str在Python中是“string”的缩写,表示字符串类型。str是用于处理和操作文本数据的一种数据类型。在Python中,字符串可以用单引号(' ')、双引号(" ")、三引号(''' ''' 或 """ """)来表示。
str表示字符串类型、用于处理和操作文本数据、可以用单引号、双引号、三引号表示
str表示字符串类型:字符串是Python中的一种基本数据类型,用于存储文本数据。字符串可以包含字母、数字、符号和空格等字符。Python中的字符串是不可变的,这意味着一旦创建,它们就不能被改变。
一、str的基本用法
在Python中,创建字符串非常简单,只需将文本数据放在引号内即可。例如:
# 使用单引号创建字符串
str1 = 'Hello, world!'
使用双引号创建字符串
str2 = "Python is awesome!"
使用三引号创建多行字符串
str3 = '''This is a
multi-line string.'''
可以使用内置函数str()将其他数据类型转换为字符串:
num = 123
str_num = str(num)
print(str_num) # 输出:'123'
二、字符串的索引与切片
字符串是一个字符序列,每个字符都有一个索引值,可以通过索引来访问字符串中的单个字符。Python中的索引从0开始,负索引从-1开始:
string = "Python"
访问第一个字符
print(string[0]) # 输出:'P'
访问最后一个字符
print(string[-1]) # 输出:'n'
字符串切片可以获取字符串的子字符串:
string = "Hello, world!"
获取从索引2到索引5的子字符串
print(string[2:6]) # 输出:'llo,'
获取从索引7到末尾的子字符串
print(string[7:]) # 输出:'world!'
获取从开头到索引5的子字符串
print(string[:6]) # 输出:'Hello,'
三、字符串的常见操作
Python提供了许多内置方法来操作字符串。以下是一些常用的字符串操作方法:
1. 字符串拼接
可以使用加号(+)将多个字符串拼接在一起:
str1 = "Hello"
str2 = "world"
result = str1 + ", " + str2 + "!"
print(result) # 输出:'Hello, world!'
2. 字符串重复
可以使用乘号(*)将字符串重复多次:
str1 = "Python"
result = str1 * 3
print(result) # 输出:'PythonPythonPython'
3. 字符串格式化
Python提供了多种方法来格式化字符串,例如使用%
操作符、str.format()
方法和f字符串(Python 3.6+):
name = "Alice"
age = 25
使用 % 操作符
formatted_str = "My name is %s and I am %d years old." % (name, age)
print(formatted_str) # 输出:'My name is Alice and I am 25 years old.'
使用 str.format() 方法
formatted_str = "My name is {} and I am {} years old.".format(name, age)
print(formatted_str) # 输出:'My name is Alice and I am 25 years old.'
使用 f 字符串
formatted_str = f"My name is {name} and I am {age} years old."
print(formatted_str) # 输出:'My name is Alice and I am 25 years old.'
4. 大小写转换
可以使用字符串方法将字符串转换为大写或小写:
str1 = "Hello, World!"
转换为大写
upper_str = str1.upper()
print(upper_str) # 输出:'HELLO, WORLD!'
转换为小写
lower_str = str1.lower()
print(lower_str) # 输出:'hello, world!'
5. 去除空白字符
可以使用strip()
、lstrip()
和rstrip()
方法去除字符串两端的空白字符:
str1 = " Hello, World! "
去除两端的空白字符
stripped_str = str1.strip()
print(stripped_str) # 输出:'Hello, World!'
去除左端的空白字符
left_stripped_str = str1.lstrip()
print(left_stripped_str) # 输出:'Hello, World! '
去除右端的空白字符
right_stripped_str = str1.rstrip()
print(right_stripped_str) # 输出:' Hello, World!'
四、字符串的查找与替换
Python提供了一些方法来查找和替换字符串中的子字符串。
1. 查找子字符串
可以使用find()
、rfind()
、index()
和rindex()
方法查找子字符串在字符串中的位置:
str1 = "Hello, world!"
查找子字符串 'world' 的位置
position = str1.find('world')
print(position) # 输出:7
查找子字符串 'Python' 的位置(不存在)
position = str1.find('Python')
print(position) # 输出:-1
查找子字符串 'o' 最后一次出现的位置
position = str1.rfind('o')
print(position) # 输出:8
2. 替换子字符串
可以使用replace()
方法替换字符串中的子字符串:
str1 = "Hello, world!"
将 'world' 替换为 'Python'
new_str = str1.replace('world', 'Python')
print(new_str) # 输出:'Hello, Python!'
五、字符串的拆分与连接
Python提供了一些方法来拆分和连接字符串。
1. 拆分字符串
可以使用split()
方法将字符串拆分为列表:
str1 = "Hello, world! Welcome to Python."
按空格拆分字符串
words = str1.split()
print(words) # 输出:['Hello,', 'world!', 'Welcome', 'to', 'Python.']
按逗号拆分字符串
parts = str1.split(',')
print(parts) # 输出:['Hello', ' world! Welcome to Python.']
2. 连接字符串
可以使用join()
方法将列表中的字符串连接成一个字符串:
words = ['Hello', 'world', 'Python']
用空格连接字符串
sentence = ' '.join(words)
print(sentence) # 输出:'Hello world Python'
用逗号连接字符串
csv = ','.join(words)
print(csv) # 输出:'Hello,world,Python'
六、字符串的编码与解码
在处理文本数据时,有时需要将字符串编码为字节或将字节解码为字符串。Python提供了encode()
和decode()
方法来完成这些操作:
str1 = "Hello, world!"
将字符串编码为字节
encoded_str = str1.encode('utf-8')
print(encoded_str) # 输出:b'Hello, world!'
将字节解码为字符串
decoded_str = encoded_str.decode('utf-8')
print(decoded_str) # 输出:'Hello, world!'
七、字符串的常用内置函数
Python还提供了一些常用的内置函数来处理字符串,例如len()
、ord()
和chr()
:
1. 获取字符串长度
可以使用len()
函数获取字符串的长度:
str1 = "Hello, world!"
length = len(str1)
print(length) # 输出:13
2. 获取字符的Unicode码点
可以使用ord()
函数获取字符的Unicode码点:
char = 'A'
code_point = ord(char)
print(code_point) # 输出:65
3. 获取Unicode码点对应的字符
可以使用chr()
函数获取Unicode码点对应的字符:
code_point = 65
char = chr(code_point)
print(char) # 输出:'A'
八、字符串的常见问题与解决方案
在处理字符串时,可能会遇到一些常见问题,以下是一些常见问题及其解决方案:
1. 去除字符串中的所有空白字符
可以使用replace()
方法去除字符串中的所有空白字符:
str1 = " H e l l o , w o r l d ! "
no_spaces = str1.replace(' ', '')
print(no_spaces) # 输出:'Hello,world!'
2. 反转字符串
可以使用切片语法反转字符串:
str1 = "Hello, world!"
reversed_str = str1[::-1]
print(reversed_str) # 输出:'!dlrow ,olleH'
3. 检查字符串是否为回文
可以将字符串与其反转字符串进行比较,检查字符串是否为回文:
def is_palindrome(s):
return s == s[::-1]
str1 = "madam"
print(is_palindrome(str1)) # 输出:True
str2 = "hello"
print(is_palindrome(str2)) # 输出:False
九、字符串的高级操作
除了上述基本操作,Python还提供了一些高级操作来处理字符串。
1. 字符串的正则表达式
可以使用re
模块中的正则表达式来匹配和操作字符串:
import re
str1 = "The quick brown fox jumps over the lazy dog."
匹配所有单词
words = re.findall(r'\b\w+\b', str1)
print(words) # 输出:['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog']
替换所有单词 'fox' 为 'cat'
new_str = re.sub(r'\bfox\b', 'cat', str1)
print(new_str) # 输出:'The quick brown cat jumps over the lazy dog.'
2. 字符串的模板
可以使用string
模块中的Template
类来创建字符串模板:
from string import Template
template = Template("Hello, $name! Welcome to $place.")
result = template.substitute(name="Alice", place="Wonderland")
print(result) # 输出:'Hello, Alice! Welcome to Wonderland.'
十、字符串的性能优化
在处理大量字符串时,可能需要优化性能。以下是一些性能优化的建议:
1. 使用列表拼接字符串
在拼接大量字符串时,可以使用列表和join()
方法来提高性能:
# 避免使用 '+' 拼接字符串
result = ""
for i in range(1000):
result += "word"
使用列表和 join() 方法拼接字符串
words = []
for i in range(1000):
words.append("word")
result = ''.join(words)
2. 使用生成器表达式
在处理大文本数据时,可以使用生成器表达式来节省内存:
# 读取大文本文件
with open('large_file.txt', 'r') as file:
# 使用生成器表达式逐行处理文件
lines = (line.strip() for line in file)
for line in lines:
# 处理每一行
pass
通过以上内容的详细讲解,我们可以对Python中的字符串类型(str)有一个全面的了解。字符串是编程中的重要数据类型,掌握字符串的操作方法和技巧,对于编写高效和健壮的Python代码至关重要。希望这些知识能帮助你在Python编程中更好地处理和操作字符串。
相关问答FAQs:
在Python中,str的主要用途是什么?
str是Python中用于表示字符串的内置数据类型。它允许用户创建和操作文本数据,包含字母、数字和符号等字符。通过str类型,用户可以实现字符串的拼接、切割、查找、替换等多种操作,帮助在程序中有效处理文本信息。
如何将其他数据类型转换为str?
Python提供了简单的方法来将其他数据类型转换为字符串。使用内置的str()
函数,可以将整数、浮点数、列表等转换为字符串。例如,str(123)
将返回'123'。这种转换在需要将非字符串类型的数据与字符串连接时非常有用。
在Python中,如何处理字符串的常见操作?
Python的str类型提供了多种内置方法来处理字符串。例如,str.lower()
和str.upper()
可以将字符串转换为小写或大写;str.split()
方法可以将字符串按指定分隔符拆分为列表;而str.replace(old, new)
则可以用新的子串替换旧的子串。这些方法使得字符串的操作变得非常灵活和方便。
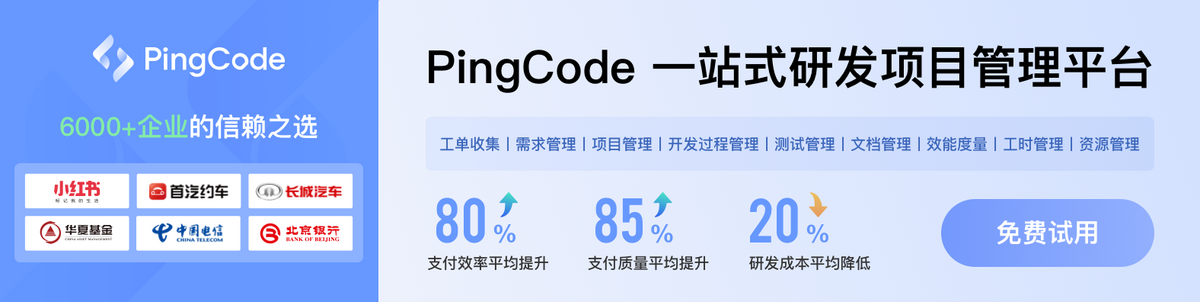