在Python中识别网页标签元素的方法有很多,但常用的有:使用BeautifulSoup、使用lxml、使用Scrapy、使用Selenium。这些工具各有优点,可以根据需求进行选择。下面我们将详细介绍如何使用这些工具来识别网页标签元素。
一、使用BeautifulSoup
BeautifulSoup是Python中用于解析HTML和XML的库,它提供了简单的API,可以方便地提取网页中的数据。使用BeautifulSoup识别网页标签元素的步骤如下:
- 安装BeautifulSoup
首先,你需要安装BeautifulSoup和HTML解析器。可以使用pip进行安装:
pip install beautifulsoup4
pip install lxml
- 解析网页内容
使用requests库获取网页内容,然后使用BeautifulSoup进行解析:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'lxml')
- 识别标签元素
使用BeautifulSoup的方法可以轻松识别和提取网页中的标签元素。例如,提取所有的链接和标题:
# 提取所有链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
提取所有标题
titles = soup.find_all(['h1', 'h2', 'h3', 'h4', 'h5', 'h6'])
for title in titles:
print(title.text)
BeautifulSoup提供了强大的选择器,可以使用标签名、类名、id等来定位元素。例如,提取指定类名的元素:
# 提取指定类名的元素
specific_class_elements = soup.find_all(class_='specific-class')
for element in specific_class_elements:
print(element.text)
二、使用lxml
lxml是另一个常用的解析HTML和XML的库,性能优于BeautifulSoup。使用lxml识别网页标签元素的步骤如下:
- 安装lxml
使用pip安装lxml:
pip install lxml
- 解析网页内容
使用requests库获取网页内容,然后使用lxml进行解析:
import requests
from lxml import html
url = 'https://example.com'
response = requests.get(url)
tree = html.fromstring(response.content)
- 识别标签元素
使用lxml的方法可以轻松识别和提取网页中的标签元素。例如,提取所有的链接和标题:
# 提取所有链接
links = tree.xpath('//a/@href')
for link in links:
print(link)
提取所有标题
titles = tree.xpath('//h1 | //h2 | //h3 | //h4 | //h5 | //h6')
for title in titles:
print(title.text)
lxml提供了强大的XPath选择器,可以使用XPath表达式来定位元素。例如,提取指定类名的元素:
# 提取指定类名的元素
specific_class_elements = tree.xpath('//*[@class="specific-class"]')
for element in specific_class_elements:
print(element.text)
三、使用Scrapy
Scrapy是一个用于抓取网站数据的强大框架,适用于复杂的网页抓取任务。使用Scrapy识别网页标签元素的步骤如下:
- 安装Scrapy
使用pip安装Scrapy:
pip install scrapy
- 创建Scrapy项目
使用Scrapy命令创建一个新的项目:
scrapy startproject myproject
- 创建Spider
在项目目录中创建一个Spider,并定义解析逻辑:
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['https://example.com']
def parse(self, response):
# 提取所有链接
links = response.css('a::attr(href)').getall()
for link in links:
print(link)
# 提取所有标题
titles = response.css('h1::text, h2::text, h3::text, h4::text, h5::text, h6::text').getall()
for title in titles:
print(title)
- 运行Spider
使用Scrapy命令运行Spider:
scrapy crawl myspider
Scrapy提供了强大的选择器,可以使用CSS选择器和XPath选择器来定位元素。例如,提取指定类名的元素:
# 提取指定类名的元素
specific_class_elements = response.css('.specific-class::text').getall()
for element in specific_class_elements:
print(element)
四、使用Selenium
Selenium是一个用于自动化网页浏览的工具,可以模拟用户的浏览器操作。使用Selenium识别网页标签元素的步骤如下:
- 安装Selenium
使用pip安装Selenium:
pip install selenium
- 下载浏览器驱动
根据你使用的浏览器下载对应的驱动程序,并将其添加到系统路径中。例如,下载ChromeDriver:
https://sites.google.com/a/chromium.org/chromedriver/downloads
- 启动浏览器
使用Selenium启动浏览器并打开网页:
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('https://example.com')
- 识别标签元素
使用Selenium的方法可以轻松识别和提取网页中的标签元素。例如,提取所有的链接和标题:
# 提取所有链接
links = driver.find_elements_by_tag_name('a')
for link in links:
print(link.get_attribute('href'))
提取所有标题
titles = driver.find_elements_by_xpath('//h1 | //h2 | //h3 | //h4 | //h5 | //h6')
for title in titles:
print(title.text)
Selenium提供了强大的选择器,可以使用标签名、类名、id等来定位元素。例如,提取指定类名的元素:
# 提取指定类名的元素
specific_class_elements = driver.find_elements_by_class_name('specific-class')
for element in specific_class_elements:
print(element.text)
总结:
在Python中识别网页标签元素的方法有很多,使用BeautifulSoup、使用lxml、使用Scrapy、使用Selenium是常用的工具。每种工具都有其优点和适用场景,可以根据需求选择合适的工具。BeautifulSoup和lxml适用于简单的网页解析任务,Scrapy适用于复杂的网页抓取任务,Selenium适用于需要模拟用户操作的任务。掌握这些工具可以帮助你高效地进行网页数据提取。
相关问答FAQs:
如何在Python中找到特定的网页标签元素?
在Python中,可以使用Beautiful Soup库来解析HTML文档并识别网页标签元素。通过使用find()
或find_all()
方法,可以轻松地定位到所需的标签。例如,使用soup.find('div')
可以找到第一个<div>
标签,而soup.find_all('a')
则可以找到所有的<a>
标签。确保在开始之前安装Beautiful Soup和requests库。
在进行网页标签元素识别时,如何处理动态生成的内容?
对于动态生成的网页内容,通常使用Selenium库来模拟浏览器操作。Selenium允许您加载完整的网页,包括JavaScript生成的部分。通过使用driver.find_element_by_xpath()
或driver.find_elements_by_css_selector()
可以有效地识别和操作这些动态生成的标签元素。
Python中还有哪些库可以用来识别网页标签元素?
除了Beautiful Soup和Selenium,Scrapy也是一个非常强大的框架,用于提取网页数据。Scrapy具有快速抓取和处理数据的能力,能够识别复杂的网页标签元素。此外,PyQuery和lxml也是流行的选择,它们提供了类似jQuery的语法,使得操作HTML文档变得更加简便。选择合适的工具可以根据具体需求和项目复杂度来决定。
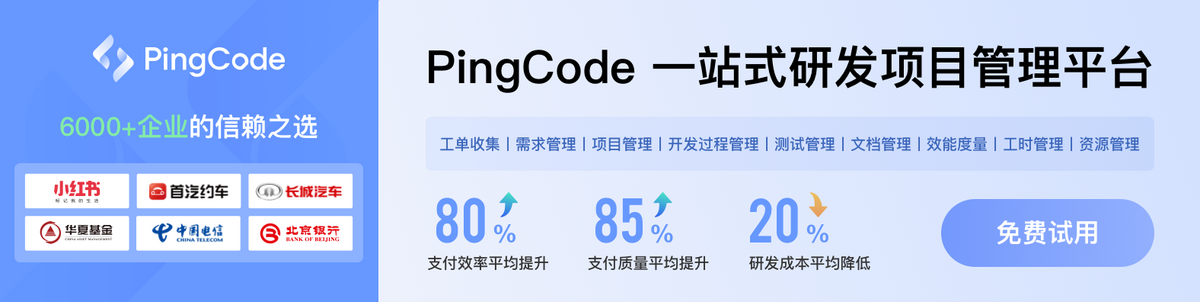