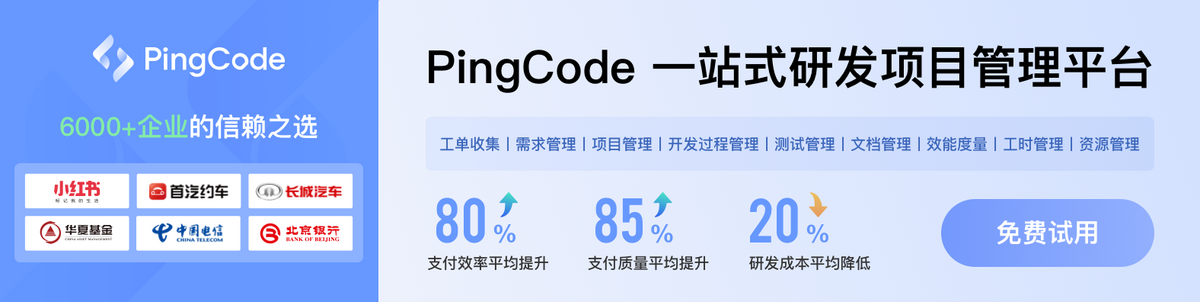