Python统计时间段数量的方法有:使用datetime模块、使用pandas模块、使用自定义函数。以下详细介绍使用datetime模块的方法。
在Python中统计时间段数量,主要依赖于datetime
模块。datetime
模块提供了强大的时间和日期处理功能,可以方便地进行时间段的统计。通过使用datetime
模块,你可以轻松地处理时间日期,计算时间差,统计特定时间段的数量。
一、使用datetime模块
1. 获取当前时间
要统计时间段数量,首先需要获取当前时间。可以使用datetime
模块中的datetime.now()
函数来获取当前时间。
from datetime import datetime
current_time = datetime.now()
print("Current Time:", current_time)
2. 计算时间差
可以使用datetime
模块的时间差功能来计算两个时间点之间的差异。timedelta
类提供了丰富的功能来计算时间差。
from datetime import datetime, timedelta
start_time = datetime(2023, 1, 1, 0, 0, 0)
end_time = datetime(2023, 1, 1, 12, 0, 0)
time_difference = end_time - start_time
print("Time Difference:", time_difference)
3. 统计特定时间段数量
要统计特定时间段的数量,可以根据需求自定义时间段,然后遍历时间数据进行统计。例如,统计每小时的时间段数量。
from datetime import datetime, timedelta
示例时间数据
time_data = [
datetime(2023, 1, 1, 0, 0, 0),
datetime(2023, 1, 1, 0, 30, 0),
datetime(2023, 1, 1, 1, 0, 0),
datetime(2023, 1, 1, 1, 30, 0),
datetime(2023, 1, 1, 2, 0, 0)
]
初始化时间段统计字典
time_periods = {}
time_period_length = timedelta(hours=1)
遍历时间数据,统计每小时的时间段数量
for time_point in time_data:
period_start = datetime(time_point.year, time_point.month, time_point.day, time_point.hour)
if period_start in time_periods:
time_periods[period_start] += 1
else:
time_periods[period_start] = 1
输出统计结果
for period_start, count in time_periods.items():
print(f"Time Period: {period_start} - Count: {count}")
二、使用pandas模块
1. 创建时间数据
使用pandas
模块处理时间数据非常方便。首先创建一个包含时间数据的DataFrame。
import pandas as pd
示例时间数据
time_data = pd.DataFrame({
'time': [
'2023-01-01 00:00:00',
'2023-01-01 00:30:00',
'2023-01-01 01:00:00',
'2023-01-01 01:30:00',
'2023-01-01 02:00:00'
]
})
将时间数据转换为datetime类型
time_data['time'] = pd.to_datetime(time_data['time'])
2. 统计特定时间段数量
使用pandas
模块可以方便地统计特定时间段的数量。例如,统计每小时的时间段数量。
# 将时间数据设置为索引
time_data.set_index('time', inplace=True)
按每小时统计时间段数量
hourly_counts = time_data.resample('H').size()
输出统计结果
print(hourly_counts)
3. 统计特定时间段数量(自定义时间段)
除了按固定时间段统计,还可以按自定义时间段进行统计。例如,统计每半小时的时间段数量。
# 按每半小时统计时间段数量
half_hourly_counts = time_data.resample('30T').size()
输出统计结果
print(half_hourly_counts)
三、使用自定义函数
1. 定义时间段统计函数
可以定义一个自定义函数来统计时间段数量。该函数可以灵活地处理各种时间段统计需求。
from datetime import datetime, timedelta
def count_time_periods(time_data, period_length):
time_periods = {}
for time_point in time_data:
period_start = datetime(time_point.year, time_point.month, time_point.day, time_point.hour, (time_point.minute // period_length) * period_length)
if period_start in time_periods:
time_periods[period_start] += 1
else:
time_periods[period_start] = 1
return time_periods
2. 使用自定义函数统计时间段数量
使用定义的自定义函数,统计每半小时的时间段数量。
# 示例时间数据
time_data = [
datetime(2023, 1, 1, 0, 0, 0),
datetime(2023, 1, 1, 0, 30, 0),
datetime(2023, 1, 1, 1, 0, 0),
datetime(2023, 1, 1, 1, 30, 0),
datetime(2023, 1, 1, 2, 0, 0)
]
统计每半小时的时间段数量
time_periods = count_time_periods(time_data, 30)
输出统计结果
for period_start, count in time_periods.items():
print(f"Time Period: {period_start} - Count: {count}")
总结
通过上述方法,详细介绍了如何使用Python中的datetime
模块、pandas
模块以及自定义函数来统计时间段数量。使用datetime
模块可以方便地进行时间和日期的处理,使用pandas
模块可以简化数据处理过程,自定义函数可以灵活处理各种统计需求。根据具体需求选择合适的方法,可以高效地统计时间段数量。
相关问答FAQs:
如何使用Python计算特定时间段内的事件数量?
在Python中,可以使用datetime
模块来处理时间数据。首先,您需要定义时间段的开始和结束时间,然后使用条件判断来统计在此时间段内发生的事件数量。例如,可以将事件存储在一个列表中,并通过循环或列表推导式筛选出符合条件的事件。
在Python中,如何处理不同格式的时间字符串?
处理时间字符串时,您可以使用datetime.strptime()
方法将字符串转换为datetime
对象。这样,您就可以方便地进行时间计算和比较。确保了解输入时间字符串的格式,并使用相应的格式符号进行转换,以避免错误。
怎样优化Python代码以提高时间统计的效率?
为了提高时间统计的效率,可以使用pandas
库来处理大量时间数据。pandas
提供了高效的数据结构和许多内置函数,可以轻松地进行时间过滤和分组操作。此外,使用numpy
进行向量化操作也能显著提升性能,尤其是在处理大数据集时。
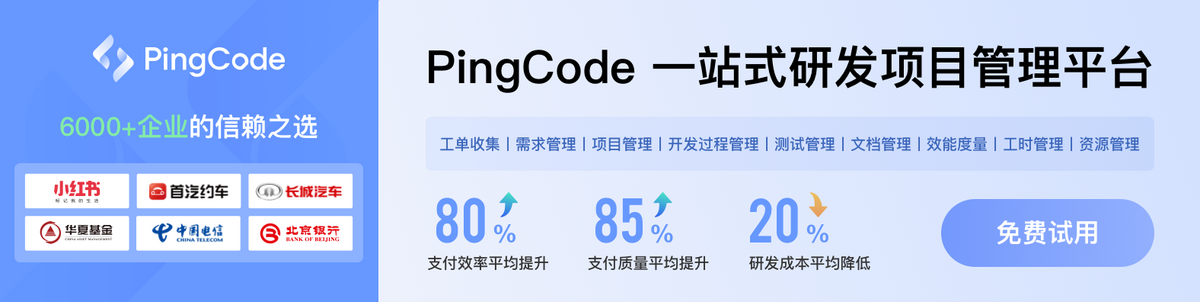