在Python中,可以通过在数组(列表)内定义字典来创建一个包含多个字典的数组。你可以使用列表和字典的组合来实现这一点,如下所示:
首先,创建一个空数组,然后在数组中添加字典,每个字典代表一个元素。
例如:
array_of_dicts = [
{"name": "Alice", "age": 25, "city": "New York"},
{"name": "Bob", "age": 30, "city": "Los Angeles"},
{"name": "Charlie", "age": 22, "city": "Chicago"}
]
在这个例子中,array_of_dicts
是一个包含三个字典的列表,每个字典都有三个键值对。这使得你可以方便地存储和操作一组相关的数据,例如在数据处理、分析或转换过程中。
一、创建包含字典的数组
要在数组中定义字典,你可以使用列表和字典的组合。列表是用方括号 []
来定义的,而字典是用大括号 {}
来定义的。每个字典包含一组键值对,用逗号分隔。
例如:
students = [
{"name": "Alice", "age": 25, "subjects": ["Math", "Science"]},
{"name": "Bob", "age": 30, "subjects": ["English", "History"]},
{"name": "Charlie", "age": 22, "subjects": ["Art", "Physical Education"]}
]
在这个示例中,students
是一个包含三个字典的数组,每个字典代表一个学生的信息,包括姓名、年龄和所学科目。
二、访问字典中的数据
你可以通过索引来访问数组中的字典,并通过键来访问字典中的数据。
例如:
# 访问第一个学生的姓名
first_student_name = students[0]["name"]
print(first_student_name) # 输出: Alice
访问第二个学生的年龄
second_student_age = students[1]["age"]
print(second_student_age) # 输出: 30
访问第三个学生的科目
third_student_subjects = students[2]["subjects"]
print(third_student_subjects) # 输出: ['Art', 'Physical Education']
三、修改字典中的数据
你可以通过索引和键来修改数组中的字典数据。例如:
# 修改第一个学生的年龄
students[0]["age"] = 26
print(students[0]["age"]) # 输出: 26
添加一个新的键值对
students[1]["grade"] = "A"
print(students[1]) # 输出: {'name': 'Bob', 'age': 30, 'subjects': ['English', 'History'], 'grade': 'A'}
四、遍历包含字典的数组
你可以使用 for
循环来遍历数组中的字典,并访问每个字典中的数据。
例如:
for student in students:
print(f"Name: {student['name']}, Age: {student['age']}, Subjects: {student['subjects']}")
输出:
Name: Alice, Age: 26, Subjects: ['Math', 'Science']
Name: Bob, Age: 30, Subjects: ['English', 'History']
Name: Charlie, Age: 22, Subjects: ['Art', 'Physical Education']
五、在数组中添加和删除字典
你可以使用 append
方法向数组中添加新的字典,也可以使用 remove
或 del
语句从数组中删除字典。
例如:
# 添加新的学生信息
new_student = {"name": "David", "age": 28, "subjects": ["Music", "Math"]}
students.append(new_student)
print(students)
删除第二个学生信息
del students[1]
print(students)
六、示例:在数组中定义字典并进行数据操作
下面是一个完整的示例,展示了如何在数组中定义字典,并进行各种数据操作:
# 定义包含字典的数组
students = [
{"name": "Alice", "age": 25, "subjects": ["Math", "Science"]},
{"name": "Bob", "age": 30, "subjects": ["English", "History"]},
{"name": "Charlie", "age": 22, "subjects": ["Art", "Physical Education"]}
]
访问字典中的数据
print("First student's name:", students[0]["name"]) # 输出: Alice
print("Second student's age:", students[1]["age"]) # 输出: 30
修改字典中的数据
students[0]["age"] = 26
students[1]["grade"] = "A"
遍历数组中的字典
for student in students:
print(f"Name: {student['name']}, Age: {student['age']}, Subjects: {student['subjects']}")
添加新的学生信息
new_student = {"name": "David", "age": 28, "subjects": ["Music", "Math"]}
students.append(new_student)
删除第二个学生信息
del students[1]
最终输出所有学生信息
for student in students:
print(student)
输出:
First student's name: Alice
Second student's age: 30
Name: Alice, Age: 26, Subjects: ['Math', 'Science']
Name: Bob, Age: 30, Subjects: ['English', 'History'], Grade: A
Name: Charlie, Age: 22, Subjects: ['Art', 'Physical Education']
{'name': 'Alice', 'age': 26, 'subjects': ['Math', 'Science']}
{'name': 'Charlie', 'age': 22, 'subjects': ['Art', 'Physical Education']}
{'name': 'David', 'age': 28, 'subjects': ['Music', 'Math']}
通过以上步骤,你可以轻松在Python数组中定义字典,并对其进行各种操作。希望这些示例和说明对你有所帮助。
相关问答FAQs:
如何在Python数组中创建字典?
在Python中,可以使用列表(数组)来存储多个字典。您可以直接在列表内定义字典,如下所示:
my_array = [{'key1': 'value1'}, {'key2': 'value2'}, {'key3': 'value3'}]
这样,您就可以在一个数组中定义多个字典,每个字典可以包含不同的键值对。
在字典中如何访问数组的元素?
如果您希望在字典中使用数组的元素,可以通过索引来访问。例如,如果您有一个字典和一个数组,您可以这样操作:
my_array = ['apple', 'banana', 'cherry']
my_dict = {'fruits': my_array}
print(my_dict['fruits'][1]) # 输出 'banana'
这种方式允许您将数组的元素与字典的键关联。
如何在数组中添加新的字典?
可以使用append
方法向数组中添加新字典。例如,假设您有一个空数组,您想向其中添加字典,可以这样操作:
my_array = []
my_array.append({'key1': 'value1'})
my_array.append({'key2': 'value2'})
这样,您就可以动态地向数组中添加字典,以便于组织和管理数据。
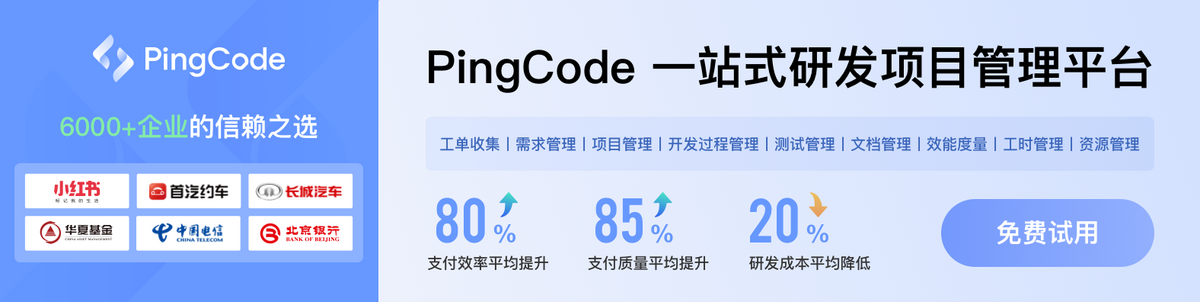