在Python中连接指定数据库的方法有很多,根据不同的数据库类型,连接方式也有所不同。核心观点包括:使用合适的数据库驱动、配置数据库连接参数、使用连接池管理连接、处理数据库连接错误。本文将详细介绍如何连接不同类型的数据库,并提供相关示例代码。
一、使用合适的数据库驱动
不同类型的数据库需要使用不同的驱动程序。Python中常用的数据库驱动包括:
- MySQL:使用
mysql-connector-python
或PyMySQL
驱动 - PostgreSQL:使用
psycopg2
驱动 - SQLite:使用内置的
sqlite3
模块 - SQL Server:使用
pyodbc
驱动 - Oracle:使用
cx_Oracle
驱动
选择合适的数据库驱动是连接数据库的第一步。例如,连接MySQL数据库时,可以选择mysql-connector-python
或PyMySQL
驱动。以下是使用mysql-connector-python
连接MySQL数据库的示例:
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
}
try:
connection = mysql.connector.connect(config)
if connection.is_connected():
print("Connected to MySQL database")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
connection.close()
print("Connection closed")
二、配置数据库连接参数
配置数据库连接参数包括设置用户名、密码、主机地址、端口号以及数据库名称等。这些参数通常存储在配置文件或环境变量中,以确保代码的可移植性和安全性。
1、MySQL数据库连接参数配置
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
'port': 3306,
}
try:
connection = mysql.connector.connect(config)
if connection.is_connected():
print("Connected to MySQL database")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
connection.close()
print("Connection closed")
2、PostgreSQL数据库连接参数配置
import psycopg2
config = {
'dbname': 'your_database',
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'port': 5432,
}
try:
connection = psycopg2.connect(config)
print("Connected to PostgreSQL database")
except psycopg2.Error as err:
print(f"Error: {err}")
finally:
if connection:
connection.close()
print("Connection closed")
三、使用连接池管理连接
使用连接池可以提高数据库连接的效率,避免频繁地创建和关闭连接。许多数据库驱动都支持连接池功能。
1、MySQL连接池
使用mysql.connector.pooling
模块创建连接池:
from mysql.connector import pooling
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
}
pool = pooling.MySQLConnectionPool(pool_name="mypool", pool_size=5, config)
try:
connection = pool.get_connection()
if connection.is_connected():
print("Connected to MySQL database using connection pool")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
connection.close()
print("Connection closed")
2、PostgreSQL连接池
使用psycopg2.pool
模块创建连接池:
from psycopg2 import pool
config = {
'dbname': 'your_database',
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'port': 5432,
}
try:
connection_pool = pool.SimpleConnectionPool(1, 10, config)
connection = connection_pool.getconn()
if connection:
print("Connected to PostgreSQL database using connection pool")
except psycopg2.Error as err:
print(f"Error: {err}")
finally:
if connection:
connection_pool.putconn(connection)
print("Connection closed")
四、处理数据库连接错误
在连接数据库时,可能会遇到各种错误,例如网络问题、数据库服务器不可用、认证失败等。处理这些错误可以提高程序的健壮性。
1、MySQL连接错误处理
import mysql.connector
from mysql.connector import Error
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
}
try:
connection = mysql.connector.connect(config)
if connection.is_connected():
print("Connected to MySQL database")
except Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
connection.close()
print("Connection closed")
2、PostgreSQL连接错误处理
import psycopg2
from psycopg2 import OperationalError
config = {
'dbname': 'your_database',
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'port': 5432,
}
try:
connection = psycopg2.connect(config)
print("Connected to PostgreSQL database")
except OperationalError as err:
print(f"Error: {err}")
finally:
if connection:
connection.close()
print("Connection closed")
五、执行数据库操作
连接数据库后,可以执行各种数据库操作,例如查询、插入、更新和删除数据。
1、MySQL数据库操作
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
# 执行查询操作
cursor.execute("SELECT * FROM your_table")
results = cursor.fetchall()
for row in results:
print(row)
# 执行插入操作
cursor.execute("INSERT INTO your_table (column1, column2) VALUES (%s, %s)", (value1, value2))
connection.commit()
# 执行更新操作
cursor.execute("UPDATE your_table SET column1 = %s WHERE column2 = %s", (new_value1, value2))
connection.commit()
# 执行删除操作
cursor.execute("DELETE FROM your_table WHERE column2 = %s", (value2,))
connection.commit()
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
print("Connection closed")
2、PostgreSQL数据库操作
import psycopg2
config = {
'dbname': 'your_database',
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'port': 5432,
}
try:
connection = psycopg2.connect(config)
cursor = connection.cursor()
# 执行查询操作
cursor.execute("SELECT * FROM your_table")
results = cursor.fetchall()
for row in results:
print(row)
# 执行插入操作
cursor.execute("INSERT INTO your_table (column1, column2) VALUES (%s, %s)", (value1, value2))
connection.commit()
# 执行更新操作
cursor.execute("UPDATE your_table SET column1 = %s WHERE column2 = %s", (new_value1, value2))
connection.commit()
# 执行删除操作
cursor.execute("DELETE FROM your_table WHERE column2 = %s", (value2,))
connection.commit()
except psycopg2.Error as err:
print(f"Error: {err}")
finally:
if connection:
cursor.close()
connection.close()
print("Connection closed")
六、使用ORM框架
使用ORM(对象关系映射)框架可以简化数据库操作,常用的ORM框架包括SQLAlchemy和Django ORM。
1、使用SQLAlchemy
SQLAlchemy是一个功能强大的ORM框架,支持多种数据库。
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
DATABASE_URL = "mysql+mysqlconnector://your_username:your_password@your_host/your_database"
engine = create_engine(DATABASE_URL)
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
定义模型
from sqlalchemy import Column, Integer, String
class YourTable(Base):
__tablename__ = 'your_table'
id = Column(Integer, primary_key=True, index=True)
column1 = Column(String, index=True)
column2 = Column(String, index=True)
创建数据库表
Base.metadata.create_all(bind=engine)
使用会话执行数据库操作
session = SessionLocal()
new_record = YourTable(column1='value1', column2='value2')
session.add(new_record)
session.commit()
session.close()
2、使用Django ORM
Django ORM是Django框架的内置ORM,适用于Django项目。
# 在Django项目的settings.py文件中配置数据库
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'your_database',
'USER': 'your_username',
'PASSWORD': 'your_password',
'HOST': 'your_host',
'PORT': '3306',
}
}
定义模型
from django.db import models
class YourTable(models.Model):
column1 = models.CharField(max_length=100)
column2 = models.CharField(max_length=100)
使用Django ORM执行数据库操作
from your_app.models import YourTable
插入数据
new_record = YourTable(column1='value1', column2='value2')
new_record.save()
查询数据
records = YourTable.objects.all()
for record in records:
print(record.column1, record.column2)
更新数据
record = YourTable.objects.get(id=1)
record.column1 = 'new_value1'
record.save()
删除数据
record = YourTable.objects.get(id=1)
record.delete()
通过以上内容,我们详细介绍了如何在Python中连接指定数据库的方法,包括使用合适的数据库驱动、配置数据库连接参数、使用连接池管理连接、处理数据库连接错误、执行数据库操作以及使用ORM框架。希望这些内容能帮助您更好地管理和操作数据库。
相关问答FAQs:
如何使用Python连接MySQL数据库?
要连接MySQL数据库,您需要安装mysql-connector-python
库。可以通过pip install mysql-connector-python
命令进行安装。连接时,需要提供数据库的主机名、用户名、密码以及数据库名称。以下是一个简单的连接示例:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
# 检查连接是否成功
if connection.is_connected():
print("成功连接到MySQL数据库")
else:
print("连接失败")
Python如何连接SQLite数据库?
连接SQLite数据库相对简单,因为SQLite是文件型数据库,不需要单独的服务器。只需导入sqlite3
模块,并指定数据库文件的路径。示例如下:
import sqlite3
connection = sqlite3.connect('example.db')
# 创建游标
cursor = connection.cursor()
# 检查连接
print("成功连接到SQLite数据库")
使用完毕后,确保关闭连接以释放资源:connection.close()
。
Python支持连接哪些类型的数据库?
Python支持多种数据库的连接,包括但不限于MySQL、PostgreSQL、SQLite、Oracle、SQL Server等。每种数据库都有对应的驱动程序,例如psycopg2
用于PostgreSQL,cx_Oracle
用于Oracle等。选择合适的库并根据官方文档进行安装和配置,可以顺利实现与这些数据库的连接。
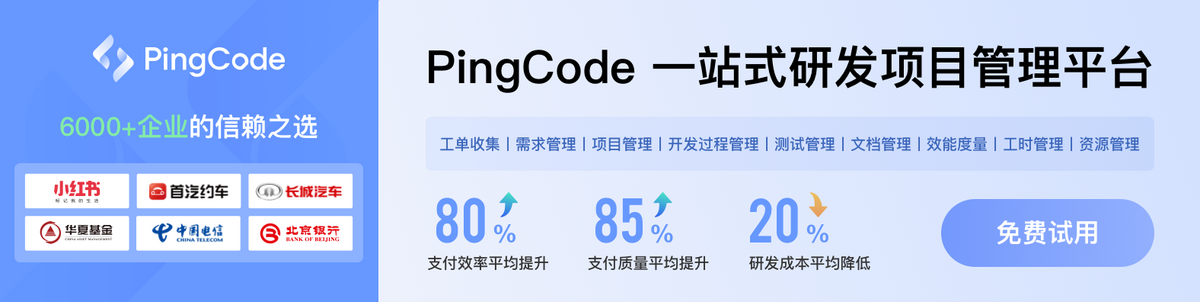