Python微信如何自动发送信息可以通过使用第三方库如itchat
、利用企业微信API、结合自动化工具如PyAutoGUI
实现。安装并使用itchat
库、配置企业微信API、使用图像识别进行自动化操作是主要方法。以下将详细介绍如何使用itchat
库实现微信消息的自动发送。
一、安装并使用itchat
库
itchat
是一个开源的微信个人号接口,支持Python语言,能够实现大部分微信功能。安装itchat
非常简单,可以通过pip命令来进行:
pip install itchat
1.1 登录微信
在使用itchat
库发送微信消息之前,首先需要登录微信。以下是一个简单的登录示例:
import itchat
登录微信,扫码后会自动登录
itchat.auto_login(hotReload=True)
hotReload=True
参数表示登录信息会缓存到本地文件中,下次运行脚本时会自动登录,无需再次扫码。
1.2 发送消息
登录成功后,可以使用itchat.send
方法发送消息。以下是一个简单的发送消息示例:
# 发送文本消息到指定用户
itchat.send('Hello, this is a message from Python!', toUserName='filehelper')
toUserName
参数指定接收消息的用户,filehelper
是微信自带的文件传输助手账号,适合用于测试。
二、配置企业微信API
企业微信提供了丰富的API接口,可以用于发送消息、管理用户、应用等。以下介绍如何配置企业微信API并发送消息。
2.1 获取企业微信API凭证
在企业微信后台,创建一个应用并获取以下信息:
- 企业ID(CorpID)
- 应用的凭证(Secret)
- 应用ID(AgentID)
2.2 发送消息
使用企业微信API发送消息需要先获取access_token
,然后调用相应的API接口。以下是一个简单的示例:
import requests
import json
企业ID和应用凭证
corp_id = 'YOUR_CORP_ID'
corp_secret = 'YOUR_CORP_SECRET'
agent_id = 'YOUR_AGENT_ID'
获取access_token
token_url = f'https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid={corp_id}&corpsecret={corp_secret}'
response = requests.get(token_url)
access_token = response.json().get('access_token')
发送消息
message_url = f'https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token={access_token}'
message_data = {
'touser': '@all',
'msgtype': 'text',
'agentid': agent_id,
'text': {
'content': 'Hello, this is a message from Python using WeChat Work API!'
}
}
response = requests.post(message_url, data=json.dumps(message_data))
print(response.json())
三、使用图像识别进行自动化操作
PyAutoGUI
是一个跨平台的GUI自动化工具,可以模拟鼠标和键盘操作。结合图像识别技术,可以实现自动发送微信消息。
3.1 安装PyAutoGUI
可以通过pip命令安装PyAutoGUI
:
pip install pyautogui
3.2 实现自动发送消息
以下是一个简单的示例,展示如何使用PyAutoGUI
自动发送微信消息:
import pyautogui
import time
打开微信应用(需要提前手动打开微信)
通过图像识别找到微信窗口位置
weixin_icon_location = pyautogui.locateOnScreen('weixin_icon.png')
if weixin_icon_location:
pyautogui.click(weixin_icon_location)
time.sleep(2) # 等待微信窗口打开
通过图像识别找到输入框位置
input_box_location = pyautogui.locateOnScreen('input_box.png')
if input_box_location:
pyautogui.click(input_box_location)
pyautogui.write('Hello, this is a message from Python using PyAutoGUI!', interval=0.1)
pyautogui.press('enter')
四、使用计划任务定时发送消息
无论是使用itchat
库还是企业微信API,都可以结合操作系统的计划任务功能实现定时发送消息。
4.1 Windows操作系统
在Windows操作系统中,可以使用任务计划程序创建定时任务。以下是步骤:
- 打开任务计划程序。
- 创建基本任务。
- 设置触发器(例如每天定时)。
- 设置操作,选择运行Python脚本。
- 完成任务创建。
4.2 Linux操作系统
在Linux操作系统中,可以使用cron
命令创建定时任务。以下是步骤:
- 打开
crontab
编辑器:
crontab -e
- 添加定时任务(例如每天定时):
0 9 * * * /usr/bin/python3 /path/to/your_script.py
五、结合数据库实现动态消息发送
在实际应用中,可能需要根据数据库中的数据动态生成发送消息内容。以下是一个简单的示例,展示如何结合SQLite数据库实现动态消息发送:
import sqlite3
import itchat
连接SQLite数据库
conn = sqlite3.connect('messages.db')
cursor = conn.cursor()
查询要发送的消息内容
cursor.execute('SELECT content FROM messages WHERE status = 0')
messages = cursor.fetchall()
登录微信
itchat.auto_login(hotReload=True)
发送消息并更新数据库状态
for message in messages:
content = message[0]
itchat.send(content, toUserName='filehelper')
cursor.execute('UPDATE messages SET status = 1 WHERE content = ?', (content,))
提交事务并关闭数据库连接
conn.commit()
conn.close()
六、结合消息队列实现异步消息发送
在高并发场景下,可以结合消息队列(如RabbitMQ、Kafka)实现异步消息发送。以下是一个简单的示例,展示如何使用RabbitMQ实现异步消息发送:
6.1 安装pika
库
可以通过pip命令安装pika
库:
pip install pika
6.2 实现消息生产者
消息生产者将待发送的消息放入消息队列:
import pika
连接RabbitMQ服务器
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
声明队列
channel.queue_declare(queue='message_queue')
发送消息
message = 'Hello, this is a message from Python using RabbitMQ!'
channel.basic_publish(exchange='', routing_key='message_queue', body=message)
关闭连接
connection.close()
6.3 实现消息消费者
消息消费者从消息队列中获取消息并发送微信消息:
import pika
import itchat
登录微信
itchat.auto_login(hotReload=True)
连接RabbitMQ服务器
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
声明队列
channel.queue_declare(queue='message_queue')
回调函数,处理从消息队列中获取的消息
def callback(ch, method, properties, body):
message = body.decode()
itchat.send(message, toUserName='filehelper')
消费消息
channel.basic_consume(queue='message_queue', on_message_callback=callback, auto_ack=True)
channel.start_consuming()
七、结合Web服务实现消息发送
可以将消息发送功能封装成Web服务,提供HTTP接口供其他系统调用。以下是一个简单的示例,展示如何使用Flask框架实现Web服务:
7.1 安装Flask框架
可以通过pip命令安装Flask框架:
pip install Flask
7.2 实现Web服务
以下是一个简单的示例,展示如何实现一个Flask Web服务,提供发送微信消息的HTTP接口:
from flask import Flask, request, jsonify
import itchat
app = Flask(__name__)
登录微信
itchat.auto_login(hotReload=True)
@app.route('/send_message', methods=['POST'])
def send_message():
data = request.get_json()
content = data.get('content')
to_user = data.get('to_user', 'filehelper')
itchat.send(content, toUserName=to_user)
return jsonify({'status': 'success'})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
以上代码实现了一个简单的Flask Web服务,提供/send_message
接口,接受POST请求,发送微信消息。可以通过以下命令启动Web服务:
python your_flask_app.py
然后,可以通过HTTP请求调用该接口发送微信消息:
curl -X POST -H "Content-Type: application/json" -d '{"content": "Hello, this is a message from Python using Flask!", "to_user": "filehelper"}' http://localhost:5000/send_message
八、结合定时任务和Web服务实现消息推送系统
可以结合定时任务和Web服务实现一个完整的消息推送系统。以下是一个简单的示例,展示如何实现一个消息推送系统:
8.1 创建消息推送任务
可以使用Flask框架提供的HTTP接口创建消息推送任务,将消息内容和发送时间存储到数据库中:
from flask import Flask, request, jsonify
import sqlite3
app = Flask(__name__)
创建SQLite数据库
conn = sqlite3.connect('tasks.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS tasks
(id INTEGER PRIMARY KEY, content TEXT, send_time TEXT, status INTEGER)''')
conn.commit()
conn.close()
@app.route('/create_task', methods=['POST'])
def create_task():
data = request.get_json()
content = data.get('content')
send_time = data.get('send_time')
conn = sqlite3.connect('tasks.db')
cursor = conn.cursor()
cursor.execute('INSERT INTO tasks (content, send_time, status) VALUES (?, ?, 0)', (content, send_time))
conn.commit()
conn.close()
return jsonify({'status': 'success'})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
8.2 定时任务检查并发送消息
可以使用cron
命令创建定时任务,定时检查数据库中的消息推送任务,并发送消息:
import sqlite3
import itchat
import time
登录微信
itchat.auto_login(hotReload=True)
while True:
conn = sqlite3.connect('tasks.db')
cursor = conn.cursor()
cursor.execute('SELECT id, content FROM tasks WHERE status = 0 AND send_time <= ?', (time.strftime('%Y-%m-%d %H:%M:%S'),))
tasks = cursor.fetchall()
for task in tasks:
task_id = task[0]
content = task[1]
itchat.send(content, toUserName='filehelper')
cursor.execute('UPDATE tasks SET status = 1 WHERE id = ?', (task_id,))
conn.commit()
conn.close()
time.sleep(60) # 每分钟检查一次
总结
Python微信自动发送消息可以通过多种方式实现,包括使用itchat
库、企业微信API、PyAutoGUI
库、结合数据库和消息队列实现动态和异步消息发送,以及将消息发送功能封装成Web服务供其他系统调用。结合定时任务和Web服务可以实现一个完整的消息推送系统。根据具体需求选择适合的实现方式,能够更好地满足业务需求。
相关问答FAQs:
如何使用Python实现自动发送微信信息的功能?
可以通过使用第三方库如itchat
来实现自动发送微信信息。首先,安装itchat
库,然后使用itchat.auto_login()
进行登录,接着使用itchat.send()
方法发送信息。需要注意的是,自动发送信息的功能可能会受到微信的限制,因此建议在使用时遵循相关的使用规范。
在使用Python发送微信信息时需要注意哪些安全问题?
在使用Python进行微信信息发送时,务必注意保护个人隐私和账号安全。切勿在公开场合泄露个人的微信登录凭证,也要避免使用不可信的库或工具。此外,频繁发送信息可能会导致账号被封禁,因此在使用自动发送功能时应控制发送频率。
如何调试Python代码以确保微信信息能够成功发送?
调试Python代码时,可以通过在代码中添加日志输出,记录每一步的执行状态和信息发送的返回结果。此外,确保网络连接正常及微信账号处于登录状态也是调试的关键步骤。如果出现错误提示,可以根据提示信息进行相应的调整或查阅相关文档以获取更多帮助。
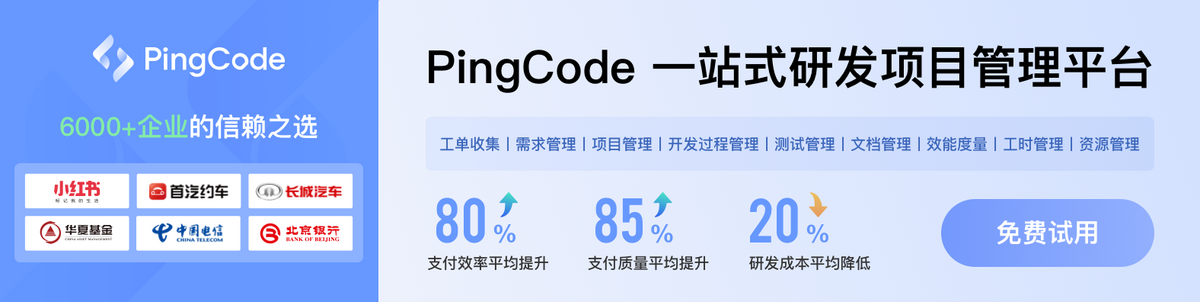