Python读取多个文本文件的方法有多种,常见的方式包括使用glob
模块、使用os
模块、使用pathlib
模块、使用pandas
库等。其中,使用glob
模块是最便捷的一种方法。接下来,我们将详细介绍这些方法中的一种:使用glob
模块。
使用glob
模块读取多个文本文件:
glob
模块可以用来查找符合特定规则的文件路径名。它可以使用通配符来匹配文件名,如*.txt
表示匹配所有的文本文件。以下是使用glob
模块读取多个文本文件的详细步骤:
import glob
定义文件路径模式
file_pattern = 'path/to/your/files/*.txt'
获取所有匹配的文件路径
file_paths = glob.glob(file_pattern)
逐个读取文件内容
for file_path in file_paths:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content) # 或者执行其他需要的操作
下面,我们将深入探讨其他几种读取多个文本文件的方法,并详细解释每个方法的具体使用步骤和注意事项。
一、使用 glob
模块
1.1 基本用法
glob
模块是 Python 中的一个标准库,可以方便地查找符合特定规则的文件路径名。它支持使用通配符来匹配文件名。
import glob
定义文件路径模式
file_pattern = 'path/to/your/files/*.txt'
获取所有匹配的文件路径
file_paths = glob.glob(file_pattern)
逐个读取文件内容
for file_path in file_paths:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content) # 或者执行其他需要的操作
1.2 读取文件并存储到列表中
有时我们可能需要将所有文件的内容读取并存储到一个列表中,以便后续进行处理。
import glob
定义文件路径模式
file_pattern = 'path/to/your/files/*.txt'
获取所有匹配的文件路径
file_paths = glob.glob(file_pattern)
存储所有文件内容的列表
contents = []
逐个读取文件内容并存储到列表中
for file_path in file_paths:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
contents.append(content)
二、使用 os
模块
2.1 基本用法
os
模块提供了与操作系统进行交互的功能,可以用来遍历文件夹并获取文件路径。
import os
定义文件夹路径
folder_path = 'path/to/your/files'
逐个读取文件内容
for file_name in os.listdir(folder_path):
if file_name.endswith('.txt'):
file_path = os.path.join(folder_path, file_name)
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content) # 或者执行其他需要的操作
2.2 递归遍历子文件夹
如果需要递归遍历文件夹及其子文件夹,可以使用 os.walk
函数。
import os
定义文件夹路径
folder_path = 'path/to/your/files'
逐个读取文件内容
for root, dirs, files in os.walk(folder_path):
for file_name in files:
if file_name.endswith('.txt'):
file_path = os.path.join(root, file_name)
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content) # 或者执行其他需要的操作
三、使用 pathlib
模块
3.1 基本用法
pathlib
是 Python 3.4 引入的新模块,用于处理文件系统路径。它提供了面向对象的方式来操作文件和目录。
from pathlib import Path
定义文件夹路径
folder_path = Path('path/to/your/files')
获取所有匹配的文件路径
file_paths = folder_path.glob('*.txt')
逐个读取文件内容
for file_path in file_paths:
with file_path.open('r', encoding='utf-8') as file:
content = file.read()
print(content) # 或者执行其他需要的操作
3.2 递归遍历子文件夹
pathlib
模块也支持递归遍历文件夹及其子文件夹。
from pathlib import Path
定义文件夹路径
folder_path = Path('path/to/your/files')
获取所有匹配的文件路径
file_paths = folder_path.rglob('*.txt')
逐个读取文件内容
for file_path in file_paths:
with file_path.open('r', encoding='utf-8') as file:
content = file.read()
print(content) # 或者执行其他需要的操作
四、使用 pandas
库
4.1 基本用法
pandas
库是一个强大的数据处理和分析工具,它可以方便地读取和处理多个文件。
import pandas as pd
import glob
定义文件路径模式
file_pattern = 'path/to/your/files/*.txt'
获取所有匹配的文件路径
file_paths = glob.glob(file_pattern)
逐个读取文件内容并存储到 DataFrame 中
data_frames = []
for file_path in file_paths:
df = pd.read_csv(file_path, sep='\t', header=None)
data_frames.append(df)
合并所有 DataFrame
all_data = pd.concat(data_frames, ignore_index=True)
print(all_data)
4.2 读取不同格式的文件
pandas
库不仅可以读取文本文件,还可以读取 CSV、Excel 等不同格式的文件。
import pandas as pd
import glob
定义文件路径模式
file_pattern = 'path/to/your/files/*.csv'
获取所有匹配的文件路径
file_paths = glob.glob(file_pattern)
逐个读取文件内容并存储到 DataFrame 中
data_frames = []
for file_path in file_paths:
df = pd.read_csv(file_path)
data_frames.append(df)
合并所有 DataFrame
all_data = pd.concat(data_frames, ignore_index=True)
print(all_data)
五、总结
在本文中,我们详细介绍了 Python读取多个文本文件 的多种方法,包括使用 glob
模块、os
模块、pathlib
模块、pandas
库等。每种方法都有其独特的优势和适用场景,可以根据具体需求选择合适的方法。
使用glob
模块、使用os
模块、使用pathlib
模块、使用pandas
库 是读取多个文本文件的常见方法。通过这些方法,我们可以方便地遍历文件夹、获取文件路径,并逐个读取文件内容。希望本文对您有所帮助,能够在实际工作中更高效地处理多个文本文件。
相关问答FAQs:
如何在Python中批量读取多个文本文件?
在Python中,可以使用os
模块和open
函数结合循环来读取多个文本文件。首先,使用os.listdir()
获取目标目录下的所有文件名,然后筛选出文本文件,最后使用open()
函数逐一读取内容。示例代码如下:
import os
directory = 'your_directory_path' # 替换为你的目录路径
for filename in os.listdir(directory):
if filename.endswith('.txt'):
with open(os.path.join(directory, filename), 'r') as file:
content = file.read()
print(content)
有没有更简便的方法读取多个文本文件?
可以使用glob
模块来简化文件读取过程。glob
允许使用通配符匹配文件名,从而直接获取所有指定类型的文件。以下示例展示了如何使用glob
读取所有文本文件:
import glob
for filename in glob.glob('your_directory_path/*.txt'):
with open(filename, 'r') as file:
content = file.read()
print(content)
读取多个文本文件时如何处理文件编码问题?
在读取文件时,可能会遇到编码不一致的问题。可以在open()
函数中指定encoding
参数,例如使用encoding='utf-8'
或encoding='gbk'
来确保正确读取文件内容。如果不确定文件编码,可以尝试使用chardet
库来检测编码类型。
import chardet
with open('your_file.txt', 'rb') as file:
rawdata = file.read()
result = chardet.detect(rawdata)
encoding = result['encoding']
with open('your_file.txt', 'r', encoding=encoding) as file:
content = file.read()
print(content)
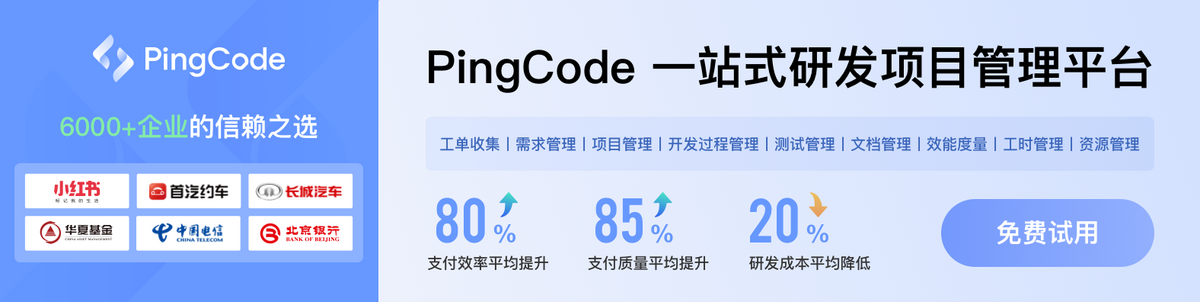