程序语言Python如何抓取信息?
使用Python抓取信息的主要步骤包括:选择合适的库、发送HTTP请求、解析HTML内容、处理数据。 推荐使用的库有requests、BeautifulSoup、Selenium等。选择合适的库和方法是成功抓取信息的关键。
一、选择合适的库
1. Requests库
Requests库是Python中最流行的HTTP库之一,它可以用来发送HTTP请求以抓取网页内容。它的优点是简单易用,且功能强大。
import requests
response = requests.get('http://example.com')
if response.status_code == 200:
page_content = response.text
print(page_content)
2. BeautifulSoup库
BeautifulSoup是一个用来解析HTML和XML文件的Python库。它能通过解析HTML文档来提取信息,非常适合用来处理静态网页。
from bs4 import BeautifulSoup
soup = BeautifulSoup(page_content, 'html.parser')
print(soup.title.text)
3. Selenium库
Selenium是一个用于自动化Web应用测试的工具,支持通过Python脚本来控制浏览器。它适合用来处理动态网页内容。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
page_content = driver.page_source
print(page_content)
driver.quit()
二、发送HTTP请求
1. 使用Requests发送HTTP请求
Requests库可以方便地发送GET和POST请求,获取网页内容。
response = requests.get('http://example.com')
print(response.text)
2. 处理HTTP请求的状态码
在发送HTTP请求后,可以通过检查状态码来确定请求是否成功。
if response.status_code == 200:
print("Request was successful")
else:
print("Request failed")
三、解析HTML内容
1. 使用BeautifulSoup解析HTML
BeautifulSoup库可以将HTML文档转换成一个树形结构,方便提取信息。
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
2. 查找特定标签和属性
可以使用BeautifulSoup提供的方法来查找特定的HTML标签和属性。
title_tag = soup.find('title')
print(title_tag.text)
all_links = soup.find_all('a')
for link in all_links:
print(link.get('href'))
四、处理数据
1. 提取并保存信息
从HTML文档中提取的信息可以保存到文件或数据库中,以便后续处理。
with open('output.txt', 'w') as file:
file.write(soup.prettify())
2. 数据清洗和处理
提取的信息可能需要进行清洗和处理,以便进一步分析。
import re
cleaned_data = re.sub(r'\s+', ' ', soup.get_text())
print(cleaned_data)
五、应用场景和案例
1. 抓取新闻网站的信息
可以使用Requests和BeautifulSoup库来抓取新闻网站的标题和文章内容。
url = 'http://newswebsite.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
articles = soup.find_all('article')
for article in articles:
title = article.find('h2').text
content = article.find('p').text
print(f'Title: {title}\nContent: {content}\n')
2. 抓取电子商务网站的商品信息
可以使用Selenium库来抓取电子商务网站的商品标题、价格和图片链接。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://ecommercewebsite.com')
products = driver.find_elements_by_class_name('product')
for product in products:
title = product.find_element_by_tag_name('h2').text
price = product.find_element_by_class_name('price').text
image = product.find_element_by_tag_name('img').get_attribute('src')
print(f'Title: {title}\nPrice: {price}\nImage: {image}\n')
driver.quit()
六、常见问题及解决方案
1. 处理动态内容
对于动态内容,可以使用Selenium库来模拟浏览器操作,等待页面加载完成后再获取内容。
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver.get('http://dynamicwebsite.com')
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, 'dynamic-content'))
)
page_content = driver.page_source
print(page_content)
2. 处理反爬虫机制
一些网站可能会有反爬虫机制,可以使用以下方法来规避:
- 修改User-Agent
- 使用代理服务器
- 模拟人类行为(如随机等待)
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get('http://example.com', headers=headers)
print(response.text)
七、抓取信息的最佳实践
1. 遵守网站的Robots.txt规则
在抓取信息时,应遵守网站的Robots.txt规则,确保不对网站造成过大的压力。
import requests
response = requests.get('http://example.com/robots.txt')
print(response.text)
2. 限制请求频率
为了避免对网站造成过大的压力,应该限制请求的频率,可以使用time.sleep()来实现。
import time
for i in range(10):
response = requests.get(f'http://example.com/page/{i}')
print(response.text)
time.sleep(2) # 等待2秒
3. 使用多线程或异步请求
对于大规模的数据抓取,可以使用多线程或异步请求来提高效率。
import threading
def fetch_data(url):
response = requests.get(url)
print(response.text)
threads = []
for i in range(10):
t = threading.Thread(target=fetch_data, args=(f'http://example.com/page/{i}',))
threads.append(t)
t.start()
for t in threads:
t.join()
4. 处理抓取失败的情况
在抓取信息时,可能会遇到请求失败的情况,应当进行异常处理,确保程序的稳定性。
try:
response = requests.get('http://example.com')
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f'Request failed: {e}')
八、总结
通过使用Python的Requests、BeautifulSoup和Selenium库,可以方便地抓取网页中的信息。选择合适的库和方法是成功抓取信息的关键。在抓取信息时,应注意遵守网站的Robots.txt规则,限制请求频率,处理反爬虫机制,并进行异常处理,以确保程序的稳定性和高效性。
相关问答FAQs:
如何使用Python抓取网页信息?
抓取网页信息通常涉及使用库如BeautifulSoup和Requests。首先,使用Requests库获取网页的HTML内容,然后利用BeautifulSoup解析HTML文档,从中提取所需的信息。例如,可以抓取特定标签的文本、链接或图像等内容。确保遵循网站的robots.txt文件,以避免抓取限制。
抓取信息时需要注意哪些法律和道德问题?
在抓取信息时,务必遵循相关法律和道德标准。尊重网站的版权和隐私政策,避免过于频繁的请求以免对服务器造成压力。同时,阅读并遵循网站的使用条款和条件,确保抓取行为不会侵犯他人的合法权益。
Python抓取信息的效率如何提高?
提高抓取效率可以通过多线程或异步请求来实现。使用库如aiohttp进行异步请求,可以在等待响应时同时处理多个请求,从而节省时间。此外,合理设置请求频率和使用缓存机制也能显著提升抓取效率,减少重复请求相同数据的时间。
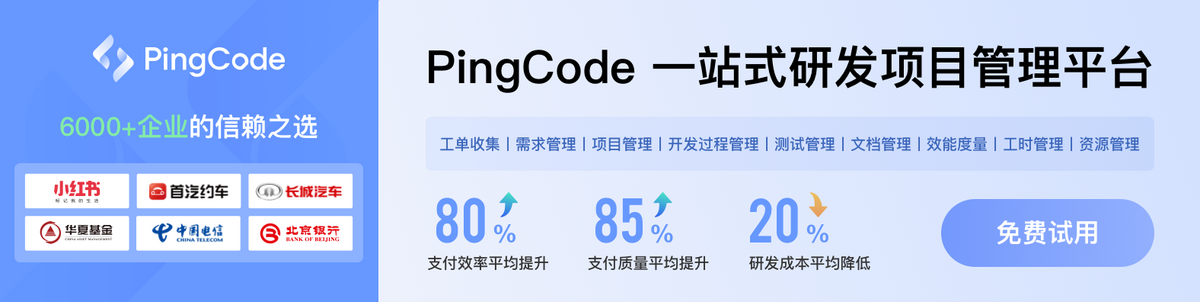