利用Python爬取多个网页信息的核心步骤包括:选择合适的爬虫工具、构建爬取逻辑、处理与解析网页内容、存储爬取的数据。
其中,选择合适的爬虫工具是至关重要的一步。Python有许多强大的库可以用于网页爬取,如Requests、BeautifulSoup、Scrapy等。Requests库用于发送HTTP请求,获取网页内容;BeautifulSoup则用于解析HTML文档;Scrapy是一个爬取网站的框架,适用于大规模的爬取任务。选择合适的工具将大大提高爬取效率和代码的简洁度。
一、选择合适的爬虫工具
Python提供了多种爬虫工具,每种工具都有其特定的应用场景和优势。
1、Requests库
Requests库是一个简单易用的HTTP库,可以用来发送HTTP请求,并获取网页内容。它的优点是简单、直观,适合用于小规模爬虫或单个网页的爬取。
import requests
url = 'http://example.com'
response = requests.get(url)
print(response.text)
2、BeautifulSoup库
BeautifulSoup库用于解析HTML文档,可以轻松地从网页中提取数据。它与Requests库配合使用效果更佳。
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title)
3、Scrapy框架
Scrapy是一个强大的爬虫框架,适用于大规模的爬取任务。它提供了很多内置功能,如处理请求、解析网页、存储数据等,使得爬取任务更加高效和灵活。
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com']
def parse(self, response):
title = response.xpath('//title/text()').get()
print(title)
二、构建爬取逻辑
在选择好合适的工具后,下一步是构建爬取逻辑。爬取逻辑的构建包括确定目标网站、分析网页结构、编写爬虫代码等。
1、确定目标网站
首先要确定要爬取的目标网站,并获取网站的URL列表。可以手动获取,也可以通过编写代码自动生成。
2、分析网页结构
在编写爬虫代码之前,需要分析目标网页的结构,找到需要爬取的数据所在的HTML标签和属性。
3、编写爬虫代码
根据分析的网页结构,编写爬虫代码,发送请求,获取网页内容,并解析所需数据。
from bs4 import BeautifulSoup
import requests
urls = ['http://example.com/page1', 'http://example.com/page2']
for url in urls:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.find('div', class_='data')
print(data.text)
三、处理与解析网页内容
在获取到网页内容后,需要对其进行处理和解析,以提取出需要的数据。
1、使用BeautifulSoup解析HTML
BeautifulSoup提供了多种方法来查找和解析HTML文档中的元素,如find、find_all、select等。
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.find_all('div', class_='data')
for item in data:
print(item.text)
2、使用正则表达式提取数据
有时需要使用正则表达式来提取特定格式的数据。Python的re模块提供了强大的正则表达式功能。
import re
import requests
url = 'http://example.com'
response = requests.get(url)
data = re.findall(r'<div class="data">(.*?)</div>', response.text)
for item in data:
print(item)
四、存储爬取的数据
爬取到的数据需要存储起来,以便后续使用。可以将数据存储到文件、数据库,或其他存储介质中。
1、存储到文件
最简单的存储方式是将数据存储到文件中,如CSV、JSON、TXT等格式。
import csv
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.find_all('div', class_='data')
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Data'])
for item in data:
writer.writerow([item.text])
2、存储到数据库
对于大规模的数据存储,可以使用数据库,如MySQL、MongoDB等。下面是将数据存储到MySQL数据库的示例。
import pymysql
from bs4 import BeautifulSoup
import requests
connection = pymysql.connect(host='localhost',
user='user',
password='password',
db='database')
cursor = connection.cursor()
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.find_all('div', class_='data')
for item in data:
cursor.execute('INSERT INTO table_name (column_name) VALUES (%s)', (item.text,))
connection.commit()
connection.close()
五、处理动态网页
有些网页内容是通过JavaScript动态加载的,这种情况下,直接使用Requests库无法获取到完整的网页内容。需要使用Selenium等工具模拟浏览器行为,加载动态内容。
1、使用Selenium模拟浏览器
Selenium是一个用于Web应用程序测试的工具,可以模拟用户操作,如点击、输入、滚动等,从而加载动态内容。
from selenium import webdriver
from bs4 import BeautifulSoup
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
soup = BeautifulSoup(driver.page_source, 'html.parser')
data = soup.find_all('div', class_='data')
for item in data:
print(item.text)
driver.quit()
六、处理反爬虫机制
有些网站为了防止被爬虫爬取,采用了反爬虫机制,如IP封禁、验证码、动态加载内容等。对于这些情况,需要采取相应的措施。
1、使用代理
使用代理IP可以避免被封禁。可以通过代理池获取多个代理IP,并在发送请求时随机选择一个使用。
import requests
proxy_pool = ['http://proxy1', 'http://proxy2']
for url in urls:
proxy = {'http': random.choice(proxy_pool)}
response = requests.get(url, proxies=proxy)
print(response.text)
2、模拟浏览器行为
通过模拟浏览器行为,可以绕过一些反爬虫机制,如使用Selenium、设置请求头、添加延迟等。
from selenium import webdriver
from bs4 import BeautifulSoup
import time
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
time.sleep(5) # 添加延迟,等待动态内容加载
soup = BeautifulSoup(driver.page_source, 'html.parser')
data = soup.find_all('div', class_='data')
for item in data:
print(item.text)
driver.quit()
七、并发爬取
为了提高爬取效率,可以使用多线程或多进程并发爬取多个网页。Python的threading和multiprocessing模块提供了多线程和多进程的支持。
1、多线程爬取
通过多线程,可以同时发送多个请求,提高爬取速度。
import threading
import requests
def fetch(url):
response = requests.get(url)
print(response.text)
threads = []
for url in urls:
thread = threading.Thread(target=fetch, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2、多进程爬取
多进程可以更好地利用多核CPU,提高爬取性能。
from multiprocessing import Pool
import requests
def fetch(url):
response = requests.get(url)
return response.text
if __name__ == '__main__':
with Pool(5) as p:
results = p.map(fetch, urls)
for result in results:
print(result)
总之,利用Python爬取多个网页信息涉及到选择合适的爬虫工具、构建爬取逻辑、处理与解析网页内容、存储爬取的数据、处理动态网页、应对反爬虫机制以及并发爬取等多个步骤。通过合理地选择工具和方法,可以高效地完成网页信息的爬取任务。
相关问答FAQs:
如何选择适合的Python库进行网页信息爬取?
在Python中,有几个常用的库可以用于网页爬取,如Beautiful Soup、Scrapy和Requests。Beautiful Soup适合处理HTML和XML文档,能够方便地提取数据。Requests库则用于发送HTTP请求,获取网页内容。Scrapy是一个全面的框架,适合大型项目,支持异步处理和爬虫管理。根据你的需求选择合适的库,可以提高开发效率。
在进行网页爬取时,如何处理反爬虫机制?
许多网站采用反爬虫技术来防止数据被大量抓取。你可以通过设置合适的请求头(如User-Agent)、使用代理IP、限制请求频率等方式来减小被检测的风险。此外,模拟人类行为,如随机等待时间和循环访问不同页面,也有助于规避反爬虫机制。
爬取数据后,如何有效存储和处理这些信息?
爬取到的数据可以存储在多种格式中,如CSV文件、JSON文件或数据库(如SQLite、MySQL)。选择存储方式时,可以考虑数据的结构和后续处理需求。对于需要频繁查询和分析的数据,使用数据库能提供更高的效率和灵活性。同时,使用Pandas等数据分析库可以方便地对数据进行清洗和分析。
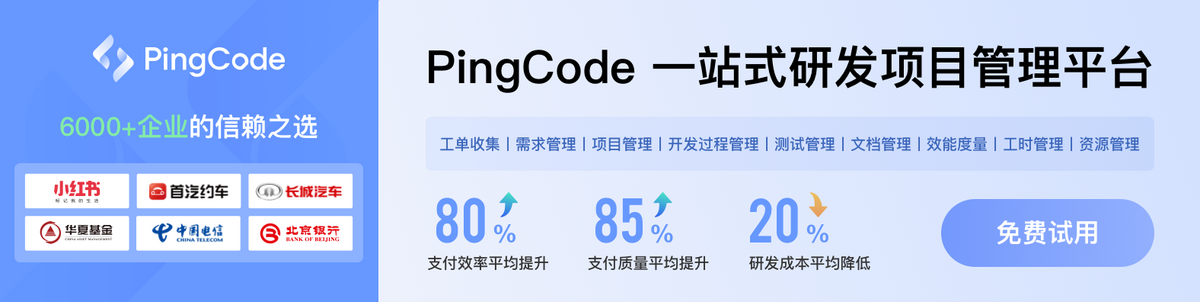